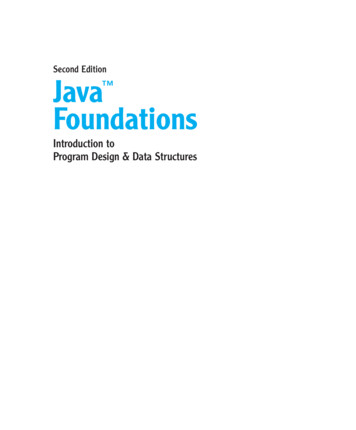
Transcription
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage iSecond EditionJavaFoundations Introduction toProgram Design & Data Structures
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage ii
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage iiiSecond EditionJavaFoundations Introduction toProgram Design & Data StructuresJohn LewisVirginia TechPeter DePasqualeThe College of New JerseyJoseph ChaseRadford UniversityAddison-WesleyBoston Columbus Indianapolis New York San FranciscoUpper Saddle River Amsterdam Cape Town Dubai London MadridMilan Munich Paris Montreal Toronto Delhi Mexico CitySao Paulo Sydney Hong Kong Seoul Singapore Taipei Tokyo
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage ivEditor-in-Chief:Editorial Assistant:Director of Marketing:Marketing Coordinator:Managing Editor:Production Project Manager:Senior Manufacturing Buyer:Media Manufacturing Buyer:Art Director:Cover Designer:Cover Art:Media Project Manager:Full-Service Project Management:Composition:Michael HirschStephanie SellingerMargaret WhaplesKathryn FerrantiJeffrey HolcombHeather McNallyCarol MelvilleGinny MichaudLinda KnowlesSusan ParadiseMarc Romanelli/Getty ImagesKatelyn BollerRose Kernan, Nesbitt Graphics, Inc.Nesbitt Graphics, Inc.Credits and acknowledgments borrowed from other sources and reproduced, with permission, in this textbook appear onappropriate page within text.Microsoft and Windows are registered trademarks of the Microsoft Corporation in the U.S.A. and other countries. Screenshots and icons reprinted with permission from the Microsoft Corporation. This book is not sponsored or endorsed by oraffiliated with the Microsoft Corporation.The programs and applications presented in this book have been included for their instructional value. They have been testedwith care, but are not guaranteed for any particular purpose. The publisher does not offer any warranties or representations,nor does it accept any liabilities with respect to the programs or applications.Copyright 2011, 2008. Pearson Education, Inc., publishing as Addison-Wesley, 501 Boylston Street, Suite 900, Boston,Massachusetts 02116. All rights reserved. Manufactured in the United States of America. This publication is protected byCopyright, and permission should be obtained from the publisher prior to any prohibited reproduction, storage in a retrievalsystem, or transmission in any form or by any means, electronic, mechanical, photocopying, recording, or likewise. To obtainpermission(s) to use material from this work, please submit a written request to Pearson Education, Inc., PermissionsDepartment, 501 Boylston Street, Suite 900, Boston, Massachusetts 02116.Many of the designations by manufacturers and sellers to distinguish their products are claimed as trademarks. Where thosedesignations appear in this book, and the publisher was aware of a trademark claim, the designations have been printed ininitial caps or all caps.Library of Congress Cataloging-in-Publication DataLewis, John, 1963Java foundations : introduction to program design & data structures / JohnLewis, Peter J. DePasquale, Joseph Chase. -- 2nd ed.p. cm.ISBN 0-13-212881-01. Java (Computer program language) I. DePasquale, Peter J. (Peter Joseph)II. Chase, Joseph, 1964- III. Title.QA76.73.J38L48845 2010005.13'3--dc22200905113410 9 8 7 6 5 4 3 2 1–EB–14 13 12 11 10ISBN-10: 0-13-212881-0www.pearsonhighered.comISBN-13: 978-0-13-212881-0
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage vTo my wife, Sharon, for everything.– JohnTo my wife, Lisa, and our twins: Lily and Adam.– PeteTo my loving wife, Melissa, for her support and encouragement.– Joe
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage vi
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage viiPrefaceWelcome to Java Foundations. This book is designed to serve as the primaryresource for a two- or three-term introductory course sequence, ranging from themost basic programming concepts to the design and implementation of complexdata structures. This unified approach makes the important introductorysequence more cohesive and accessible for students.We’ve borrowed the best elements from the industry-leading text Java SoftwareSolutions for the introductory material, reworked to complement the design andvision of the overall text. For example, instead of having graphics sections spreadthroughout many chapters, the coverage of graphical user interfaces is accomplished in a well-organized chapter of its own.In the later chapters, the exploration of collections and data structures is modeled somewhat after the coverage in Java Software Structures, but has been thoroughly retooled to flow cleanly from the introductory material. The result is acomprehensive, cohesive, and seamless exploration of programming concepts.New in the Second EditionWe appreciate the feedback we’ve received about this book and are pleased thatit served so well as an introductory text. The following modifications have beenmade to improve the presentation of particular topics and the overall flow: A stack is now used as the initial example of a collection so that the concept of a collection is more clearly established. The discussion of Generics has been expanded and clarified. The coverage of the Quick Sort and Merge Sort algorithms has beenexpanded. The coverage of Analysis of Algorithms has been separated into its ownchapter and expanded. Material on Testing and Debugging has been incorporated into moreappropriate locations of the text. The coverage of Search Trees and Heaps have been divided into separatechapters.vii
7112 Lewis FM ppi-xxviii.qxdviii2/5/1011:27 AMPage viiiPR EFA CE Two new chapters covering Hashing and Databases have been added. End-of-chapter exercises and projects have been updated to reflect changesin the book.Regarding ObjectsPhrases like objects-first, objects-early, and objects-late continue to be bandiedabout by computing educators, despite the fact that the nuances of the pedagogyof the introductory sequence cannot be summed up so easily. We’ll take thisopportunity to discuss our approach.First, this book is purely object-oriented, presented in a gradual, natural manner. Concepts that overlap with procedural programming, such as methods andtheir invocation, are discussed in terms of an object-oriented approach. Thus, noexample is ever made up of a single class with multiple methods. In fact, in ourexamples the class that contains the main method never contains another.We use objects right from the start, and discuss everything in object-orientedterms at all times. An overview of object-oriented concepts is given in Chapter 1,then reinforced and fleshed out throughout the book. Classes from the Java standard class library are introduced immediately, and objects from these classes areinstantiated and used for the various services they provide. In the first four chapters, students explore and write programs made up of a single class with a singlemain method—but these programs actively use predefined classes and objectsfrom the standard library in addition to exploring fundamental programmingconcepts such as expressions and conditionals.We never introduce third-party classes simply as fodder to create examples.That approach can confuse students by blurring the distinction between classesthat are part of the standard library (and thus always available) and “extras”thrown in by textbook authors as a convenience. Every non-library class usedin an example is fully explored in this book. There’s no “magic” behind thescenes.The debate continues: should coverage of control structures come before thedetails of writing classes, or vice versa? The truth is there are advantages eitherway, and a knowledgeable instructor can capitalize on either approach. If classcomposition comes first, it exposes the underlying essence of objects earlier anddemystifies their use. However, without the ability to use basic control structures,the examples at that point are often uninteresting and unrealistic. This bookexplores control structures before writing classes. Chapter 4 uses small, singlemethod examples to examine the details of conditionals and loops, providing astrong foundation for the multiclass examples in Chapter 5.
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage ixP REFAC EChapter BreakdownChapter 1 (Introduction) introduces the Java programming language and thebasics of program development. It contains an introduction to object-orienteddevelopment, including an overview of concepts and terminology. This chaptercontains broad introductory material that can be covered while students becomefamiliar with their development environment.Chapter 2 (Data and Expressions) explores some of the basic types of data usedin a Java program and the use of expressions to perform calculations. It discussesthe conversion of data from one type to another, and how to read input interactively from the user with the help of the Scanner class.Chapter 3 (Using Classes and Objects) explores the use of predefined classesand the objects that can be created from them. Classes and objects are used tomanipulate character strings, produce random numbers, perform complex calculations, and format output. Packages, enumerated types, and wrapper classes arealso discussed.Chapter 4 (Conditionals and Loops) covers the use of boolean expressions tomake decisions. All related statements for conditionals and loops are discussed,including the enhanced version of the for loop. The Scanner class is revisitedfor iterative input parsing and reading text files.Chapter 5 (Writing Classes) explores the basic issues related to writing classesand methods. Topics include instance data, visibility, scope, method parameters,and return types. Constructors, method design, static data, and method overloading are covered as well. Testing and debugging are now covered in this chapter aswell.Chapter 6 (Graphical User Interfaces) is a thorough exploration of Java GUIprocessing, focusing on components, events, and listeners. Many types of components and events are discussed using numerous GUI examples. Additionally, layout mangers, containment hierarchies, borders, tooltips, and mnemonics areintroduced.Chapter 7 (Arrays) contains extensive coverage of arrays and array processing.Topics include bounds checking, initializer lists, command-line arguments, variablelength parameter lists, and multidimensional arrays.Chapter 8 (Inheritance) covers class derivations and associated concepts suchas class hierarchies, overriding, and visibility. Strong emphasis is put on the properuse of inheritance and its role in software design.Chapter 9 (Polymorphism) explores the concept of binding and how it relatesto polymorphism. Then we examine how polymorphic references can be accomplished using either inheritance or interfaces. Design issues related to polymorphism are examined as well.ix
7112 Lewis FM ppi-xxviii.qxdx2/5/1011:27 AMPage xPR EFA CEChapter 10 (Exceptions) covers exception handling and the effects ofuncaught exceptions. The try-catch statement is examined, as well as a discussion of exception propagation. The chapter also explores the use of exceptions when dealing with input and output, and examines an example that writesa text file.Chapter 11 (Recursion) covers the concept, implementation, and proper use ofrecursion. Several examples are used to elaborate on the discussion, including amaze traversal and the classic Towers of Hanoi problem.Chapter 12 (Analysis of Algorithms) discusses the techniques for analyzing thecomplexity of algorithms, including recursive algorithms. Big Oh notation isintroduced.Chapter 13 (Searching and Sorting) explores the linear and binary searchingalgorithms, as well as five sorting algorithms. The sorts include both quadraticand O(N log N) algorithms. The efficiency of these algorithms is examined.Chapter 14 (Stacks) introduces the concept of a collection and establishes theimportance of separating the interface from the implementation. Stacks are usedas the initial example of a collection, and both dynamic and fixed implementations of a stack are explored. Generic types are introduced in this chapter, detailing their use in supporting the collection classes.Chapter 15 (Queues) introduces FIFO queues and discusses options for theirimplementation. As with stacks, a queue is explored first conceptually, then astools to help us solve problems, and finally by examining their underlying datastructures. Both array-based and dynamic link implementations are discussed.Chapter 16 (Trees) introduces the terms and concepts behind trees. Variousimplementation strategies are discussed, and a recursive, linked approach is examined in detail. An example of a binary decision tree is explored as well.Chapter 17 (Binary Search Trees) covers the concept of search trees and alinked implementation for a classic binary search tree. Tree rotation algorithmsare also discussed.Chapter 18 (Heaps and Priority Queues) discusses the concept of a heap andits relationship to trees. A full linked implementation of a heap is explored.Priority queues are used as an example of a collection in its own right, and thenatural relationship between heaps and priority queues are explored.Chapter 19 (Graphs) discusses both directed and undirected graphs.Additionally, weighted graphs are explored, and the differences between breadthfirst and depth-first graph traversals are covered. Minimal spanning trees areintroduced, and implementation strategies are discussed.Chapter 20 (Hashing) covers the concept of creating a hash table to facilitatestorage and retrieval of objects. Various classes that relate to hashing from theJava API are explored.
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xiP REFAC EChapter 21 (Databases) explores the concept of databases and their management, and discusses the basics of SQL queries. It then explores the techniques forestablishing a connection between a Java program and a database, and the APIused to interact with it.Student CDThe CD included with each textbook contains: Source code for all of the programs in the text. The Java Software Development Kit (SDK). Various Java development environments, including NetBeans , Eclipse ,DrJava, jGRASP , and TextPad .Instructor ResourcesThe following supplements are available to qualified instructors only. Visit thePearson Education Instructor Resource Center (www.pearsonhighered.com/irc)or send email to computing@aw.com for information on how to access theseresources. Presentation Slides—lecture-ready presentations for each chapter inMicrosoft PowerPoint format. Solutions—full solutions to the exercises and programming projects. Test Bank with powerful test generator software—includes a wealth offree-response, multiple-choice, and true/false questions.AcknowledgmentsEducators and students from around the world have provided feedback on previous work that has allowed us to mold this book into a fresh, valuable resource.Your comments and questions are always welcome.The talent and commitment of the team at Addison-Wesley continues to amazeus. We greatly appreciate the insight of Michael Hirsch, our editor, and the hardwork of his assistant, Stephanie Sellinger. Rose Kernan at Nesbitt Graphics was agreat help throughout the production process. We thank all of these people forensuring that this book meets the highest quality standards.We’d like to acknowledge the collective input from hundreds of professors andstudents around the world in the development of the material upon which thisxi
7112 Lewis FM ppi-xxviii.qxdxii2/5/1011:27 AMPage xiiPREFA CEbook is based. There are too many of you to individually name, but your influence on Java Software Solutions and Java Software Structures is evident in JavaFoundations.Special thanks go to Ruth Dannenfelser, Cory Samaha, and Zach Zappala atthe College of New Jersey for their help with solutions to the database projects.And our continued thanks go to Jason Snyder at Virginia Tech for his assistancetesting code and many other contributions.Groups like the ACM Special Interest Group on Computer Science Education(SIGCSE), the Consortium for Computing Sciences in Colleges (CCSC), and theComputer Science Teachers Association (CSTA) are phenomenal resources. Theirconferences and online activities provide opportunities for educators from all levels and all types of schools to share ideas and materials. If you are a computingeducator and are not involved with these groups, you’re missing out.Finally, we thank our families for their support and patience during the busyprocess of writing.
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xiiiContentsPrefaceviiChapter 1 Introduction11.1The Java Programming LanguageA Java ProgramCommentsIdentifiers and Reserved WordsWhite Space1.2Program DevelopmentProgramming Language LevelsEditors, Compilers, and InterpretersDevelopment EnvironmentsSyntax and SemanticsErrors1111131516171.3Problem Solving181.4Software Development Activities191.5Object-Oriented ProgrammingObject-Oriented Software Principles2121Chapter 2 Data and Expressions23569312.1Character StringsThe print and println MethodsString ConcatenationEscape Sequences323234372.2Variables and AssignmentVariablesThe Assignment StatementConstants383840422.3Primitive Data TypesIntegers and Floating Points4343xiii
7112 Lewis FM ppi-xxviii.qxdxiv2/5/1011:27 AMPage xivC ON T E NTSCharactersBooleans45472.4ExpressionsArithmetic OperatorsOperator PrecedenceIncrement and Decrement OperatorsAssignment Operators47484851532.5Data ConversionConversion Techniques54562.6Reading Input DataThe Scanner Class5757Chapter 3 Using Classes and Objects713.1Creating ObjectsAliases72743.2The String Class763.3PackagesThe import Declaration79803.4The Random Class823.5The Math Class853.6Formatting OutputThe NumberFormat ClassThe DecimalFormat ClassThe printf Method888890923.7Enumerated Types923.8Wrapper ClassesAutoboxing9597Chapter 4 Conditionals and Loops4.1Boolean ExpressionsEquality and Relational OperatorsLogical Operators105106107108
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xvC O NT ENT S4.2The if StatementThe if-else StatementUsing Block StatementsThe Conditional OperatorNested if Statements1101131151181194.3Comparing DataComparing FloatsComparing CharactersComparing Objects1211211211224.4The switch Statement1234.5The while StatementInfinite LoopsNested LoopsOther Loop Controls1271321331374.6IteratorsReading Text Files1371384.7The do Statement1414.8The for StatementIterators and for LoopsComparing Loops144149149Chapter 5 Writing Classes1615.1Classes and Objects RevisitedIdentifying Classes and ObjectsAssigning Responsibilities1621631655.2Anatomy of a ClassInstance DataUML Class Diagrams1651701715.3EncapsulationVisibility ModifiersAccessors and Mutators1731741755.4Anatomy of a MethodThe return StatementParameters180186188xv
7112 Lewis FM ppi-xxviii.qxdxvi2/5/1011:27 AMPage xviC ON T E NTSLocal DataConstructors Revisited1891905.5Static Class MembersStatic VariablesStatic Methods1901911915.6Class RelationshipsDependencyDependencies Among Objectsof the Same ClassAggregationThe this Reference1921955.7Method DesignMethod DecompositionMethod Parameters Revisited2032042095.8Method Overloading2105.9TestingReviewsDefect TestingUnit TestingIntegration TestingSystem TestingTest-Driven e Debugging with print StatementsDebugging Concepts220221221Chapter 6 Graphical User Interfaces1951972022336.1GUI ElementsFrames and PanelsButtons and Action EventsDetermining Event Sources2342352392406.2More ComponentsText FieldsCheck BoxesRadio Buttons243244247250
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xviiC O NT ENT SSlidersCombo BoxesTimers2552602656.3Layout ManagersFlow LayoutBorder LayoutGrid LayoutBox LayoutContainment Hierarchies2692712742782802846.4Mouse and Key EventsMouse EventsKey EventsExtending Adapter Classes2842842922976.5Dialog BoxesFile ChoosersColor Choosers2983013046.6Some Important DetailsBordersTool Tips and Mnemonics3053053096.7GUI Design315Chapter 7 Arrays3257.1Array Elements3267.2Declaring and Using ArraysBounds CheckingAlternate Array SyntaxInitializer ListsArrays as Parameters3273293353353367.3Arrays of Objects3377.4Command-Line Arguments3467.5Variable-Length Parameter Lists3487.6Two-Dimensional ArraysMultidimensional Arrays351355xvii
7112 Lewis FM ppi-xxviii.qxdxviii2/5/1011:27 AMPage xviiiC ON T E NTSChapter 8 Inheritance3638.1Creating SubclassesThe protected ModifierThe super ReferenceMultiple Inheritance3643683703718.2Overriding MethodsShadowing Variables3743758.3Class HierarchiesThe Object ClassAbstract Classes3763783808.4Visibility3828.5Designing for InheritanceRestricting Inheritance385385Chapter 9 Polymorphism3939.1Late Binding3949.2Polymorphism via Inheritance3949.3InterfacesInterface HierarchiesThe Comparable InterfaceThe Iterator Interface4074124134139.4Polymorphism via InterfacesEvent Processing414416Chapter 10 Exceptions42310.1Exception Handling42410.2Uncaught Exceptions42510.3The try-catch StatementThe finally Clause42542910.4Exception Propagation430
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xixC O NT ENT S10.5The Exception Class HierarchyChecked and Unchecked Exceptions43343610.6I/O Exceptions437Chapter 11 Recursion44711.1Recursive ThinkingInfinite RecursionRecursion in Math44844944911.2Recursive ProgrammingRecursion vs. IterationDirect vs. Indirect Recursion45045345311.3Using RecursionTraversing a MazeThe Towers of Hanoi454454459Chapter 12 Analysis of Algorithms46912.1Algorithm Efficiency47012.2Growth Functions and Big-Oh Notation47112.3Comparing Growth FunctionsMethod CallsAnalyzing Recursive Algorithms473475477Chapter 13 Searching and Sorting48113.1SearchingLinear SearchBinary Search48248548713.2SortingSelection SortInsertion SortBubble SortQuick SortMerge Sort489490497498500501xix
7112 Lewis FM ppi-xxviii.qxdxx2/5/1011:27 AMPage xxC ON T E NTS13.3Analyzing Searching and SortingAlgorithmsComparing Search AlgorithmsComparing Sort AlgorithmsChapter 14 Stacks50350450451314.1Introduction to CollectionsAbstract Data TypesThe Java Collections API51451451614.2A Stack Collection51714.3Inheritance, Polymorphism,and GenericsGenerics51952014.4A Stack ADT52114.5Using Stacks: Evaluating ting a Stack: with ArraysManaging Capacity53253214.8The ArrayStack ClassThe push OperationThe pop OperationThe peek OperationOther Operations53353753753853814.9References as Links53814.10 Managing Linked ListsAccessing ElementsInserting NodesDeleting NodesSentinel Nodes54154154254354414.11 Elements Without LinksDoubly Linked Lists544545
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xxiC O NT ENT S14.12 Implementing a Stack: With LinksThe LinkedStack ClassThe push OperationThe pop OperationOther Operations54654655055255514.13 Implementing Stacks:The Java.Util.Stack ClassUnique OperationsInheritance and Implementation55355355414.14 PackagesOrganizing PackagesUsing CLASSPATH554555555Chapter 15 Queues56715.1A Queue ADT56815.2Using Queues: Code Keys57015.3Using Queues: Ticket Counter Simulation57415.4Implementing Queues: With LinksThe enqueue OperationThe dequeue OperationOther Operations57758258358315.5Implementing Queues: With ArraysThe enqueue OperationThe dequeue OperationOther Operations584587590590Chapter 16 Trees59516.1Tree TerminologyTree Classifications59659716.2Tree TraversalsPreorder TraversalInorder TraversalPostorder TraversalLevel-Order Traversal598600601601602xxi
7112 Lewis FM ppi-xxviii.qxdxxii2/5/1011:27 AMPage xxiiC ON T E NTS16.3Strategies for Implementing TreesComputed Links in an ArrayStored Links in an ArrayLinked Nodes60260360360416.4A Binary Tree Implementation60516.5Decision Trees606Chapter 17 Binary Search Trees17.1625Binary Search TreesAdding an Element to a Binary Search TreeRemoving an Element from a BinarySearch Tree62662717.2Binary Search Tree Implementation63017.3Balanced Binary Search TreesRight RotationLeft RotationRight-Left RotationLeft-Right Rotation640641642642643Chapter 18 Heaps and Priority Queues64918.1629HeapsAdding an Element to a HeapRemoving the Largest Elementfrom a Heap65065018.2Heap Implementation65218.3Heap Sort66018.4Priority Queues660Chapter 19 Graphs65166919.1Undirected Graphs67019.2Directed Graphs671
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xxiiiC O NT ENT S19.3Weighted Graphs67319.4Common Graph AlgorithmsTraversalsTesting for ConnectivityMinimum Spanning TreesDetermining the Shortest Path67467467868068219.5Strategies for Implementing GraphsAdjacency ListsAdjacency Matrices683684684Chapter 20 Hashing68920.1Hashing69020.2Hashing FunctionsThe Division MethodThe Folding MethodThe Mid-square MethodThe Radix Transformation MethodThe Digit Analysis MethodThe Length-Dependent MethodHashing Functions in the Java Language69269269369369469469469520.3Resolving CollisionsChainingOpen Addressing69569569820.4Deleting Elements from A Hash TableDeleting from a Chained ImplementationDeleting from an Open AddressingImplementation701701Hash Tables in the Java Collections APIThe Hashtable ClassThe HashSet ClassThe HashMap ClassThe IdentityHashMap ClassThe WeakHashMap ClassLinkedHashSet and LinkedHashMap70370470470670770971020.5702xxiii
7112 Lewis FM ppi-xxviii.qxdxxiv2/5/1011:27 AMPage xxivC ON T E NTSChapter 21 Databases71721.1Introduction to Databases71821.2Establishing a Connection to a DatabaseObtaining A Database Driver72072021.3Creating and Altering Database TablesCreate TableAlter TableDrop Column72272372472521.4Querying The DatabaseShow Columns72572621.5Inserting, Viewing, and Updating(Modifying) DataInsertSelect . . . fromUpdate728729729734Deleting Data and Database TablesDeleting DataDeleting Database Tables73573573621.6Appendix A Glossary741Appendix B Number Systems775Place Value776Bases Higher Than 10778Conversions779Shortcut Conversions781Appendix C The Unicode Character Set787Appendix D Java Bitwise Operators791
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xxvC O NT ENT SAppendix E Java Modifiers797Java Visibility Modifiers798A Visibility Example798Other Java Modifiers799Appendix F Java Graphics801Coordinate Systems802Representing Color802Drawing Shapes803Polygons and Polylines812The Polygon Class813Appendix G Java Applets821Embedding Applets in HTML824More Applet Methods824GUIs in Applets829Appendix H Regular Expressions837Appendix I Javadoc DocumentationGenerator839Doc Comments840Tags841Files Generated841Appendix J Java Syntax845Index859xxv
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xxvi
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xxviiSecond EditionJavaFoundations Introduction toProgram Design & Data Structures
7112 Lewis FM ppi-xxviii.qxd2/5/1011:27 AMPage xxviii
Phrases like objects-first, objects-early, and objects-latecontinue to be bandied about by computing educators, despite the fact that the nuances of the pedagogy of the introductory sequence cannot be summed up so easily. We'll take this opportunity to discuss our approach.