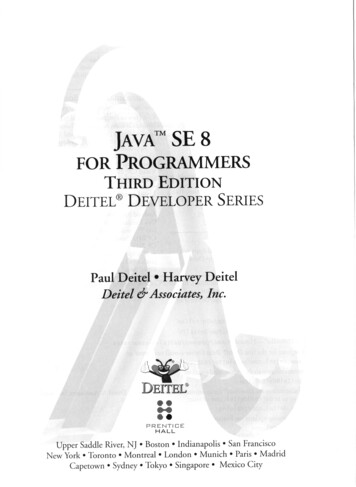
Transcription
JAVA SE 8FOR PROGRAMMERSTHIRD EDITIONDEITEL DEVELOPER SERIESPaul Deitel Harvey DeitelDeitel & Associates, Inc.D'C'TT'n'THil1 XIIJ-JPRENTICEHALLUpper Saddle River, NJ Boston Indianapolis San FranciscoNew York Toronto Montreal London Munich Paris MadridCapetown Sydney Tokyo Singapore Mexico City
ContentsForewordPrefaceBefore You BeginIIntroduction to Java and Test-Driving aJava Applicationl.i1.2IntroductionObject Technology Concepts1.2.1The Automobile as an Object1.2.2Methods and ClassesInstantiation1.2.31.2.4ReuseMessages and Method Calls1.2.51.2.6Attributes and Instance VariablesEncapsulation and Information Hiding1.2.71.2.8InheritanceInterfaces1.2.91.2.10 Object-Oriented Analysis and Design (OOAD)1.2.11 The UML (Unified Modeling Language)Open .Source SoftwareJavaA Typical Java Development EnvironmentTest-D riving a Java ApplicationSoftware TechnologiesKeepin g Up-to-Date with Information ction to Java Applications;Input/Output and OperatorsIntroductionYour First Program in Java: Printing a Line of TextModifying Your First Java ProgramDisplaying Text with 628
vüiContents2.52.62.72.8Another Application: Adding IntegersArithmeticDecision Making: Equality and Relational OperatorsWrap-Up293334373Introduction to Classes, Objects,Methods and Strings383.13.2IntroductionInstance Variables, set Methods and get Methods3.2.1 Account Class with an Instance Variable, a set Method anda get Method3.2.2 AccountTest Class That Creates and Uses an Object ofClass Account3.2.3Compiling and Executing an App with Multiple Classes3.2.4Account UML Class Diagram with an Instance Variable andset and get Methods3.2.5 Additional Notes on Class AccountTest3.2.6Software Engineering with pri vate Instance Variables andp u b 1 i c set and get MethodsPrimitive Types vs. Reference TypesAccount Class: Initializing Objects with Constructors3.4.1Declaring an Account Constructor for Custom Object Initialization3.4.2Class AccountTest: Initializing Account Objects WhenThey're CreatedAccount Class with a Balance; Floating-Point Numbers3.5.1Account Class with a bal ance Instance Variable of Type doubl e3.5.2 AccountTest Class to Use Class AccountWrap-Up3.33.43.53.64Control Statements: Part I; Assignment, and — 13IntroductionControl Structuresi f Single-Selection Statementi f . . . e l s e Double-Selection StatementStudent Class: Nested if.else Statementswhile Repetition StatementCounter-Controlled RepetitionSentinel-Controlled RepetitionNested Control StatementsCompound Assignment OperatorsIncrement and Decrement OperatorsPrimitive TypesWrap-Up 47981818485
Contentsix5Control Statements: Part 2; Logical tionEssentials of Counter-Controlled Repetitionfor Repetition StatementExamples Using the for Statementdo.while Repetition Statementswitch Multiple-Selection StatementClass AutoPolicy Case Study: Strings in switch Statementsbreak and continue StatementsLogical OperatorsWrap-Up8787889297981041081101156Methods: A Deeper ctionProgram Modules in Javastati c Methods, s t a t i c Fields and Class MathDeclaring Methods with Multiple ParametersNotes on Declaring and Using MethodsArgument Promotion and CastingJava API PackagesCase Study: Secure Random-Number GenerationCase Study: A Game of Chance; Introducing enum TypesScope of DeclarationsMethod OverloadingWrap-Up7Arrays and ArrayLists7.17.27.37.4IntroductionArraysDeclaring and Creating ArraysExamples Using Arrays7.4.1Creating and Initializing an Array7.4.2Using an Array Initializer7.4.3 Calculating the Values to Store in an Array7.4.4Summing the Elements of an Array7.4.5Using Bar Charts to Display Array Data Graphically7.4.6 Using the Elements of an Array as Counters7.4.7Using Arrays to Analyze Survey ResultsException Handling: Processing the Incorrect Response7.5-1 The try Statement7.5.2Executing the catch Block7.5.3toString Method of the Exception ParameterCase Study: Card Shuffling and Dealing SimulationEnhanced for 57158158163
sing Arrays to MethodsPass-By-Value vs. Pass-By-ReferenceCase Study: Class GradeBook Using an Array to Store GradesMultidimensional ArraysCase Study: Class GradeBook Using a Two-Dimensional ArrayVariable-Length Argument ListsUsing Command-Line ArgumentsClass ArraysIntroduction to Collections and Class ArrayListWrap-Up8Classes and Objects: A Deeper 48.158.168.17IntroductionTi me Class Case StudyControlling Access to MembersReferring to the Current Object's Members with the thi s ReferenceTi me Class Case Study: Overloaded ConstructorsDefault and No-Argument ConstructorsNotes on Set and Get MethodsCompositionenum TypesGarbage Collections t a t i c Class Memberss t a t i c Importf i n a l Instance VariablesTime Class Case Study: Creating PackagesPackage AccessUsing BigDeci mal for Precise Monetary CalculationsWrap-Up9Object-Oriented Programming: Inheritance9.19.29.39.4IntroductionSuperclasses and Subclassesp r o t e c t e d MembersRelationship Between Superclasses and Subclasses9.4.1Creating and Using a Commi ssi onEmpl oyee Class9.4.2Creating and Using a BasePlusCommi s s i onEmpl oyee Class9.4.3Creating a Commi ssi onEmpl oyee—BasePlusCommi ssi onEmpl oyeeInheritance Hierarchy9.4.4Commi ssi onEmpl oyee-BasePl usCommi ssi onEmpl oyee InheritanceHierarchy Using p r o t e c t e d Instance Variables9.4.5Commi ssi onEmpl oyee—BasePl usCommi ssi onEmpl oyee InheritanceHierarchy Using p r i v a t e Instance VariablesConstructors in 235236238239239245250253256261
Contents9.69.7Class ObjectWrap-Upxi26126210 Object-Oriented Programming: Polymorphismand hism ExamplesDemonstrating Polymorphic BehaviorAbstract Classes and MethodsCase Study: Payroll System Using Polymorphism10.5.1 Abstract Superclass Employee10.5.2 Concrete Subclass Sal ariedEmployee10.5.3 Concrete Subclass Hourly Employee10.5.4 Concrete Subclass CommissionEmployee10.5.5 Indirect Concrete Subclass BasePlusCommissionEmployee10.5.6 Polymorphic Processing, Operator i nstanceof and Downcasting10.6 Allowed Assignments Between Superclass and Subclass Variables10.7 fi nal Methods and Classes10.8 A Deeper Explanation of Issues with Calling Methods from Constructors10.9 Creating and Using Interfaces10.9.1 Developing a Payabl e Hierarchy10.9.2 Interface Payabl e10.9.3 Class Invoice10.9.4 Modifying Class Employee to Implement Interface Payable10.9.5 Modifying Class Sal ariedEmployee for Use in the PayableHierarchy10.9.6 Using Interface Payable to Process Invoices and EmployeesPolymorphically10.9.7 Some Common Interfaces of the Java API10.10 Java SE 8 Interface Enhancements10.10.1 d e f a u l t Interface Methods10.10.2 s t a t i c Interface Methods10.10.3 Functional Interfaces10.11 Wrap-UpI I Exception Handling: A Deeper e: Divide by Zero without Exception HandlingException Handling: ArithmeticExceptions andInputMismatchExceptionsWhen to Use Exception HandlingJava Exception Hierarchyf i n a l l y BlockStack Unwinding and Obtaining Information from an Exception 08314314317322
xii11.811.911.1011.1111.1211.13ContentsChained ExceptionsDeclaring New Exception TypesPreconditions and PostconditionsAssertionstry-with-Resources: Automatic Resource DeallocationWrap-Up12 Swing GUI Components: Part roductionJava's Nimbus Look-and-FeelSimple GUI-Based Input/Output with JOptionPaneOverview of Swing ComponentsDisplaying Text and Images in a WindowText Fields and an Introduction to Event Handling with Nested ClassesCommon GUI Event Types and Listener InterfacesH o w Event Handling WorksJButtonButtons That Maintain State12.10.1 JCheckBox12.10.2 JRadioButtonJComboBox; Using an Anonymous Inner Class for Event HandlingJListMultiple-Selection ListsMouse Event HandlingAdapter ClassesJPanel Subclass for Drawing with the MouseKey Event HandlingIntroduction to Layout Managers12.18.1 Flow/Layout12.18.2 BorderLayout12.18.3 GridLayoutUsing Panels to Manage More Complex LayoutsDTextAreaWrap-UpI 3 Graphics and Java Graphics Contexts and Graphics ObjectsColor ControlManipulating FontsDrawing Lines, Rectangles and OvalsDrawing ArcsDrawing Polygons and PolylinesJava 2 D 397398401402403405406413418422425428435
Contents14 Strings, Characters and Regular Expressionsxiii43614.114.2IntroductionFundamentals of Characters and Strings43743714.3Class String14.3.1 String Constructors14.3.2 String Methods length, charAt and 44544744844814.414.514.614.714.8Comparing StringsLocating Characters and Substrings in StringsExtracting Substrings from StringsConcatenating StringsMiscellaneous String Methods14.3.8 String Method valueOfClass StringBuilder14.4.1 StringBuilder Constructors14.4.2 StringBuilder Methods length, capacity, setLength andensureCapacity14.4.3 StringBuilder Methods charAt, setCharAt, getCharsand reverse14.4.4 StringBuilder append Methods45445514.4.5 StringBuilder Insertion and Deletion MethodsClass CharacterTokenizing StringsRegular Expressions, Class Pattern and Class MatcherWrap-Up45745846346447315 Files, Streams and Object Serialization15.115.215.315.415.5IntroductionFiles and StreamsUsing NIO Classes and Interfaces to Get File and Directory InformationSequential-Access Text Files15.4.1 Creating a Sequential-Access Text File15.4.2 Reading Data from a Sequential-Access Text File15.4.3 Case Study: A Credit-Inquiry Program15.4.4 Updating Sequential-Access FilesObject Serialization15.5.1 Creating a Sequential-Access File Using Object Serialization15.5.2 Reading and Deserializing Data from a Sequential-Access 9815.6Opening Files with J Fi leChooser50015.7(Optional) Additional java. i o Classes15.7.1 Interfaces and Classes for Byte-Based Input and Output15.7.2 Interfaces and Classes for Character-Based Input and OutputWrap-Up50350350550615.8
xivContents16 Generic tions OverviewType-Wrapper ClassesAutoboxing and Auto-UnboxingInterface C o l l e c t i o n and Class C o l l e c t i o n sLists16.6.1 ArrayLi s t and I t e r a t o r16.6.2 LinkedListCollections Methods16.7.1 M e t h o d s o r t16.7.2 Method s h u f f l e16.7.3 Methods reverse, f i l l , copy, max and min16.7.4 Method binarySearch16.7-5 Methods addAl 1, frequency and di sjoi ntStack Class of Package Java, u t i lClass PriorityQueue and Interface QueueSetsMapsP r o p e r t i e s ClassSynchronized CollectionsUnmodifiable CollectionsAbstract ImplementationsWrap-UpI 7 Java SE 8 Lambdas and Streams17.117.217.317.4IntroductionFunctional Programming Technologies Overview17.2.1 Functional Interfaces17.2.2 Lambda Expressions17.2.3 StreamsIntStream Operations17.3.1 Creating an IntStream and Displaying Its Values with theforEach Terminal Operation17.3.2 Terminal Operations count, min, max, sum and average17.3.3 Terminal Operation reduce17.3.4 Intermediate Operations: Filtering and Sorting IntStream Values17.3.5 Intermediate Operation: Mapping17.3.6 Creating Streams of i n t s with IntStream Methods range andrangeClosedStream Integer Manipulations17.4.1 Creating a Stream Integer 17.4.2 Sorting a Stream and Collecting the Results17.4.3 Filtering a Stream and Storing the Results for Later 57557559560561561562563563
Contents17.4.4 Filtering and Sorting a Stream and Collecting the Results17.4.5 Sorting Previously Collected Results17.5 Stream String Manipulations17.5.1 Mapping S t r i ngs to Uppercase Using a Method Reference17.5.2 Filtering S t r i ngs Then Sorting Them in Case-InsensitiveAscending Order17.5.3 Filtering S t r i ngs Then Sorting Them in Case-InsensitiveDescending Order17.6 St ream Employee Manipulations17.6.1 Creating and Displaying a Li st Emp"loyee 17.6.2 Filtering Empl oyees with Salaries in a Specified Range17.6.3 Sorting Empl oyees By Multiple Fields17.6.4 Mapping Employees to Unique Last Name Strings17.6.5 Grouping Employees By Department17.6.6 Counting the Number of Employees in Each Department17.6.7 Summing and Averaging Employee Salaries17.7 Creating a Stream Stri ng from a File17.8 Generating Streams of Random Values17.9 Lambda Event Handlers17.10 Additional Notes on Java SE 8 Interfaces17.11 Java SE 8 and Functional Programming Resources17.12 Wrap-Up18 Generic Classes and ctionMotivation for Generic MethodsGeneric Methods: Implementation and Compile-Time TranslationAdditional Compile-Time Translation Issues: Methods That Use aType Parameter as the Return TypeOverloading Generic MethodsGeneric ClassesRaw TypesWildcards in Methods That Accept Type ParametersWrap-Up19 Swing GUI Components: Part erUnderstanding Windows in JavaUsing Menus with FramesIPopupMenuPluggable Look-and-FeelJDesktopPane and 90593594601605609611612612616617625628633636
xviContents19.9 BoxLayout Layout Manager19.10 Cn'dBagLayout Layout Manager19.11 Wrap-Up2 0 1020.1120.1220.1320.1420.1520.16IntroductionThread States and Life Cycle20.2.1 New and Runnable States20.2.2 Waiting State20.2.3 Timed Waiting State.20.2.4 Blocked State20.2.5 Terminated State20.2.6 Operating-System View of the Runnable State20.2.7 Thread Priorities and Thread Scheduling20.2.8 Indefinite Postponement and DeadlockCreating and Executing Threads with the Executor FrameworkThread Synchronization20.4.1 Immutable Data20.4.2 Monitors20.4.3 Unsynchronized Mutable Data Sharing20.4.4 Synchronized Mutable Data Sharing—MakingOperations AtomicProducer/Consumer Relationship without SynchronizationProducer/Consumer Relationship: ArrayBlockingQueue(Advanced) Producer/Consumer Relationship with synchronized,wait, n o t i f y and notifyAII(Advanced) Producer/Consumer Relationship: Bounded Buffers(Advanced) Producer/Consumer Relationship: The Lock andCondi t i on InterfacesConcurrent CollectionsMultithreading with GUI: SwingWorker20.11.1 Performing Computations in a Worker Thread: Fibonacci Numbers20.11.2 Processing Intermediate Results: Sieve of Eratostheness o r t / p a r a l l e i Sort Timings with the Java SE 8 Date/Time APIJava SE 8: Sequential vs. Parallel Streams(Advanced) Interfaces C a l l a b l e and Future(Advanced) Fork/Join FrameworkWrap-Up2 1 Accessing Databases with JDBC21.121.221.321.4IntroductionRelational DatabasesA books 1723726730730732733734735739
Contents21.4.1 Basic SELECT Query21.4.2 WHERE Clause21.4.3 ORDER BY Clause21.4.4 Merging Data from Multiple Tables: INNER JOIN21.4.5 INSERT Statement21.4.6 UPDATE Statement21.4.7 DELETE Statement21.5 Setting up a Java DB Database21.5.1 Creating the Chapter's Databases on Windows21.5.2 Creating the Chapter's Databases on Mac OS X21.5.3 Creating the Chapter's Databases on Linux21.6 Manipulating Databases with J D B C21.6.1 Connecting to and Querying a Database21.6.2 Querying the books Database21.7 RowSet Interface21.8 PreparedStatements21.9 Stored Procedures21.10 Transaction Processing21.11 Wrap-Up2 2 X Scene Builder and the NetBeans IDEJavaFX App Window StructureWelcome App—Displaying Text and an Image22.4.1 Creating the App's Project22.4.2 NetBeans Projects Window—Viewing the Project Contents22.4.3 Adding an Image to the Project22.4.4 Opening JavaFX Scene Builder from NetBeans22.4.5 Changing to a VBox Layout Container22.4.6 Configuring the VBox Layout Container22.4.7 Adding and Configuring a Label22.4.8 Adding and Configuring an ImageView22.4.9 Running the Wei come AppTip Calculator App—Introduction to Event Handling22.5.1 Test-Driving the Tip Calculator App22.5.2 Technologies Overview22.5.3 Building the App's GUI22.5.4 TipCalculator Class22.5.5 TipCalculatorController ClassWrap-Up2 3 ATM Case Study, Part 1: Object-OrientedDesign with the UML23.1Case Study 795796796796797798799799802806808813815816
xviii23.223.323.423.523.623.723.8ContentsExamining the Requirements DocumentIdentifying the Classes in a Requirements DocumentIdentifying Class AttributesIdentifying Objects'States and ActivitiesIdentifying Class OperationsIndicating Collaboration Among ObjectsWrap-Up2 4 A T M Case Study Part 2: Implementing anObject-Oriented oductionStarting to Program the Classes of the ATM SystemIncorporating Inheritance and Polymorphism into the ATM SystemATM Case Study 2869874875876877878880883884885889892Class ATMClass ScreenClass KeypadClass CashDispenserClass DepositSlotClass AccountClass BankDatabaseClass TransactionClass BalancelnquiryClass WithdrawalClass DepositClass ATMCaseStudy24.5Wrap-Up893AOperator Precedence Chart895BASCII Character Set897CKeywords and Reserved Words898DPrimitive Types899EUsing the Debugger900E.lE.2E.3IntroductionBreakpoints and the run, stop, cont and print CommandsThe print and set Commands901901905
ContentsE.4E.5E.6E.7Controlling Execution Using the step, step up and next CommandsThe watch CommandThe clear CommandWrap-UpFUsing the Java API DocumentationF.lF.2IntroductionNavigating the Java APIGCreating Documentation with j avadocG.lG.2G.3G.4G.5IntroductionDocumentation CommentsDocumenting Java Source Codej avadocFiles Produced by j avadocHUnicode H.lH.2H.3H.4H.5H.6IntroductionUnicode Transformation FormatsCharacters and GlyphsAdvantages/Disadvantages of UnicodeUsing UnicodeCharacter RangesFormatted .14IntroductionStreamsFormatting Output with printfPrinting IntegersPrinting Floating-Point NumbersPrinting Strings and CharactersPrinting Dates and TimesOther Conversion CharactersPrinting with Field Widths and PrecisionsUsing Flags in the printf Format StringPrinting with Argument IndicesPrinting Literals and Escape SequencesFormatting Output with Class 950952954956960960961962
XXContentsJNumber SystemsJ.lJ.2J.3J.4J. 5J.6IntroductionAbbreviating Binary Numbers as Octal and Hexadecimal NumbersConverting Octal and Hexadecimal Numbers to Binary NumbersConverting from Binary, Octal or Hexadecimal to DecimalConverting from Decimal to Binary, Octal or HexadecimalNegative Binary Numbers: Two's Complement NotationKBit ManipulationK. 1K.2K.3IntroductionBit Manipulation and the Bitwise OperatorsBitSet ClassLLabeled break and c o n t i n u e StatementsL.lL.2L.3IntroductionLabeled break StatementLabeled continue StatementMUML 2: Additional Diagram TypesM.lM.2IntroductionAdditional Diagram 990990990NDesign Patterns992N. 1N.2IntroductionCreational, Structural and Behavioral Design PatternsN.2.1 Creational Design PatternsN.2.2 Structural Design PatternsN.2.3 Behavioral Design PatternsN.2.4 ConclusionDesign Patterns in Packages j ava. awt and j avax. swi ngN.3.1 Creational Design PatternsN.3.2 Structural Design PatternsN.3.3 Behavioral Design PatternsN.3.4 ConclusionConcurrency Design PatternsDesign Patterns Used in Packages j ava. i o and j ava .netN.5.1 Creational Design PatternsN.5.2 Structural Design PatternsN.5.3 Architectural PatternsN.5.4 ConclusionDesign Patterns Used in Package 0610061006100810101010N.3N.4N. 5N.6
ContentsN.7N.6.1 Creational Design PatternsN.6.2 Behavioral Design PatternsWrap-UpIndexxxi1010101010111013
JAVA SE 8 FOR PROGRAMMERS THIRD EDITION DEITEL DEVELOPER SERIES Paul Deitel Harvey Deitel Deitel & Associates, Inc. D'C'TT'n'T Hil 1 XIIJ-J PRENTICE . 2.2 Your First Program in Java: Printing a Line of Text 21 2.3 Modifying Your First Java