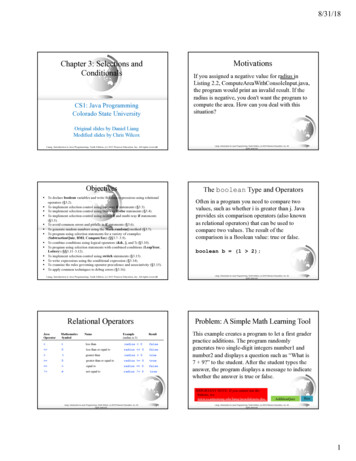
Transcription
8/31/18MotivationsChapter 3: Selections andConditionalsIf you assigned a negative value for radius inListing 2.2, ComputeAreaWithConsoleInput.java,the program would print an invalid result. If theradius is negative, you don't want the program tocompute the area. How can you deal with thissituation?CS1: Java ProgrammingColorado State UniversityOriginal slides by Daniel LiangModified slides by Chris WilcoxLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved.1Objectives2The boolean Type and Operators§ To declare boolean variables and write Boolean expressions using relationaloperators (§3.2).§ To implement selection control using one-way if statements (§3.3).§ To implement selection control using two-way if-else statements (§3.4).§ To implement selection control using nested if and multi-way if statements(§3.5).§ To avoid common errors and pitfalls in if statements (§3.6).§ To generate random numbers using the Math.random() method (§3.7).§ To program using selection statements for a variety of examples(SubtractionQuiz, BMI, ComputeTax) (§§3.7–3.9).§ To combine conditions using logical operators (&&, , and !) (§3.10).§ To program using selection statements with combined conditions (LeapYear,Lottery) (§§3.11–3.12).§ To implement selection control using switch statements (§3.13).§ To write expressions using the conditional expression (§3.14).§ To examine the rules governing operator precedence and associativity (§3.15).§ To apply common techniques to debug errors (§3.16).Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. All rights reserved.3Relational OperatorsNameLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Example(radius is 5)boolean b (1 2);Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.4Problem: A Simple Math Learning ToolJavaOperatorMathematicsSymbol less thanradius 0false less than or equal toradius 0false greater thanradius 0true greater than or equal toradius 0true equal toradius 0false! not equal toradius ! 0trueLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Often in a program you need to compare twovalues, such as whether i is greater than j. Javaprovides six comparison operators (also knownas relational operators) that can be used tocompare two values. The result of thecomparison is a Boolean value: true or false.Result5This example creates a program to let a first graderpractice additions. The program randomlygenerates two single-digit integers number1 andnumber2 and displays a question such as “What is7 9?” to the student. After the student types theanswer, the program displays a message to indicatewhether the answer is true or false.IMPORTANT NOTE: If you cannot run thebuttons, itionQuiz.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Run61
8/31/18One-way if Statementsif (boolean-expression) {statement(s);}if (radius 0) {area radius * radius * PI;System.out.println("The area" " for the circle of radius " radius " is " area);}Noteif i 0 {System .out.pr intln( " i is po sitive ");}if (i 0) {Sys tem.out .printl n( "i is positi ve ");}(a) W ron gi f (i 0) {System .out.pr intln( " i is po sitive " );}(b) C orre ctEqu iva le ntif (i 0)Syste m.out. println ( "i is positiv e ");(b)(a)Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.78The Two-way if StatementSimple if Demoif (boolean-expression) {statement(s)-for-the-true-case;Write a program that prompts the user to enter an integer. If thenumber is a multiple of 5, print HiFive. If the number is divisibleby 2, print ;}RunLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.9Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.10Multiple Alternative if Statementsif-else Exampleif (radius 0) {area radius * radius * 3.14159;System.out.println("The area for the “ “circle of radius " radius " is " area);}else {System.out.println("Negative input");}Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.}else {if (score 90.0)System.out.print("A");elseif (score 80.0)System.out.print("B");elseif (score 70.0)System.out.print("C");elseif (score ");Equivalentif (score 90.0)System.out.print("A");else if (score 80.0)System.out.print("B");else if (score 70.0)System.out.print("C");else if (score ");This is better(a)11Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.(b)122
8/31/18animationMulti-Way if-else StatementsTrace if-else statementSuppose score is 70.0The condition is falseif (score 90.0)System.out.print("A");else if (score 80.0)System.out.print("B");else if (score 70.0)System.out.print("C");else if (score ");Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.animationanimationTrace if-else statementSuppose score is 70.0The condition is falseThe condition is trueif (score 90.0)System.out.print("A");else if (score 80.0)System.out.print("B");else if (score 70.0)System.out.print("C");else if (score ");Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.animationgrade is C16Trace if-else statementSuppose score is 70.0if (score 90.0)System.out.print("A");else if (score 80.0)System.out.print("B");else if (score 70.0)System.out.print("C");else if (score ");Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.15Trace if-else statementSuppose score is 70.014Trace if-else statementSuppose score is 70.0if (score 90.0)System.out.print("A");else if (score 80.0)System.out.print("B");else if (score 70.0)System.out.print("C");else if (score ");animationLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.13Exit the if statementif (score 90.0)System.out.print("A");else if (score 80.0)System.out.print("B");else if (score 70.0)System.out.print("C");else if (score ");17Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.183
8/31/18NoteNote, cont.The else clause matches the most recent if clause in thesame block.Nothing is printed from the preceding statement. To forcethe else clause to match the first if clause, you must add apair of braces:int i 1;int j 2;int k 3;if (i j) {if (i "B");This statement prints B.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.19Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Common ErrorsTIPAdding a semicolon at the end of an if clause is a commonmistake.if (radius 0);Wrong{area radius*radius*PI;System.out.println("The area for the circle of radius " radius " is " area);}This mistake is hard to find, because it is not a compilation error ora runtime error, it is a logic error.This error often occurs when you use the next-line block style.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.21CAUTIONif (even true)System.out.println("It is even.");Equivalentif (number % 2 0)even true;elseeven false;Equivalentboolean even number % 2 0;(b)(a)Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.(b)This example creates a program to teach afirst grade child how to learn subtractions.The program randomly generates two singledigit integers number1 and number2 withnumber1 number2 and displays a questionsuch as “What is 9 – 2?” to the student. Afterthe student types the answer, the programdisplays whether the answer is correct.SubtractionQuizLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.22Problem: An Improved Math Learning Toolif (even)System.out.println("It is even.");(a)2023Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Run244
8/31/18Problem: Computing TaxesProblem: Body Mass IndexBody Mass Index (BMI) is a measure of health onweight. It can be calculated by taking your weightin kilograms and dividing by the square of yourheight in meters. The interpretation of BMI forpeople 16 years or older is as follows:BMIInterpretationBMI 18.518.5 BMI 25.025.0 BMI 30.030.0 pretBMIThe US federal personal income tax is calculatedbased on the filing status and taxable income.There are four filing statuses: single filers, marriedfiling jointly, married filing separately, and head ofhousehold. The tax rates for 2009 are shown below.RunLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.25Problem: Computing Taxes, cont.26Logical Operatorsif (status 0) {// Compute tax for single ical negation&&andlogical conjunctionif (status 3) {Compute tax for head of household orlogical disjunction{Display wrong status exclusive orlogical exclusionif (status 1) {Compute tax for married file jointlyor qualifying widow(er)if (status 2) {// Compute tax for married file separately}else//}else//}ComputeTaxLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.RunLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.27Truth Table for Operator !p!p28Truth Table for Operator &&Example (assume age 24, weight 140)p1p2p1 && p2 Example (assume age 24, weight 140)falsefalse false(age 18) && (weight 140) is false, because bothconditions are both false.true false !(age 18) is false, because (age 18) is true.false true!(weight 150)falsetruefalsetruefalse falsetruetrueis true, because (weight 150) is false.(age 18) && (weight 140) is false, because (weight 140) is false.true(age 18) && (weight 140) is true, because both(age 18) and (weight 140) are true.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.29Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.305
8/31/18Truth Table for Operator p1p2Truth Table for Operator p1 p2 Example (assume age 24, weihgt 140)false false falsefalse truetruetruep2p1 p2 Example (assume age 24, weight 140)falsefalsefalseand (weight 140) is false.falsetruetruetruefalsetrue(age 34) (weight 140) is true, because (age 34) is falsebut (weight 140) is true.(age 14) (weight 150) is false, because(age 14) is true.true true(age 34) (weight 140) is true, because (age 34) is false(age 34) (weight 140) is true, because (age 34)is false, but (weight 140) is true.true falsep1(age 14) (weight 140) is true, because (age 14) istrue and (weight 140) is false.truetrueLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.truefalseLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.31Examples32ExamplesHere is a program that checks whether a number is divisible by 2and 3, whether a number is divisible by 2 or 3, and whether anumber is divisible by 2 or 3 but not both:System.out.println("Is " number " divisible by 2 and 3? " ((number % 2 0) && (number % 3 0)));System.out.println("Is " number " divisible by 2 or 3? " ((number % 2 0) (number % 3 0)));System.out.println("Is " number TestBooleanOperatorsRunLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.CompanionWebsite" divisible by 2 or 3, but not both? " TestBooleanOperators((number % 2 0) (number % 3 0)));Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.33CompanionWebsiteThe & and OperatorsRun34The & and OperatorsIf x is 1, what is x after thisexpression?(x 1) & (x 10)Supplement III.B, “The & and Operators”If x is 1, what is x after thisexpression?(1 x) && ( 1 x )How about (1 x) (10 x )?(1 x) (10 x )?Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.35Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.366
8/31/18Problem: Determining Leap Year?This program first prompts the user to enter a year asan int value and checks if it is a leap year.A year is a leap year if it is divisible by 4 but not by100, or it is divisible by 400.(year % 4 0 && year % 100 ! 0) ( year % 400 0)LeapYearProblem: LotteryWrite a program that randomly generates a lottery of a twodigit number, prompts the user to enter a two-digit number,and determines whether the user wins according to thefollowing rule: If the user input matches the lottery in exact order, theaward is 10,000. If the user input matches the lottery, the award is 3,000. If one digit in the user input matches a digit in thelottery, the award is 1,000.RunLotteryLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.37RunLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.38switch Statement Flow Chartswitch Statementsswitch (status) {case 0: compute taxes for single filers;break;case 1: compute taxes for married file jointly;break;case 2: compute taxes for married file separately;break;case 3: compute taxes for head of household;break;default: System.out.println("Errors: invalid status");System.exit(1);}Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.39switch Statement RulesThe switch-expressionmust yield a value of char,byte, short, or int type andmust always be enclosed inparentheses.The value1, ., and valueN musthave the same data type as thevalue of the switch-expression.The resulting statements in thecase statement are executed whenthe value in the case statementmatches the value of the switchexpression. Note that value1, .,and valueN are constantexpressions, meaning that theycannot contain variables in theexpression, such as 1 x.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.switch Statement RulesThe keyword break is optional,switch (switch-expression) {switch (switch-expression) {but it should be used at the end ofeach case in order to terminate theremainder of the switchstatement. If the break statementis not present, the next casestatement will be executed.case value1: statement(s)1;break;case value2: statement(s)2;break;case value1: statement(s)1;break;case value2: statement(s)2;break; case valueN: statement(s)N; case valueN: statement(s)N;The default case, which isbreak;default: statement(s)-for-default;optional, can be used to performactions when none of thespecified cases matches theswitch-expression.}Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.4041break;default: statement(s)-for-default;}When the value in a case statement matches the valueof the switch-expression, the statements starting fromthis case are executed until either a break statement orthe end of the switch statement is reached.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.427
8/31/18animationanimationTrace switch statementSuppose day is 2:Match case 2switch (day) {case 1:case 2:case 3:case 4:case 5: System.out.println("Weekday"); break;case 0:case 6: System.out.println("Weekend");}Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.animationswitch (day) {case 1:case 2:case 3:case 4:case 5: System.out.println("Weekday"); break;case 0:case 6: System.out.println("Weekend");}animationFall through case 3Trace switch statementswitch (day) {case 1:case 2:case 3:case 4:case 5: System.out.println("Weekday"); break;case 0:case 6: System.out.println("Weekend");}Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.45animationTrace switch statementFall through case 546Trace switch statementEncounter breakswitch (day) {case 1:case 2:case 3:case 4:case 5: System.out.println("Weekday"); break;case 0:case 6: System.out.println("Weekend");}Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.44Fall through case 4switch (day) {case 1:case 2:case 3:case 4:case 5: System.out.println("Weekday"); break;case 0:case 6: System.out.println("Weekend");}animationLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.43Trace switch statementLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Trace switch statementswitch (day) {case 1:case 2:case 3:case 4:case 5: System.out.println("Weekday"); break;case 0:case 6: System.out.println("Weekend");}47Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.488
8/31/18animationTrace switch statementProblem: Chinese ZodiacWrite a program that prompts the user to enter a yearExit the statementand displays the animal for the year.switch (day) {case 1:case 2:case 3:case 4:case 5: System.out.println("Weekday"); break;case 0:case 6: System.out.println("Weekend");}ChineseZodiacLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.49Conditional Expressionsif (num % 2 0)System.out.println(num “is even”);elseSystem.out.println(num “is odd”);is equivalent toy (x 0) ? 1 : -1;(boolean-expression) ? expression1 : expression2Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.50Conditional Operatorif (x 0)y 1elsey -1;Ternary operatorBinary operatorUnary operatorRunSystem.out.println((num % 2 0)? num “is even” :num “is odd”);Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.5152Operator PrecedenceConditional Operator, cont. () var , var--boolean-expression ? exp1 : exp2 , - (Unary plus and minus), var,--var (type) Casting ! (Not) *, /, % (Multiplication, division, and remainder) , - (Binary addition and subtraction) , , , (Relational operators) , ! ; (Equality) (Exclusive OR) && (Conditional AND) Short-circuit AND (Conditional OR) Short-circuit OR , , - , * , / , % (Assignment operator)Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.53Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.549
8/31/18Operator AssociativityOperator Precedence and AssociativityThe expression in the parentheses is evaluated first.(Parentheses can be nested, in which case the expressionin the inner parentheses is executed first.) Whenevaluating an expression without parentheses, theoperators are applied according to the precedence rule andthe associativity rule.If operators with the same precedence are next to eachother, their associativity determines the order ofevaluation. All binary operators except assignmentoperators are left-associative.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.When two operators with the same precedenceare evaluated, the associativity of the operatorsdetermines the order of evaluation. All binaryoperators except assignment operators are leftassociative.a – b c – d is equivalent to ((a – b) c) – dAssignment operators are right-associative.Therefore, the expressiona b c 5 is equivalent to a (b (c 5))Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.55CompanionWebsiteExampleApplying the operator precedence and associativity rule,the expression 3 4 * 4 5 * (4 3) - 1 is evaluated asfollows:56Operand Evaluation OrderSupplement III.A, “Advanced discussions onhow an expression is evaluated in the JVM.”3 4 * 4 5 * (4 3) - 13 4 * 4 5 * 7 – 13 16 5 * 7 – 1(1) inside parentheses first(2) multiplication(3) multiplication3 16 35 – 119 35 – 119 34false(4) addition(5) subtraction(6) greater thanLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.57Debugging58DebuggerLogic errors are called bugs. The process of finding andcorrecting errors is called debugging. A common approachto debugging is to use a combination of methods to narrowdown to the part of the program where the bug is located.You can hand-trace the program (i.e., catch errors byreading the program), or you can insert print statements inorder to show the values of the variables or the executionflow of the program. This approach might work for a short,simple program. But for a large, complex program, themost effective approach for debugging is to use a debuggerutility.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.59Debugger is a program that facilitates debugging.You can use a debugger to Execute a single statement at a time. Trace into or stepping over a method. Set breakpoints. Display variables. Display call stack. Modify variables.Liang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.6010
8/31/18CompanionWebsiteDebugging in EclipseSupplement II.G, Learning Java Effectively withEclipseLiang, Introduction to Java Programming, Tenth Edition, (c) 2015 Pearson Education, Inc. Allrights reserved.6111
§ To implement selection control using one -way ifstatements (§3.3). § To implement selection control using two -way if-else statements (§3.4). § To implement selection control usi