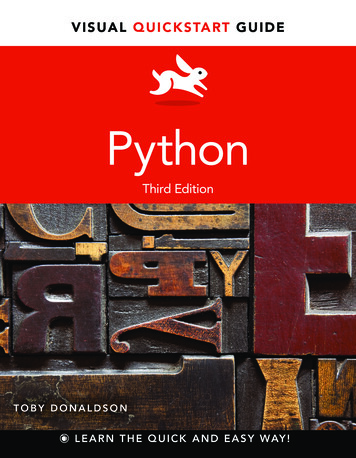
Transcription
V I S U A L Q U I C K S TA RT G U I D EPythonThird EditionLARRY ULLMANTOBY DONALDSONL E A R N T H E Q U I C K A N D E A S Y WAY !
V I S UA L Q U I C K S TA R T G U I D EPythonTOBY DONALDSONPeachpit Press
Visual QuickStart GuidePython, Third EditionToby DonaldsonPeachpit Presswww.peachpit.comTo report errors, please send a note to errata@peachpit.comPeachpit Press is a division of Pearson EducationCopyright 2014 by Toby DonaldsonEditor: Scout FestaProduction Editor: Katerina MaloneCompositor: David Van NessIndexer: Valerie Haynes PerryCover Design: RHDG / Riezebos Holzbaur Design Group, Peachpit PressInterior Design: Peachpit PressLogo Design: MINE www.minesf.comNotice of RightsAll rights reserved. No part of this book may be reproduced or transmitted in any form by any means,electronic, mechanical, photocopying, recording, or otherwise, without the prior written permission of thepublisher. For information on getting permission for reprints and excerpts, contact permissions@peachpit.com.Notice of LiabilityThe information in this book is distributed on an “As Is” basis, without warranty. While every precaution hasbeen taken in the preparation of the book, neither the author nor Peachpit shall have any liability to anyperson or entity with respect to any loss or damage caused or alleged to be caused directly or indirectly by theinstructions contained in this book or by the computer software and hardware products described in it.TrademarksVisual QuickStart Guide is a registered trademark of Peachpit Press, a division of Pearson Education.Many of the designations used by manufacturers and sellers to distinguish their products are claimed astrademarks. Where those designations appear in this book, and Peachpit was aware of a trademark claim,the designations appear as requested by the owner of the trademark. All other product names and servicesidentified throughout this book are used in editorial fashion only and for the benefit of such companies with nointention of infringement of the trademark. No such use, or the use of any trade name, is intended to conveyendorsement or other affiliation with this book.ISBN-13: 978-0-321-92955-6ISBN-10:0-321-92955-19 8 7 6 5 4 3 2 1Printed and bound in the United States of America
AcknowledgmentsThanks to Clifford Colby and Scout Festa for their expertise andpatience in bringing this edition of the book to life; to the many studentsat SFU who continue to teach me how best to learn Python; to JohnEdgar and the other computer science teachers at SFU with whom I’vehad the pleasure to work; and to Bonnie, Thomas, and Emily for recommending I avoid using the word blithering more than once in theseacknowledgments. And a special thank you to Guido van Rossum andthe rest of the Python community for creating a programming languagethat is so much fun to use.
Contents at a GlanceChapter 1Introduction to Programming . . . . . . . . . . . . . 1Chapter 2Arithmetic, Strings, and Variables . . . . . . . . . . 9Chapter 3Writing Programs . . . . . . . . . . . . . . . . . . . . 31Chapter 4Flow of Control . . . . . . . . . . . . . . . . . . . . . 43Chapter 5Functions . . . . . . . . . . . . . . . . . . . . . . . . . 67Chapter 6Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . 83Chapter 7Data Structures . . . . . . . . . . . . . . . . . . . . . 101Chapter 8Input and Output . . . . . . . . . . . . . . . . . . . . 123Chapter 9Exception Handling . . . . . . . . . . . . . . . . . . 143Chapter 10Object-Oriented Programming . . . . . . . . . . . 153Chapter 11Case Study: Text Statistics . . . . . . . . . . . . . . 177Appendix A Popular Python Packages . . . . . . . . . . . . . . . 195Appendix B Comparing Python 2 and Python 3 . . . . . . . . 199Index . . . . . . . . . . . . . . . . . . . . . . . . . . . . 203ivContents at a Glance
Table of ContentsChapter 1Introduction to Programming . . . . . . . . . . . . . . 1The Python Language . . .What Is Python Useful For?How Programmers Work . .Installing Python . . . . . .Chapter 2.2346.101113161719202224262829Writing Programs . . . . . . . . . . . . . . . . . . . . . 31Using IDLE’s Editor . . . . . . . . . .Compiling Source Code . . . . . . .Reading Strings from the Keyboard .Printing Strings on the Screen . . . .Source Code Comments . . . . . . .Structuring a Program . . . . . . . .Chapter 4.Arithmetic, Strings, and Variables . . . . . . . . . . . 9The Interactive Command ShellInteger Arithmetic . . . . . . . .Floating Point Arithmetic . . . .Other Math Functions . . . . .Strings . . . . . . . . . . . . . .String Concatenation . . . . . .Getting Help . . . . . . . . . . .Converting Between Types . .Variables and Values . . . . . .Assignment Statements . . . .How Variables Refer to ValuesMultiple Assignment . . . . . .Chapter 3.323536394142Flow of Control . . . . . . . . . . . . . . . . . . . . . . 43Boolean Logic . . . . . . . . .If-Statements . . . . . . . . .Code Blocks and IndentationLoops . . . . . . . . . . . . . .44495154Table of Contents v
Comparing For-Loops and While-Loops . . . . . . . . . 59Breaking Out of Loops and Blocks . . . . . . . . . . . . 64Loops Within Loops . . . . . . . . . . . . . . . . . . . . . 66Chapter 5Functions . . . . . . . . . . . . . . . . . . . . . . . . . . 67Calling Functions . . .Defining Functions . .Variable Scope . . . .Using a main FunctionFunction Parameters .Modules . . . . . . . .Chapter 6.Table of Contents.687073757680.8487899298.102103104108. 110. 113. 115. 118122Input and Output . . . . . . . . . . . . . . . . . . . . . 123Formatting Strings . . . . .String Formatting . . . . . .Reading and Writing Files .Examining Files and FoldersProcessing Text Files . . . .Processing Binary Files . .Reading Webpages . . . . .vi.Data Structures . . . . . . . . . . . . . . . . . . . . . . 101The type Command .Sequences . . . . . .Tuples . . . . . . . .Lists . . . . . . . . . .List Functions . . . .Sorting Lists . . . . .List ComprehensionsDictionaries . . . . .Sets . . . . . . . . . .Chapter 8.Strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83String Indexing . . . . . .Characters . . . . . . . . .Slicing Strings . . . . . . .Standard String FunctionsRegular Expressions . . .Chapter 7.124126128. 131134138. 141
Chapter 9Exception Handling . . . . . . . . . . . . . . . . . . 143Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . 144Catching Exceptions . . . . . . . . . . . . . . . . . . . 146Clean-Up Actions . . . . . . . . . . . . . . . . . . . . . 150Chapter 10Object-Oriented Programming . . . . . . . . . . . . 153Writing a Class . . .Displaying Objects .Flexible InitializationSetters and Getters .Inheritance . . . . . .Polymorphism . . . .Learning More . . . .Chapter 11.154156160162168. 171175Case Study: Text Statistics . . . . . . . . . . . . . . . 177Problem Description . . . . . . . . . . . . . . .Keeping the Letters We Want . . . . . . . . . .Testing the Code on a Large Data File . . . . .Finding the Most Frequent Words . . . . . . .Converting a String to a Frequency DictionaryPutting It All Together . . . . . . . . . . . . . .Exercises . . . . . . . . . . . . . . . . . . . . . .The Final Program . . . . . . . . . . . . . . . .178180182184187188190192Appendix A Popular Python Packages . . . . . . . . . . . . . . . 195Some Popular Packages . . . . . . . . . . . . . . . . . 196Appendix B Comparing Python 2 and Python 3 . . . . . . . . 199What’s New in Python 3 . . . . . . . . . . . . . . . . . 200Index . . . . . . . . . . . . . . . . . . . . . . . . . . . . 203Table of Contents vii
This page intentionally left blank
4Flow of ControlThe programs we’ve written so far arestraight-line programs that consist of asequence of Python statements executedone after the other. The flow of execution issimply a straight sequence of statements,with no branching or looping back to previous statements.In this chapter, we look at how to changethe order in which statements are executedby using if-statements and loops. Both areessential in almost any nontrivial program.Both if-statements and loops are controlledby logical expressions, and so the first partof this chapter will introduce the idea ofBoolean logic.Read the sample programs in this chaptercarefully. Take the time to try them out andmake your own modifications.In This ChapterBoolean Logic44If-Statements49Code Blocks and Indentation51Loops54Comparing For-Loops and While-Loops59Breaking Out of Loops and Blocks64Loops Within Loops66
Boolean LogicIn Python, as in most programming languages, decisions are made using Booleanlogic. Boolean logic is all about manipulating so-called truth values, which in Pythonare written True and False. Boolean logicis simpler than numeric arithmetic, and isa formalization of logical rules you alreadyknow.We combine Boolean values using fourmain logical operators (or logical connectives): not, and, or, and . All decisions that can be made by Python—or anycomputer language, for that matter—canbe made using these logical operators.Suppose that p and q are two Python variables each labeling Boolean values. Sinceeach has two possible values (True orFalse), altogether there are four differentsets of values for p and q (see the first twocolumns of Table 4.1). We can now definethe logical operators by specifying exactlywhat value they return for the differenttruth values of p and q. These kinds ofdefinitions are known as truth tables, andPython uses an internal version of them toevaluate Boolean expressions.TABLE 4.1 Truth Table for Basic Logical Operatorspqp qp ! qp and qp or qnot eTrueTrueFalseTrueTrueFalse44Chapter 4
Logical equivalenceLogical “or”Let’s start with . The expression p qis True only when p and q both have thesame truth value—that is, when p and qare either both True or both False. Theexpression p ! q tests if p and q are notthe same, and returns True only when theyhave different values.The Boolean expression p or q is Trueexactly when p is True or q is True, orwhen both are True. This is summarizedin the sixth column of Table 4.1. The onlyslightly tricky case is when both p and qare True. In this case, the expression p orq is True. False False False or FalseTrueFalse True False False or TrueFalseTrue True True True or FalseTrueTrue False ! False True or TrueFalseTrue True ! FalseTrue True ! TrueFalseLogical “and”The Boolean expression p and q is Trueonly when both p is True and q is True. Inevery other case it is False. The fifth column of Table 4.1 summarizes each case.Logical negationFinally, the Boolean expression not p isTrue when p is False, and False when pis True. It essentially flips the value of thevariable. not TrueFalse not FalseTrue False and FalseFalse False and TrueFalse True and FalseFalse True and TrueTrueFlow of Control 45
Evaluating larger BooleanexpressionsSince Boolean expressions are used tocontrol both if-statements and loops, it isimportant to understand how they are evaluated. Just as with arithmetic expressions,Boolean expressions use both brackets andoperator precedence to specify the order inwhich their sub-parts are evaluated.To evaluate a Booleanexpression with brackets:Suppose we want to evaluate the expression not (True and (False or True)).We can do it by following these steps: not (True and (False or True))Expressions in brackets are alwaysevaluated first, and so we first evaluate False or True, which is True.This makes the original expression equivalent to this simpler one:not (True and True). not (True and True)To evaluate this expression, we againevaluate the expression in bracketsfirst: True and True evaluates to True,which gives us the equivalent expression: not True. not TrueFinally, to evaluate this expression, wesimply look up the answer in the lastcolumn of Table 4.1: not True evaluatesto False. Thus, the entire expressionnot (True and (False or True))evaluates to False. You can easilycheck that this is the correct answer inPython itself: not (True and (False or True))False46Chapter 4
TABLE 4.2 Boolean Operator Priority(Highest to Lowest)p qp ! qnot pp and qTo evaluate a Booleanexpression without brackets:Suppose we want to evaluate the expression not True and False or True. This isthe same as the previous one, but this timethere are no brackets. p or qnot True and False or TrueWe first evaluate the operator withthe highest precedence, as listed inTable 4.2. In this case, not has the highest precedence, and so not True isevaluated first (the fact that it happensto be at the start of the expression is acoincidence). This simplifies the expression to False and False or True. False and False or TrueWe again evaluate the operator with thehighest precedence. According to Table4.2, and has higher precedence thanor, and so False and True is evaluated first. The expression simplifies toFalse or True. False or TrueThis final expression evaluates to True,which is found by looking up the answerin Table 4.1. Thus the original expression, False and not False or True,evaluates to True.Writing complicated Boolean expressionswithout brackets is usually a bad idea becausethey are hard to read and evaluate—not allprogrammers remember the order of precedence of Boolean operators!One exception is when you use the samelogical operator many times in a row. Then itis usually easier to read without the brackets.For example: (True or (False or (True or False)))True True or False or True or FalseTrueFlow of Control 47
Short-circuit evaluationThe definition of the logical operatorsgiven in Table 4.1 is the standard definitionyou would find in any logic textbook. However, like most modern programming languages, Python uses a simple trick calledshort-circuit evaluation to speed up theevaluation of some Boolean expressions.Consider the Boolean expression Falseand X, where X is any Boolean expression. It turns out that no matter whether Xis True or X is False, the entire expression is False. The reason is that the initialFalse makes the whole and-expressionFalse. The value of False and X does notdepend on X—it is always False. In suchcases, Python does not evaluate X at all—itsimply stops and returns the value False.This can speed up the evaluation of Boolean expressions.Similarly, Boolean expressions of the formTrue or X are always True, no matterthe value of X. The precise rules for howPython does short-circuiting are given inTable 4.3.Most of the time you can ignore shortcircuiting and just reap its performancebenefits. However, it is useful to remember that Python does this, since everyonce in a while it could be the source of asubtle bug.It’s possible to use the definitions of andand or from Table 4.3 to write short and trickycode that simulates if-statements (which wewill see in the next section). However, suchexpressions are usually quite difficult to read,so if you ever run across such expressions inother people’s Python code (you should neverput anything so ugly in your programs!), youmay need to refer to Table 4.3 to figure outexactly what they are doing.48Chapter 4TABLE 4.3 Definition of Boolean Operatorsin PythonOperationResultp or qif p is False, then q, else pp and qif p is False, then p, else q
If-StatementsIf-statements let you change the flow ofcontrol in a Python program. Essentially,they let you write programs that candecide, while the programming is running,whether or not to run one block of codeor another. Almost all nontrivial programsuse one or more if-statements, so they areimportant to understand.If/else-statementsSuppose you are writing a passwordchecking program. The user enters theirpassword, and if it is correct, you log themin to their account. If it is not correct, thenyou tell them they’ve entered the wrongpassword:# password1.pypwd input('What is the password? ')if pwd 'apple': # note use of # instead of print('Logging on .')else:print('Incorrect password.')print('All done!')It’s pretty easy to read this program: Ifthe string that pwd labels is 'apple', thena login message is printed. But if pwd isanything other than 'apple', the messageincorrect password is printed.An if-statement always begins with thekeyword if. It is then (always) followedby a Boolean expression called theif-condition, or just condition for short.After the if-condition comes a colon (:).As we will see, Python uses the : tokento mark the end of conditions inif-statements, loops, and functions.Flow of Control 49
Everything from the if to the : is referredto as the if-statement header. If the condition in the header evaluates to True,then the statement print('Loggingon .') is immediately executed, andprint('Incorrect password.') isskipped and never executed.If the condition in the header evaluates to False, then print('Logging on.') is skipped, and only the statementprint('Incorrect password.') isexecuted.In all cases, the final print('All done!')statement is executed.We will often refer to the entire multilineif structure as a single if-statement.You must put at least one space afterthe if keyword.The if keyword, the condition, and theterminating : must appear all on one linewithout breaks.The else-block of an if-statement isoptional. Depending on the problem you aresolving, you may or may not need one.The general structure of an if/else-statementis shown in A.A This flow chart shows the general format and behavior of an if/else-statement.The code blocks can consist of any number of Python statements (even otherif-statements!).50Chapter 4
Code Blocks andIndentationOne of the most distinctive features ofPython is its use of indentation to markblocks of code. Consider the if-statementfrom our password-checking program:if pwd 'apple':print('Logging on .')else:print('Incorrect password.')print('All done!')The lines print('Logging on .') andprint('Incorrect password.') are twoseparate code blocks. These ones happento be only a single line long, but Pythonlets you write code blocks consisting ofany number of statements.To indicate a block of code in Python, youmust indent each line of the block by thesame amount. The two blocks of code inour example if-statement are both indentedfour spaces, which is a typical amount ofindentation for Python.In most other programming languages,indentation is used only to help makethe code look pretty. But in Python, it isrequired for indicating what block of codea statement belongs to. For instance, thefinal print('All done!') is not indented,and so is not part of the else-block.IDLE is designed to automatically indentcode for you. For instance, pressing Returnafter typing the : in an if-header automaticallyindents the cursor on the next line.The amount of indentation matters: Amissing or extra space in a Python block couldcause an error or unexpected behavior. Statements within the same block of code need tobe indented at the same level.Programmers familiar with other languagesoften bristle at the thought that indentationmatters: Many programmers like the freedom to format their code how they please.However, Python’s indentation rules followa style that many programmers already useto make their code readable. Python simplytakes this idea one step further and givesmeaning to the indentation.Flow of Control 51
If/elif-statementsAn if/elif-statement is a generalized ifstatement with more than one condition.It is used for making complex decisions.For example, suppose an airline has thefollowing “child” ticket rates: Kids 2 yearsold or younger fly for free, kids older than 2but younger than 13 pay a discounted childfare, and anyone 13 years or older pays aregular adult fare. This program determineshow much a passenger should pay:# airfare.pyAfter Python gets age from the user, itenters the if/elif-statement and checkseach condition one after the other in theorder they are given. So first it checks ifage is less than 2, and if so, it indicatesthat the flying is free and jumps out of theelif-condition. If age is not less than 2, thenit checks the next elif-condition to see ifage is between 2 and 13. If so, it prints theappropriate message and jumps out of theif/elif-statement. If neither the if-conditionnor the elif-condition is True, then itexecutes the code in the else-block.age int(input('How old are you? '))if age 2:print(' free')elif 2 age 13:print(' child fare)else:print('adult fare')52Chapter 4elif is short for else if, and you can useas many elif-blocks as needed.Each of the code blocks in an if/elifstatement must be consistently indented thesame amount.As with a regular if-statement, the elseblock is optional. In an if/elif-statement withan else-block, exactly one of the if/elif-blockswill be executed. If there is no else-block, thenit is possible that none of the conditions areTrue, in which case none of the if/elif-blocksare executed.
Conditional expressionsPython has one more logical operator thatsome programmers like (and some don’t!).It’s essentially a shorthand notation for ifstatements that can be used directly withinexpressions. Consider this code:food input("What's your favorite food? ")reply 'yuck' if food 'lamb''yum' elseThe expression on the right-hand side of in the second line is called a conditionalexpression, and it evaluates to either'yuck' or 'yum'. It’s equivalent to thefollowing:food input("What's your favorite food? ")if food 'lamb':reply 'yuck'else:reply 'yum'Conditional expressions are usually shorterthan the corresponding if/else-statements,although not always as flexible or easyto read. In general, you should use themwhen they make your code simpler.Flow of Control 53
LoopsNow we turn to loops, which are used torepeatedly execute blocks of code. Pythonhas two main kinds of loops: for-loops andwhile-loops. For-loops are generally easierto use and less error prone than whileloops, although not quite as flexible.For-loopsThe basic for-loop repeats a given blockof code some specified number of times.For example, this snippet of code prints thenumbers 0 to 9 on the screen:# count10.pyfor i in range(10):print(i)The first line of a for-loop is called the forloop header. A for-loop always begins withthe keyword for. After that comes the loopvariable, in this case i. Next is the keyword in, typically (but not always) followedby range(n) and a terminating : token. Afor-loop repeats its body, the code blockunderneath it, exactly n times.Each time the loop executes, the loopvariable i is set to be the next value. Bydefault, the initial value of i is 0, and itgoes up to n - 1 (not n!) by ones. Startingnumbering at 0 instead of 1 might seemunusual, but it is common in programming.If you want to change the starting value ofthe loop, add a starting value to range:for i in range(5, 10):print(i)This prints the numbers from 5 to 9.54Chapter 4Lingo AlertProgrammers often use the variable ibecause it is short for index, and is alsocommonly used in mathematics. Whenwe start using loops within loops, it iscommon to use j and k as other loopvariable names.
If you want to print the numbers from 1to 10 (instead of 0 to 9), there are two commonways of doing so. One is to change the startand end of the range:for i in range(1, 11):print(i)Or, you can add 1 to i inside the loop body:for i in range(10):print(i 1)If you would like to print numbers inreverse order, there are again two standardways of doing so. The first is to set the rangeparameters like this:for i in range(10, 0, -1):print(i)Notice that the first value of range is 10, thesecond value is 0, and the third value, calledthe step, is 1. Alternatively, you can use a simpler range and modify i in the loop body:for i in range(10):print(10 - i)For-loops are actually more general thandescribed in this section: They can be usedwith any kind of iterator, which is a specialkind of programming object that returnsvalues. For instance, we will see later that forloops are the easiest way to read the lines ofa text file.Flow of Control 55
While-loopsThe second kind of Python loop is a whileloop. Consider this program:# while10.pyi 0while i 10:print(i)i i 1 # add 1 to iThis prints out the numbers from 0 to 9 onthe screen. It is noticeably more complicated than a for-loop, but it is also moreflexible.The while-loop itself begins on the linebeginning with the keyword while; this lineis called the while-loop header, and theindented code underneath it is called thewhile-loop body. The header always startswith while and is followed by the whileloop condition. The condition is a Booleanexpression that returns True or False.The flow of control through a while-loopgoes like this: First, Python checks if theloop condition is True or False. If it’sTrue, it executes the body; if it’s False, itskips over the body (that is, it jumps out ofthe loop) and runs whatever statementsappear afterward. When the conditionis True, the body is executed, and thenPython checks the condition again. As longas the loop condition is True, Python keepsexecuting the loop. B shows a flow chartfor this program.The very first line of the sample programis i 0, and in the context of a loop it isknown as an initialization statement, oran initializer. Unlike with for-loops, whichautomatically initialize their loop variable, itis the programmer’s responsibility to giveinitial values to any variables used by awhile-loop.56Chapter 4B This is a flow chart for code that counts from 0to 9. Notice that when the loop condition is False(that is, the no branch is taken in the decision box),the arrow does not go into a box. That’s becausein our sample code there is nothing after thewhile-loop.
The last line of the loop body is i i 1.As it says in the source code comment, thisline causes i to be incremented by 1. Thus,i increases as the loop executes, whichguarantees that the loop will eventuallystop. In the context of a while-loop, thisline is called an increment, or incrementer,since its job is to increment the loopvariable.The general form of a while-loop is shownin the flow chart of C.Even though almost all while-loops needan initializer and an incrementer, Pythondoes not require that you include them. Itis entirely up to you, the programmer, toremember these lines. Even experiencedprogrammers find that while-loop initializers and incrementers are a commonsource of errors.C A flow chart for the general form of a while-loop. Note that theincrementer is not shown explicitly: It is embedded somewhere inbody block, often (but not always) at the end of that block.Flow of Control 57
While-loops are extremely flexible. Youcan put any code whatsoever before a whileloop to do whatever kind of initialization isnecessary. The loop condition can be anyBoolean expression, and the incrementer canbe put anywhere within the while-loop body,and it can do whatever you like.A loop that never ends is called an infinite loop. For instance, this runs forever:while True:print('spam')Some programmers like to use infiniteloops as a quick way to write a loop. However,this is generally considered to be poor stylebecause such loops often become complexand hard to understand.Many Python programmers try to use forloops whenever possible and use while-loopsonly when absolutely necessary.While-loops can be written with an elseblock. However, this unusual feature is rarelyused in practice, so we haven’t discussedit. If you are curious, you can read about itin the online Python documentation—forexample, http://docs.python.org/3/reference/compound stmts.html.58Chapter 4
Comparing For-Loopsand While-LoopsLet’s take a look at a few examples of howfor-loops and while-loops can be used tosolve the same problems. Plus we’ll see asimple program that can’t be written usinga for-loop.Calculating factorialsFactorials are numbers of the form 1 2 3 n, and they tell you how manyways n objects can be arranged in a line.For example, the letters ABCD can bearranged in 1 2 3 4 24 differentways. Here’s one way to calculate factorials using a for-loop:# forfact.pyn int(input('Enter an integer 0: '))fact 1for i in range(2, n 1):fact fact * iprint(str(n) ' factorial is ' str(fact))Here’s another way to do it using awhile-loop:# whilefact.pyn int(input('Enter an integer 0: '))fact 1i 2while i n:fact fact * ii i 1print(str(n) ' factorial is ' str(fact))continues on next pageFlow of Control 59
Both of these programs behave the samefrom the user’s perspective, but the internals are quite different. As is usually thecase, the while-loop version is a little morecomplicated than the for-loop version.In mathematics, the notation n! is used toindicate factorials. For example, 4! 1 2 3 4 24. By definition, 0! 1. Interestingly, thereis no simple formula for calculating factorials.Python has no maximum integer, so youcan use these pro
Visual QuickStart Guide Python, Third Edition Toby Donaldson Peachpit Press www.peachpit.com To