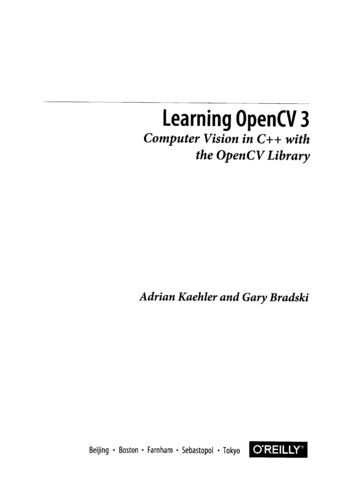
Transcription
Learning OpenCV3ComputerVision in C withtheOpenCVLibraryAdrian Kaehler and Gary BradskiBeijing Boston Farnham Sebastopol TokyoO'REILLY
Table of ContentsPrefacexv1. OverviewWhat Is1OpenCV?OpenCV?1Who Uses2What Is Computer Vision?3The6OriginofOpenCVOpenCV Block DiagramSpeeding Up OpenCV withWho Owns OpenCV?Downloadingand8IPP910Installing OpenCV10InstallationGettingMore10the LatestOpenCVOpenCVvia Git13Documentation13Supplied Documentation14Online Documentation and the ilding ses192. Introduction toOpenCV21Include Files21ResourcesFirstProgram—Display aSecondProgram—VideoMoving Around22Picture232527iii
AASimple TransformationNot-So-Simple TransformationInput g to Know OpenCV Data Types41The Basics41OpenCVDataTypes41Overview of the Basic42Basic Types:44TypesGetting Down to DetailsHelper ObjectsUtility FunctionsThe Template Structures4.606768Exercises69Images and Large Array Typesand Variable71Storage71The cv::Mat Class: N-Dimensional Dense Arrays72CreatingArrayAccessing Array Elements IndividuallyThe N-ary Array Iterator: NAryMatlteratorAccessing Array Elements by BlockMatrix Expressions: Algebra and cv::MatSaturation CastingMore Things an Array Can DoThe cv::SparseMat Class: Sparse ArraysAccessing Sparse Array ElementsFunctions Unique to Sparse ArraysTemplate Structures for Large Array rray 235AVI Filean31 You Can Do eighted()103cv::bitwise and()106Table of Contents104
cv::bitwise not()107cv::bitwise or()107cv::bitwise xor()108cv::calcCovarMatrix()108cv:: cv::flip()cv::gemm()cv::getConvertElem() and 122123124125130136136136Table of Contents v
()142cv::randu()cv::randn()143cv::randShuf spose()Summary153Exercises154Drawing and AnnotatingDrawing ThingsLine Art and Filled Polygons157Fonts and Text153154157158165Summary167Exercises1677. Functors inOpenCVThat "Do Stuff'vi141169ObjectsPrincipal Component Analysis (cv::PCA)Singular Value Decomposition (cv::SVD)169Random Number Generator (cv::RNG)176169173Summary179Exercises180 Table of Contents
8.Image, Video, and Data FilesHighGUI: Portable Graphics ToolkitWorking with Image FilesLoading and Saving Images183183185185A Note About Codecs188and DecompressionCompressionWorking with VideoReading Video with the cv::VideoCapture ObjectWriting Video with the cv:: Video Writer Object188Data leStoragea cises2049. Cross-Platform and Native WindowsWorking207with Windows207HighGUI Native Graphical UserInterface208with the Qt BackendWorkingIntegrating OpenCVSummary220with Full GUI Toolkits232247Exercises24710. Filters and Convolution249Overview249Before We Begin249Filters, Kernels, and Convolution249Border Extrapolation and251ThresholdOtsu'sBoundary veSmoothingSimple Blur and the Box Filter259261262Median Filter265Gaussian Filter266Bilateral Filter267Derivatives and Gradients269The Sobel Derivative269Scharr Filter272The273LaplacianImage Morphology275Table of Contents vii
Dilation and Erosion276The GeneralMorphology FunctionandOpeningClosingMorphological GradientTop Hat and Black Hat281Making289Your Own KernelConvolution withanArbitraryLinear FilterApplyingaGeneral Filter withApplyingaGeneralcv::filter2D()Separable Filter with cv::sepFilter2DKernel Builders287290291292292Summary294294Image Transforms299Overview299Stretch, Shrink, Warp, andRotateUniform Resize299300Pyramids302Nonuniform MappingsAffine Transformation306ImagePerspective TransformationGeneral RemappingsPolar MappingsLogPolarArbitrary MappingsImage 25Histogram Equalizationcv::equalizeHist(): Contrast equalizationSummary328Exercises332Image Analysis335Overview335Discrete Fourier Transformcv::dft(): TheDiscrete Fourier Transformcv::idft(): The331336336339339Convolution Using Discrete Fourier Transforms340The Discrete Cosine Transformcv::idct(): The Inverse Discrete Fourier Transform331cv::mulSpectrums(): Spectrum Multiplicationcv::dct():viii285Exercises11. General12.281Table of ContentsInverse Discrete Cosine Transform342343
Integral Imagescv::integral() for Standard Summation Integralcv::integral() for Squared Summation Integralcv::integral() for Tilted Summation IntegralThe Canny Edge msHough349Line Transform349Hough Circle Transform354Distance Transformation358cv::distanceTransform() for Unlabeled Distance Transformcv::distanceTransform() for Labeled Distance Transform359360Segmentation360Flood 66Segmentation368Summary370Exercises371Histograms and TemplatesHistogram Representationcv::calcHist(): Creating a373in376OpenCVHistogram from Data377with380ManipulationsHistogramsHistogram NormalizationBasic380380Histogram ThresholdFinding the MostPopulated Bin380Comparing Two HistogramsHistogram Usage ExamplesSome More Sophisticated Histograms Methods382386388Earth Mover's Distance389Back ProjectionTemplate MatchingSquare Difference Matching Method (cv::TM SQDIFF)Normalized Square Difference Matching Method(cv::TM SQDIFF NORMED)Correlation Matching Methods (cv::TM CCORR)Normalized Cross-Correlation Matching Method(cv::TM CCORR NORMED)Correlation Coefficient Matching Methods (cv::TM CCOEFF)Normalized Correlation Coefficient Matching Method(cv::TM CCOEFF NORMED)394397399400400400400401Table of Contents ix
Summary404Exercises40414. ContoursContour407Finding407Contour HierarchiesDrawing408ContoursA Contour413Example414Another Contour Example416Fast Connected417Component AnalysisMore to Do with Contours420Polygon ApproximationsGeometry and Summary CharacteristicsGeometrical TestsMatching15.428Contours andImages429429More About Moments431Matching and Hu MomentsUsing Shape Context to Compare ShapesSummary435Exercises442Background Subtraction445Overview of Background Subtraction445Weaknesses of Background Subtraction446ModelingFrame436441447A Slice of Pixels447Differencing451MethodAveraging BackgroundAccumulating Means, Variances, and Covariances452A More Advanced467BackgroundSubtraction ning with Moving ForegroundObjectsBackground Differencing: Finding Foreground Objects474Using the Codebook477Background ModelA Few MoreThoughts on Codebook ModelsConnected Components for Foreground CleanupAQuick TestTwoTable of CV Background Subtraction EncapsulationThe cv::BackgroundSubtractor Base Class 421MomentsScenex420483485485
KaewTraKuPongand Bowden Method486Zivkovic Method16.488Summary490Exercises491Keypoints and Descriptors493Keypoints and the Basics of Tracking493CornerFinding494Introduction toOpticalFlowLucas-Kanade Method for500and DescriptorsGeneralizedKeypointsOptical Flow, Tracking, and RecognitionHow OpenCV Handles Keypoints and Descriptors,Core498Sparse Optical FlowKeypoint511513the General CaseDetection Methods526Keypoint FilteringMatching MethodsDisplaying ckingin587ConceptsTrackingDense Optical FlowThe Farneback588Polynomial Expansion Algorithm589The Dual TV-L1AlgorithmThe Simple Flow AlgorithmMean-Shift and Camshift plates605Estimators613The Kalman FilterA Brief Note on615the Extended Kalman Filter633Summary634Exercises63418. Camera Models and Calibration637Camera ModelThe Basics of ns Distortions648CalibrationTable of Contents xi
Rotation Matrix and Translation Vector650Calibration Boards652Homography660Camera Calibration665Undistortion677Undistortion entationscv::convertMaps()Computing Undistortion Maps with cv::initUndistortRectifyMap()Undistorting an Image with cv::remap()Undistortion with19.cv::undistort()Undistortion withSparse680682683683cv::undistortPoints()Putting Calibration All Together684Summary687Exercises688Projection and Three-Dimensional Vision691Projections692Affine and694Perspective TransformationsBird's-Eye-View Transform Example695Three-Dimensional Pose EstimationPose Estimation pipolar Geometry703704708The Essential and Fundamental Matrices710Computing Epipolar720LinesStereo Calibration721Stereo Rectification726StereoCorrespondenceStereo Calibration,Rectification, and737CorrespondenceDepth Maps from Three-Dimensional ReprojectionStructure from MotionFittingLines in Two and Three es766Learning in OpenCVWhat Is Machine Learning?Training and Test SetsSupervised and Unsupervised Learning76920. The Basics of MachineGenerative and Discriminative Modelsxii679 Table of Contents770770771773
OpenCV ML AlgorithmsUsing Machine Learning in774Vision776Variable Importance778Diagnosing Machine Learning ProblemsLegacy Routines in the ML Library785K-Means786Mahalanobis Distance793Summary797Exercises79721. StatModel: The Standard Model forCommon Routines in the MLLearning in OpenCV799Library799Training and the cv::ml::TrainData Structure802Prediction809Machine LearningNaive/NormalBinary22.779Algorithms UsingBayes Classifiercv::StatModel810810Decision Trees816Boosting830Random Trees837Expectation MaximizationK-Nearest NeighborsMultilayer PerceptronSupport Vector MachineSummary842Exercises871Object DetectionTree-Based Object846849859870875DetectionTechniques875Cascade ClassifiersSupervised Learning876andBoosting Theory879Learning New Objects888DetectionUsing Support Vector MachinesLatent SVM for Object DetectionThe Bag of Words Algorithm and Semantic 723. Future of OpenCV909Past and Present909OpenCV3.x910How Well Did Our Predictions Go Last Time?911Future Functions912Table of Contents xiii
Current GSoC WorkCommunityContributionsOpenCV.orgSome AISpeculationAfterword913915916917920A. Planar Subdivisions923B.opencv contrib939C. Calibration Patterns943Bibliography949Index967xiv Table of Contents
Learning OpenCV 3 : computer vision in C with the OpenCV library Subject: Sebastopol, CA, O'Reilly Media, 2016 Keywords: Signatur des Originals (Print): T 17 B 2052. Digitalisiert von der TIB, Hannover, 2017. Created Date: