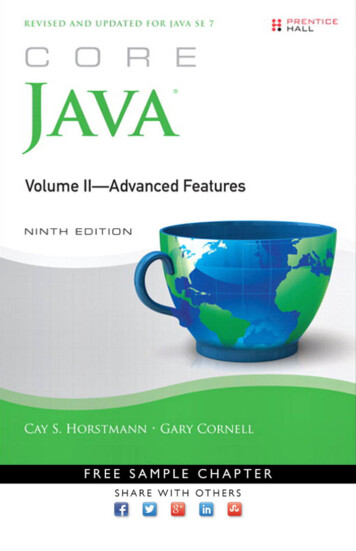
Transcription
Core Java Volume II—Advanced FeaturesNinth Edition
This page intentionally left blank
Core Java Volume II—AdvancedFeaturesNinth EditionCay S. HorstmannGary CornellUpper Saddle River, NJ Boston Indianapolis San FranciscoNew York Toronto Montreal London Munich Paris MadridCapetown Sydney Tokyo Singapore Mexico City
Many of the designations used by manufacturers and sellers to distinguish their products are claimed astrademarks. Where those designations appear in this book, and the publisher was aware of a trademark claim,the designations have been printed with initial capital letters or in all capitals.Oracle and Java are registered trademarks of Oracle and/or its affiliates. Other names may be trademarks of theirrespective owners.The authors and publisher have taken care in the preparation of this book, but make no expressed or impliedwarranty of any kind and assume no responsibility for errors or omissions. No liability is assumed for incidentalor consequential damages in connection with or arising out of the use of the information or programs containedherein.This document is provided for information purposes only and the contents hereof are subject to change withoutnotice. This document is not warranted to be error-free, nor subject to any other warranties or conditions, whetherexpressed orally or implied in law, including implied warranties and conditions of merchantability or fitness fora particular purpose. We specifically disclaim any liability with respect to this document and no contractualobligations are formed either directly or indirectly by this document. This document may not be reproduced ortransmitted in any form or by any means, electronic or mechanical, for any purpose, without our prior writtenpermission.The publisher offers excellent discounts on this book when ordered in quantity for bulk purchases or special sales,which may include electronic versions and/or custom covers and content particular to your business, traininggoals, marketing focus, and branding interests. For more information, please contact:U.S. Corporate and Government Sales(800) 382-3419corpsales@pearsontechgroup.comFor sales outside the United States, please contact:International Salesinternational@pearson.comVisit us on the Web: informit.com/phLibrary of Congress Cataloging-in-Publication Data:Horstmann, Cay S., 1959Core Java / Cay S. Horstmann, Gary Cornell.—Ninth edition.pages cmIncludes index.ISBN 978-0-13-708189-9 (v. 1 : pbk. : alk. paper) 1. Java (Computer programlanguage) I. Cornell, Gary. II. Title.QA76.73.J38H6753 2013005.13'3—dc232012035397Copyright 2013 Oracle and/or its affiliates. All rights reserved.500 Oracle Parkway, Redwood Shores, CA 94065Printed in the United States of America. This publication is protected by copyright, and permission must beobtained from the publisher prior to any prohibited reproduction, storage in a retrieval system, or transmissionin any form or by any means, electronic, mechanical, photocopying, recording, or likewise. To obtain permissionto use material from this work, please submit a written request to Pearson Education, Inc., Permissions Department,One Lake Street, Upper Saddle River, New Jersey 07458, or you may fax your request to (201) 236-3290.ISBN-13: 978-0-13-708160-8ISBN-10:0-13-708160-XText printed in the United States on recycled paper at Edwards Brothers Malloy in Ann Arbor, Michigan.Second printing, September 2013
ContentsPreface . xvAcknowledgments . xixChapter 1: Streams and Files . 11.11.21.31.41.51.6Streams . 21.1.1 Reading and Writing Bytes . 21.1.2 The Complete Stream Zoo . 41.1.3 Combining Stream Filters . 9Text Input and Output . 131.2.1 How to Write Text Output . 131.2.2 How to Read Text Input . 161.2.3 Saving Objects in Text Format . 161.2.4 Character Sets . 20Reading and Writing Binary Data . 251.3.1 Random-Access Files . 28ZIP Archives . 33Object Streams and Serialization . 361.5.1 Understanding the Object Serialization File Format . 421.5.2 Modifying the Default Serialization Mechanism . 481.5.3 Serializing Singletons and Typesafe Enumerations . 501.5.4 Versioning . 521.5.5 Using Serialization for Cloning . 54Working with Files . 571.6.1 Paths . 571.6.2 Reading and Writing Files . 601.6.3 Copying, Moving, and Deleting Files . 611.6.4 Creating Files and Directories . 621.6.5 Getting File Information . 631.6.6 Iterating over the Files in a Directory . 641.6.7 ZIP File Systems . 67v
viContents1.71.8Memory-Mapped Files .1.7.1 The Buffer Data Structure .1.7.2 File Locking .Regular Expressions .68777981Chapter 2: XML . 932.12.22.32.42.52.62.72.8Introducing XML . 942.1.1 The Structure of an XML Document . 96Parsing an XML Document . 99Validating XML Documents . 1132.3.1 Document Type Definitions . 1142.3.2 XML Schema . 1222.3.3 A Practical Example . 125Locating Information with XPath . 140Using Namespaces . 147Streaming Parsers . 1502.6.1 Using the SAX Parser . 1502.6.2 Using the StAX Parser . 156Generating XML Documents . 1592.7.1 Documents without Namespaces . 1592.7.2 Documents with Namespaces . 1602.7.3 Writing Documents . 1612.7.4 An Example: Generating an SVG File . 1612.7.5 Writing an XML Document with StAX . 164XSL Transformations . 173Chapter 3: Networking . 1853.13.23.33.4Connecting to a Server .3.1.1 Socket Timeouts .3.1.2 Internet Addresses .Implementing Servers .3.2.1 Serving Multiple Clients .3.2.2 Half-Close .Interruptible Sockets .Getting Web Data .3.4.1 URLs and URIs .185190192194197201202210210
Contents3.53.4.2 Using a URLConnection to Retrieve Information . 2123.4.3 Posting Form Data . 222Sending E-Mail . 230Chapter 4: Database Programming . 2354.14.24.34.44.54.64.74.84.9The Design of JDBC .4.1.1 JDBC Driver Types .4.1.2 Typical Uses of JDBC .The Structured Query Language .JDBC Configuration .4.3.1 Database URLs .4.3.2 Driver JAR Files .4.3.3 Starting the Database .4.3.4 Registering the Driver Class .4.3.5 Connecting to the Database .Executing SQL Statements .4.4.1 Managing Connections, Statements, and Result Sets .4.4.2 Analyzing SQL Exceptions .4.4.3 Populating a Database .Query Execution .4.5.1 Prepared Statements .4.5.2 Reading and Writing LOBs .4.5.3 SQL Escapes .4.5.4 Multiple Results .4.5.5 Retrieving Autogenerated Keys .Scrollable and Updatable Result Sets .4.6.1 Scrollable Result Sets .4.6.2 Updatable Result Sets .Row Sets .4.7.1 Constructing Row Sets .4.7.2 Cached Row Sets .Metadata .Transactions .4.9.1 Save Points .4.9.2 Batch Updates 69271272273274274277281282282286296297298vii
viiiContents4.9.3 Advanced SQL Types . 3004.10 Connection Management in Web and Enterprise Applications . 302Chapter 5: Internationalization . 3055.15.25.35.45.55.65.75.8Locales .Number Formats .5.2.1 Currencies .Date and Time .Collation .5.4.1 Collation Strength .5.4.2 Decomposition .Message Formatting .5.5.1 Choice Formats .Text Files and Character Sets .5.6.1 Character Encoding of Source Files .Resource Bundles .5.7.1 Locating Resource Bundles .5.7.2 Property Files .5.7.3 Bundle Classes .A Complete Example hapter 6: Advanced Swing . 3636.16.2Lists .6.1.1 The JList Component .6.1.2 List Models .6.1.3 Inserting and Removing Values .6.1.4 Rendering Values .Tables .6.2.1 A Simple Table .6.2.2 Table Models .6.2.3 Working with Rows and Columns .6.2.3.1 Column Classes .6.2.3.2 Accessing Table Columns .6.2.3.3 Resizing Columns .6.2.3.4 Resizing Rows .6.2.3.5 Selecting Rows, Columns, and Cells .364364370375377381382386390390392392393394
Contents6.36.46.56.66.2.3.6 Sorting Rows .6.2.3.7 Filtering Rows .6.2.3.8 Hiding and Displaying Columns .6.2.4 Cell Rendering and Editing .6.2.4.1 Rendering the Header .6.2.4.2 Cell Editing .6.2.4.3 Custom Editors .Trees .6.3.1 Simple Trees .6.3.1.1 Editing Trees and Tree Paths .6.3.2 Node Enumeration .6.3.3 Rendering Nodes .6.3.4 Listening to Tree Events .6.3.5 Custom Tree Models .Text Components .6.4.1 Change Tracking in Text Components .6.4.2 Formatted Input Fields .6.4.2.1 Integer Input .6.4.2.2 Behavior on Loss of Focus .6.4.2.3 Filters .6.4.2.4 Verifiers .6.4.2.5 Other Standard Formatters .6.4.2.6 Custom Formatters .6.4.3 The JSpinner Component .6.4.4 Displaying HTML with the JEditorPane .Progress Indicators .6.5.1 Progress Bars .6.5.2 Progress Monitors .6.5.3 Monitoring the Progress of Input Streams .Component Organizers and Decorators .6.6.1 Split Panes .6.6.2 Tabbed Panes .6.6.3 Desktop Panes and Internal Frames .6.6.4 Cascading and Tiling .6.6.5 Vetoing Property Settings 527531ix
xContents6.6.5.16.6.5.26.6.6.3Dialogs in Internal Frames . 533Outline Dragging . 534Layers . 543Chapter 7: Advanced AWT . The Rendering Pipeline .Shapes .7.2.1 Using the Shape Classes .Areas .Strokes .Paint .Coordinate Transformations .Clipping .Transparency and Composition .Rendering Hints .Readers and Writers for Images .7.10.1 Obtaining Readers and Writers for Image File Types .7.10.2 Reading and Writing Files with Multiple Images .Image Manipulation .7.11.1 Constructing Raster Images .7.11.2 Filtering Images .Printing .7.12.1 Graphics Printing .7.12.2 Multiple-Page Printing .7.12.3 Print Preview .7.12.4 Print Services .7.12.5 Stream Print Services .7.12.6 Printing Attributes .The Clipboard .7.13.1 Classes and Interfaces for Data Transfer .7.13.2 Transferring Text .7.13.3 The Transferable Interface and Data Flavors .7.13.4 Building an Image Transferable .7.13.5 Transferring Java Objects via the System Clipboard .7.13.6 Using a Local Clipboard to Transfer Object References .Drag and Drop 36637647649659664664672674674678680685689689
Contents7.14.1 Data Transfer Support in Swing .7.14.2 Drag Sources .7.14.3 Drop Targets .7.15 Platform Integration .7.15.1 Splash Screens .7.15.2 Launching Desktop Applications .7.15.3 The System Tray .691696699707708713719Chapter 8: JavaBeans Components . 7258.18.28.38.48.58.68.78.88.9Why Beans? .The Bean-Writing Process .Using Beans to Build an Application .8.3.1 Packaging Beans in JAR Files .8.3.2 Composing Beans in a Builder Environment .Naming Patterns for Bean Properties and Events .Bean Property Types .8.5.1 Simple Properties .8.5.2 Indexed Properties .8.5.3 Bound Properties .8.5.4 Constrained Properties .BeanInfo Classes .Property Editors .8.7.1 Writing Property Editors .8.7.1.1 String-Based Property Editors .8.7.1.2 GUI-Based Property Editors .Customizers .8.8.1 Writing a Customizer Class .JavaBeans Persistence .8.9.1 Using JavaBeans Persistence for Arbitrary Data .8.9.1.1 Writing a Persistence Delegateto Construct an Object .8.9.1.2 Constructing an Object from Properties .8.9.1.3 Constructing an Object with a Factory Method .8.9.1.4 Postconstruction Work .8.9.1.5 Transient Properties .8.9.2 A Complete Example for JavaBeans Persistence 70772779784784786787787788791xi
xiiContentsChapter 9: Security . 8039.19.29.39.49.59.69.7Class Loaders .9.1.1 The Class Loader Hierarchy .9.1.2 Using Class Loaders as Namespaces .9.1.3 Writing Your Own Class Loader .Bytecode Verification .Security Managers and Permissions .9.3.1 Java Platform Security .9.3.2 Security Policy Files .9.3.3 Custom Permissions .9.3.4 Implementation of a Permission Class .User Authentication .9.4.1 JAAS Login Modules .Digital Signatures .9.5.1 Message Digests .9.5.2 Message Signing .9.5.3 Verifying a Signature .9.5.4 The Authentication Problem .9.5.5 Certificate Signing .9.5.6 Certificate Requests .Code Signing .9.6.1 JAR File Signing .9.6.2 Software Developer Certificates .Encryption .9.7.1 Symmetric Ciphers .9.7.2 Key Generation .9.7.3 Cipher Streams .9.7.4 Public Key Ciphers 68870872873873878880881882887888Chapter 10: Scripting, Compiling, and Annotation Processing . 89310.1 Scripting for the Java Platform .10.1.1 Getting a Scripting Engine .10.1.2 Script Evaluation and Bindings .10.1.3 Redirecting Input and Output .10.1.4 Calling Scripting Functions and Methods .10.1.5 Compiling a Script .894894895898899901
Contents10.210.310.410.510.610.710.1.6 An Example: Scripting GUI Events .The Compiler API .10.2.1 Compiling the Easy Way .10.2.2 Using Compilation Tasks .10.2.3 An Example: Dynamic Java Code Generation .Using Annotations .10.3.1 An Example: Annotating Event Handlers .Annotation Synt
Core Java Volume II—Advanced . The publisher offers excellent discounts on this book when ordered in quantity for bulk purchases or special sales, which may include electronic versions and/or custom covers and content particular to your business, tra