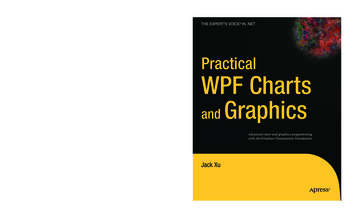
Transcription
CYANMAGENTAYELLOWBLACKBooks for professionals by professionals Author ofPractical C# Charts andGraphicsPractical SilverlightProgrammingCompanioneBook AvailablePractical WPF Charts andGraphicsPracticalDear Reader,Practical Numerical Methodswith C#If you’ve picked up this book, then you must know how important charts andgraphics are in every Windows application. Whether you’re a professional programmer, a scientist, an engineer, a teacher, or a student, you will always end updealing with chart and graphics applications to some degree.I’ve been working with charts and graphics for many years. As a theoreticalphysicist, my work involves extensive numerical computations and graphicalrepresentations of calculated data. I still remember how hard it was in the earlydays to present computational results graphically. I often spent hours creatingpublication-quality charts by hand, using a ruler, graph paper, and rub-off lettering. Around that time, I started paying attention to various development toolsI could use to create integrated applications. The C# and Windows PresentationFoundation (WPF) development environment made it possible to create suchintegrated applications because the .NET platform is one of the best developmenttools available that provides the computational capabilities to both generate dataas a simulation engine and to display it in a variety of graphical representations.I wrote this book with the intention of providing you with a comprehensiveexplanation of WPF chart and graphics capability, and I paid special attentionto creating various charts that can be used directly for real-world applications.In this book, I wanted to show you how to create a variety of graphics and chartsthat range from simple two-dimensional (2D) line plots to complicated threedimensional (3D) surface graphs.In short, this is more than just a book—it’s a powerful 2D and 3D charts andgraphics package. You will find that many of the examples in the book can be immediately used in your real-world applications, and that many others will inspire youto create advanced graphical and sophisticated chart capabilities of your own.Jack Xu, PhDCompanion eBookTHE APRESS ROADMAPPractical WPFCharts and GraphicsWPF Recipesin C# 2008ProWPF in C# 2008ProSilverlight 3 in C#FoundationExpression Blend 2SOURCE CODE ONLINEwww.apress.comUS 54.99PracticalWPF Chartsand GraphicsAdvanced chart and graphics programmingwith the Windows Presentation FoundationJack XuXu)3". WPF Charts and GraphicsPractical WPF GraphicsProgrammingSee last page for detailson 10 eBook versionThe EXPERT’s VOIce in .netShelve in:.NETUser level:Intermediate–Advanced this print for content only—size & color not accuratetrim 7.5" x 9.25" spine 1.34375"712 page count
Practical WPF Chartsand GraphicsAdvanced Chart and Graphics Programmingwith the Windows Presentation FoundationJack Xui
Practical WPF Charts and GraphicsCopyright 2009 by Jack XuAll rights reserved. No part of this work may be reproduced or transmitted in any form or byany means, electronic or mechanical, including photocopying, recording, or by anyinformation storage or retrieval system, without the prior written permission of the copyrightowner and the publisher.ISBN-13 (pbk): 978-1-4302-2481-5ISBN-13 (electronic): 978-1-4302-2482-2Printed and bound in the United States of America 9 8 7 6 5 4 3 2 1Trademarked names may appear in this book. Rather than use a trademark symbol with everyoccurrence of a trademarked name, we use the names only in an editorial fashion and to thebenefit of the trademark owner, with no intention of infringement of the trademark.Lead Editor: Ewan BuckinghamTechnical Reviewer: Todd MeisterEditorial Board: Clay Andres, Steve Anglin, Mark Beckner, Ewan Buckingham, TonyCampbell, Gary Cornell, Jonathan Gennick, Michelle Lowman, Matthew Moodie,Jeffrey Pepper, Frank Pohlmann, Ben Renow-Clarke, Dominic Shakeshaft, Matt Wade,Tom WelshCopy Editor: Elliot SimonCompositor: MacPS, LLCIndexer: BIM Indexing and Proofreading ServicesArtist: April MilneDistributed to the book trade worldwide by Springer-Verlag New York, Inc., 233 Spring Street,6th Floor, New York, NY 10013. Phone 1-800-SPRINGER, fax 201-348-4505, e-mail ordersny@springer-sbm.com, or visit http://www.springeronline.com.For information on translations, please e-mail info@apress.com, or visithttp://www.apress.com.Apress and friends of ED books may be purchased in bulk for academic, corporate, orpromotional use. eBook versions and licenses are also available for most titles. For moreinformation, reference our Special Bulk Sales–eBook Licensing web page athttp://www.apress.com/info/bulksales.The information in this book is distributed on an “as is” basis, without warranty. Althoughevery precaution has been taken in the preparation of this work, neither the author(s) norApress shall have any liability to any person or entity with respect to any loss or damagecaused or alleged to be caused directly or indirectly by the information contained in this work.The source code for this book is available to readers at http://www.apress.com. You will needto answer questions pertaining to this book in order to successfully download the code.ii
For my family, Ruth, Anna, Betty, and Tyleriii
Contents at a Glance Contents at a Glance . iv Contents. v About the Author . xv Technical Reviewer . xvi Acknowledgments . xvii Introduction . xix Overview of WPF Programming .1 2D Transformations .11 WPF Graphics Basics in 2D .59 Colors and Brushes.123 2D Line charts .163 Specialized 2D Charts .217 Stock Charts .275 Interactive 2D Charts .305 2D Chart Controls .333 Data Interpolations .393 Curve Fitting .419 3D Transformations .445 WPF Graphics Basics in 3D .499 3D Charts with the WPF 3D Engine .531 3D Charts Without the WPF 3D Engine .571 Specialized 3D Charts .633 Index .673iv
Contents Contents at a Glance . iv Contents. v About the Author . xv Technical Reviewer . xvi Acknowledgments . xvii Introduction . xix Chapter 1: Overview of WPF Programming .1New features in WPF . 1XAML Basics . 2Why Is XAML Needed? . 2Creating XAML Files. 2Code-Behind Files . 3Your First WPF Program. 4Properties in XAML . 5Event Handlers in Code-Behind Files . 5Code-Only Example. 7XAML-Only Example . 9 Chapter 2: 2D Transformations.11Basics of Matrices and Transformations . 11Vectors and Points . 11Scaling . 12Reflection . 13Rotation . 13Translation . 14Homogeneous Coordinates . 15Translation in Homogeneous Coordinates . 15v
Scaling in Homogeneous Coordinates . 16Rotation in Homogeneous Coordinates . 17Combining Transforms . 18vi
CONTENTSVectors and Matrices in WPF . 19 Vector Structure .19 Matrix Structure .21 Matrix Operations.22 Matrix Transforms.25 Creating Perpendicular Lines.31 Object Transformations in WPF. 36 MatrixTransform Class.38 ScaleTransform Class .42 TranslateTransform Class .45 RotateTransform Class.48 SkewTransform Class .52 Composite Transforms.55 Chapter 3: WPF Graphics Basics in 2D.59 2D Coordinate Systems in WPF. 59 Default Coordinates.59 Custom Coordinates.62 Custom Coordinates for 2D Charts.67 2D Viewport.72 Zooming and Panning .73 Resizable Canvas .76 Basic 2D Graphics Shapes in WPF . 80 Lines.80 Rectangles and Ellipses .82 Polylines.86 Polygons.88 Paths and Geometries. 91 Line, Rectangle, and Ellipse Geometries.92 GeometryGroup Class.93 CombinedGeometry Class .95 PathGeometry Class.98 Lines and Polylines .99 Arcs.99 Bezier Curves .100 Geometry and Mini-Language.102 Hit Testing.105 Custom Shapes . 108 Star Class.108 ArrowLine Class .112 vi
CONTENTSTesting Custom Shapes .117 Chapter 4: Colors and Brushes.123 Colors. 123 System Colors .124 Color Picker.127 Brushes. 134 SolidColorBrush .134 LinearGradientBrush .137 RadialGradientBrush .141 DrawingBrush .144 Custom Colormap and Shading. 147 Custom Colormap Brushes.147 Testing Colormap Brushes .153 Color Shading.155 Testing Color Shading .159 Chapter 5: 2D Line charts.163 Simple Line Charts. 163 Creating Simple Line Charts .163 How It Works.166 Line Charts with Data Collection. 166 Chart Style .166 Data Series.168 Data Collection .170 Creating Line Charts.171 Gridlines and Labels. 174 Chart Style with Gridlines.174 Creating a Chart with Gridlines .179 Legends . 183 Legend Class.183 Creating a Chart with a Legend .188 Symbols . 192 Defining Symbols .192 Symbols Class.193 Creating a Chart with Symbols .200 Line Charts with Two Y Axes. 203 Why We Need Two Y Axes.203 Chart Style with Two Y Axes .205 DataSeries and DataCollection with Two Y Axes .211 vii
CONTENTSCreating a Chart with Two Y Axes.213 Chapter 6: Specialized 2D Charts.217 Bar Charts . 217 DataSeries for Bar Charts .217 DataCollection for Bar Charts.218 Creating Simple Bar Charts.224 Creating Group Bar Charts .229 Creating Overlay Bar Charts.233 Creating Stacked Bar Charts.234 Stair-Step Charts . 236 DataSeries for Stair-Step Charts.236 DataCollection for Stair-Step Charts .237 Creating Stair-Step Charts .238 Stem Charts . 241 DataCollection for Stem Charts.241 Creating Stem Charts.242 Error Bar Charts . 244 DataSeries for Error Bars .244 DataCollection for Error Bars .246 Creating Error Bar Charts.248 Area Charts . 250 DataSeries for Area Charts.250 DataCollection for Area Charts.251 Creating Area Charts.253 Polar Charts . 255 Chart Style for Polar Charts.255 DataCollection for Polar Charts .260 Creating Polar Charts .261 Pie Charts. 265 Pie Chart Style.265 Legend for Pie Charts.268 Creating Pie Charts .269 Chapter 7: Stock Charts .275 Static Stock Charts . 275 Text File Reader .275 DataSeries and DataCollection for Stock Charts.278 Hi-Lo Stock Charts .281 Hi-Lo Open-Close Stock Charts.285 Candlestick Stock Charts .286 viii
CONTENTSMoving Averages . 287 Simple Moving Averages.287 Implementation .288 Creating SMA Curves .290 Weighted Moving Averages .293 Implementation .293 Creating WMA Curves .295 Exponential Moving Averages .296 Implementation .297 Creating EMA Curves .299 Using Yahoo Stock Charts in WPF. 300 Connecting to Yahoo Stock Charts.300 Creating Yahoo Stock Charts in WPF .302 Chapter 8: Interactive 2D Charts.305 Automatic Tick Placeme
Xu WPF Charts and Graphics Companion eBook Available Practical trim 7.5" x 9.25" spine 1.34375" 712 page count The eXPeRT's VOIce In .neT Practical WPF Charts and Graphics