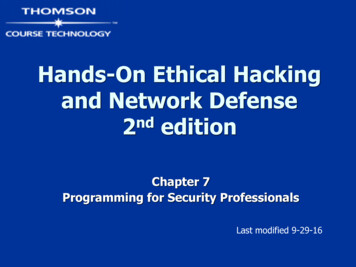
Transcription
Hands-On Ethical Hackingand Network Defensend2 editionChapter 7Programming for Security ProfessionalsLast modified 9-29-16
Objectives Explain basic programming conceptsWrite a simple C programExplain how Web pages are created withHTMLDescribe and create basic Perl programsExplain basic object-oriented programmingconcepts2
Introduction to ComputerProgramming Computer programmers must understandthe rules of programming languages One minor mistake and the program willnot run Programmers deal with syntax errorsOr worse, it will produce unpredictable resultsBeing a good programmer takes time andpatience3
Computer ProgrammingFundamentals Fundamental concepts Branching, Looping, and Testing (BLT)DocumentationFunction Mini program within a main program thatcarries out a task4
Branching, Looping, and Testing(BLT) Branching Looping Takes you from one area of the program toanother areaAct of performing a task over and overTesting Verifies some condition and returns true orfalse5
A C Program Filename ends in .cIt's hard to read at firstA single missing semicolon can ruin aprogram6
Comments Comments make code easier to read7
Branching and Testingmain()Diagram of branchesSee links Ch 7b, 7cprintf()scanf()8
Looping9
Branching, Looping, and Testing(BLT) Algorithm Bug Defines steps for performing a taskKeep it as simple as possibleAn error that causes unpredictable resultsPseudocode English-like language used to create thestructure of a program10
Pseudocode For Shopping PurchaseIngredients Function Call GetCar FunctionCall DriveToStore FunctionPurchase Bacon, Bread, Tomatoes,Lettuce, and MayonnaiseEnd PurchaseIngredients Function11
Documentation Documenting your work is essential Add comments to your programsComments should explain what you are doingMany programmers find it time consumingand tediousHelps others understand your work12
Bugs Industry standard 20 to 30 bugs for every 1000 lines of code(link Ch 7f) Windows 2000 contains almost 50 million lines Textbook claims a much smaller number without a sourceAnd fewer than 60,000 bugs (about 1 per 1000 lines)See link Ch 7e for comments in the leaked Win 2000source codeLinux has 0.17 bugs per 1000 lines of code (Link Ch 7f)13
Learning the C Language Developed by Dennis Ritchie at BellLaboratories in 1972Powerful and concise languageUNIX was first written in assemblylanguage and later rewritten in CC is an enhancement of the C languageC is powerful but dangerous Bugs can crash computers, and it's easy toleave security holes in the code14
Assembly Language The binary language hard-wired into theprocessor is machine languageAssembly Language uses a combination ofhexadecimal numbers and expressions Very powerful but hard to use (Link Ch 7g)15
Compiling C in Ubuntu Linux Compiler Converts a text-based program (source code)into executable or binary codeTo prepare Ubuntu Linux for Cprogramming, use this command:sudo apt-get install build-essential Then you compile a file named "program.c"with this command:gcc program.c –o program16
Anatomy of a C Program The first computer program a C studentlearns "Hello, World!"17
Comments Use /* and */ to comment large portions oftextUse // for one-line comments18
Include #include statement Loads libraries that hold the commands andfunctions used in your program19
Functions A Function Name is always followed byparentheses ( )Curly Braces { } shows where a functionbegins and endsmain() function Every C program requires a main() functionmain() is where processing starts20
Functions Functions can call other functions Parameters or arguments are optional\n represents a line feed21
Declaring Variables A variable represents a numeric or stringvalueYou must declare a variable before using it22
Variable Types in C23
Mathematical Operators The i in the example below adds one tothe variable i24
Mathematical Operators25
Logical Operators The i 11 in the example below comparesthe variable i to 1126
Logical Operators27
Demonstration: Buffer Overflow28
Buffer Overflow Defenses30
Detecting stack smashing with a canary valueCANARY
Understanding HTML Basics HTML is a language used to create WebpagesHTML files are text filesSecurity professionals often need toexamine Web pages Be able to recognize when something lookssuspicious40
Creating a Web Page Using HTML Create HTML Web page in NotepadView HTML Web page in a Web browserHTML does not use branching, looping, ortestingHTML is a static formatting language Rather than a programming language and symbols denote HTML tags Each tag has a matching closing tag HTML and /HTML 41
42
43
44
Understanding Practical Extractionand Report Language (Perl) PERL Powerful scripting languageUsed to write scripts and programs for securityprofessionals45
Background on Perl Developed by Larry Wall in 1987Can run on almost any platform *NIX-base OSs already have Perl installedPerl syntax is similar to CHackers use Perl to write malwareSecurity professionals use Perl to performrepetitive tasks and conduct securitymonitoring46
47
Understanding the Basics of Perl perl –h command Gives you a list of parameters used with perl48
49
Understanding the BLT of Perl Some syntax rules Keyword “sub” is used in front of functionnamesVariables begin with the characterComment lines begin with the # characterThe & character is used when calling afunction50
Branching in Perl&speak; Calls the subroutinesub speak Defines the subroutine51
For Loop in Perl For loop52
Testing Conditions in Perl53
Understanding Object-OrientedProgramming Concepts New programming paradigmThere are several languages that supportobject-oriented programming C C#JavaPerl 6.0Object Cobol54
Components of Object-OrientedProgramming Classes Structures that hold pieces of data andfunctionsThe :: symbol Used to separate the name of a class from amember functionExample: Employee::GetEmp()55
Example of a Class in C class Employee{public:char firstname[25];char lastname[25];char PlaceOfBirth[30];[code continues]};void GetEmp(){// Perform tasks to get employee info[program code goes here]}56
Ruby Example Metasploit is written in RubySee link Ch 7u57
LOLCODELinks Ch 7x, Ch 7y56
53
BrainfuckLink Ch 7z56
"Hello, World!" in Brainfuck56
13 Bugs Industry standard 20 to 30 bugs for every 1000 lines of code (link Ch 7f) Textbook claims a much smaller number without a source Windows 2000 contains almost 50 million lines And fewer than 60,000 bugs (about 1 per 1000 lines) See link Ch 7e for comments in the leaked Win 2000 source code Linux has 0.17 bugs per 1000 lines of code