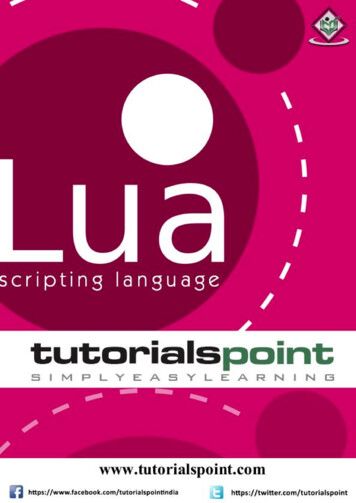
Transcription
LuaAbout the TutorialLua is an open source language built on top of C programming language. Luahas its value across multiple platforms ranging from large server systems tosmall mobile applications.This tutorial covers various topics ranging from the basics of Lua to its scope invarious applications.AudienceThis tutorial is designed for all those readers who are looking for a starting pointto learn Lua. It has topics suitable for both beginners as well as advanced users.PrerequisitesIt is a self-contained tutorial and you should be able to grasp the concepts easilyeven if you are a total beginner. However it would help if you have a basicunderstanding of working with a simple text editor and command line.Copyright & Disclaimer Copyright 2015 by Tutorials Point (I) Pvt. Ltd.All the content and graphics published in this e-book are the property ofTutorials Point (I) Pvt. Ltd. The user of this e-book is prohibited to reuse, retain,copy, distribute or republish any contents or a part of contents of this e-book inany manner without written consent of the publisher.We strive to update the contents of our website and tutorials as timely and asprecisely as possible, however, the contents may contain inaccuracies or errors.Tutorials Point (I) Pvt. Ltd. provides no guarantee regarding the accuracy,timeliness or completeness of our website or its contents including this tutorial.If you discover any errors on our website or in this tutorial, please notify us atcontact@tutorialspoint.comi
LuaTable of ContentsAbout the Tutorial . iAudience . iPrerequisites . iCopyright & Disclaimer . iTable of Contents . ii1. OVERVIEW . 1Features . 1How Lua is Implemented? . 1Learning Lua. 2Some Uses of Lua . 22. ENVIRONMENT . 3Try it Option Online . 3Local Environment Setup . 3Text Editor . 3The Lua Interpreter . 4The Lua Compiler . 4Installation on Windows . 4Installation on Linux. 4Installation on Mac OS X . 5Lua IDE . 53. BASIC SYNTAX . 7First Lua Program . 7Tokens in Lua . 8Comments . 9ii
LuaIdentifiers . 9Keywords . 9Whitespace in Lua . 104. VARIABLES . 11Variable Definition in Lua. 11Variable Declaration in Lua . 12Lvalues and Rvalues in Lua . 135. DATA TYPES . 14Type Function . 146. OPERATORS . 16Arithmetic Operators . 16Relational Operators . 17Logical Operators . 20Misc Operators . 22Operators Precedence in Lua . 227. LOOPS . 25while loop . 26for loop . 27repeat.until loop . 29nested loops . 31Loop Control Statement . 32break statement . 33The Infinite Loop . 348. DECISION MAKING . 35if statement . 36iii
Luaif.else statement . 37The if.else if.else Statement . 39nested if statements . 409. FUNCTIONS . 42Defining a Function . 42Function Arguments. 43Calling a Function . 43Assigning and Passing Functions . 44Function with Variable Argument . 4510. STRINGS . 46String Manipulation . 47Case Manipulation . 48Replacing a Substring . 48Finding and Reversing . 49Formatting Strings . 49Character and Byte Representations . 50Other Common Functions . 5111. ARRAYS . 52One-Dimensional Array . 52Multi-Dimensional Array . 5312. ITERATORS . 56Generic For Iterator . 56Stateless Iterators . 56Stateful Iterators. 58iv
Lua13. TABLES . 60Introduction . 60Representation and Usage . 60Table Manipulation . 62Table Concatenation . 62Insert and Remove . 63Sorting Tables . 6414. MODULES. 66What is a Module? . 66Specialty of Lua Modules . 66The require Function . 67Things to Remember . 68Old Way of Implementing Modules . 6815. METATABLES . 70index . 70newindex . 71Adding Operator Behavior to Tables . 72call . 74tostring . 7516. COROUTINES . 76Introduction . 76Functions Available in Coroutines . 76What Does the Above Example Do? . 78Another Coroutine Example . 78v
Lua17. FILE I/O. 81Implicit File Descriptors . 82Explicit File Descriptors . 8318. ERROR HANDLING . 86Need for Error Handling . 86Assert and Error Functions . 87pcall and xpcall . 8819. DEBUGGING . 90Debugging – Example . 93Debugging Types . 94Graphical Debugging . 9520. GARBAGE COLLECTION . 96Garbage Collector Pause . 96Garbage Collector Step Multiplier . 96Garbage Collector Functions . 9621. OBJECT ORIENTED . 99Introduction to OOP. 99Features of OOP . 99OOP in Lua . 99A Real World Example. 100Creating a Simple Class . 100Creating an Object . 101Accessing Properties . 101Accessing Member Function . 101Complete Example . 101vi
LuaInheritance in Lua . 102Overriding Base Functions . 103Inheritance Complete Example . 10322. WEB PROGRAMMING . 106Applications and Frameworks . 106Orbit . 106Creating Forms . 109WSAPI . 110Xavante. 111Lua Web Components . 113Ending Note . 11323. DATABASE ACCESS . 115MySQL db Setup . 115Importing MySQL . 115Setting up Connection . 115Execute Function. 116Create Table Example . 116Insert Statement Example . 117Update Statement Example . 117Delete Statement Example . 117Select Statement Example . 117A Complete Example . 118Performing Transactions . 119Start Transaction. 119Rollback Transaction . 119Commit Transaction . 119vii
LuaImporting SQLite . 120Setting Up Connection . 120Execute Function. 120Create Table Example . 120Insert Statement Example . 121Select Statement Example . 121A Complete Example . 12124. GAME PROGRAMING . 124Corona SDK . 124Gideros Mobile . 125ShiVa3D . 125Moai SDK . 126LOVE . 126CryEngine . 126An Ending Note . 12725. STANDARD LIBRARIES. 128Basic Library . 128Modules Library . 131String manipulation . 132Table manipulation . 132File Input and output . 132Debug facilities . 13226. MATH LIBRARY . 133Trigonometric Functions . 135Other Common math Functions . 136viii
Lua27. OPERATING SYSTEM FACILITIES. 138Common OS functions . 139ix
1. OVERVIEWLuaLua is an extensible, lightweight programming language written in C. It startedas an in-house project in 1993 by Roberto Ierusalimschy, Luiz Henrique deFigueiredo, and Waldemar Celes.It was designed from the beginning to be a software that can be integrated withthe code written in C and other conventional languages. This integration bringsmany benefits. It does not try to do what C can already do but aims at offeringwhat C is not good at: a good distance from the hardware, dynamic structures,no redundancies, ease of testing and debugging. For this, Lua has a safeenvironment, automatic memory management, and good facilities for handlingstrings and other kinds of data with dynamic size.FeaturesLua provides a set of unique features that makes it distinct from otherlanguages. These include: Extensible Simple Efficient Portable Free and openExample Codeprint("Hello World!")How Lua is Implemented?Lua consists of two parts - the Lua interpreter part and the functioning softwaresystem. The functioning software system is an actual computer application thatcan interpret programs written in the Lua programming language. The Luainterpreter is written in ANSI C, hence it is highly portable and can run on a vastspectrum of devices from high-end network servers to small devices.Both Lua's language and its interpreter are mature, small, and fast. It hasevolved from other programming languages and top software standards. Beingsmall in size makes it possible for it to run on small devices with low memory.1
LuaLearning LuaThe most important point while learning Lua is to focus on the concepts withoutgetting lost in its technical details.The purpose of learning a programming language is to become a betterprogrammer; that is, to become more effective in designing and implementingnew systems and at maintaining old ones.Some Uses of Lua Game Programming Scripting in Standalone Applications Scripting in Web Extensions and add-ons for databases like MySQL Proxy and MySQLWorkBench Security systems like Intrusion Detection System.2
2. ENVIRONMENTLuaTry it Option OnlineWe have already set up the Lua Programming environment online, so that youcan build and execute all the available examples online at the same time whenyou are doing your theory work. This gives you confidence in what you arereading and to check the result with different options. Feel free to modify anyexample
Lua consists of two parts - the Lua interpreter part and the functioning software system. The functioning software system is an actual computer application that can interpret programs written in the Lua programming language. The Lua interpreter is written in ANSI C, hence it is highly portable and can run on a vast