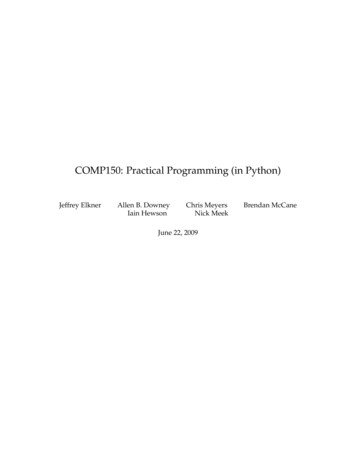
Transcription
COMP150: Practical Programming (in Python)Jeffrey ElknerAllen B. DowneyIain HewsonChris MeyersNick MeekJune 22, 2009Brendan McCane
ii
Contents1The way of the program11.1The Python programming language . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .11.2What is a program? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .31.3What is debugging? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .41.4Experimental debugging . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .41.5Formal and natural languages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .51.6The first program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .61.7Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .61.8The COMP150 lab . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .71.8.1Logging-on . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .81.8.2The OSX desktop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .81.8.3Menus . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .81.8.4The dock . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .81.8.5The Finder window . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .101.8.6Your home directory . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .101.8.7Applications . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .101.8.8IDLE . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .111.8.9Getting help . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .111.8.10 Coursework files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .131.8.11 Terms requirements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .13Blackboard . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .141.10 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .14Variables, expressions and statements192.1Values and types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .192.2Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .202.3Variable names and keywords . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .212.4Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .222.5Evaluating expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .221.92iii
34562.6Operators and operands . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .232.7The modulus operator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .232.8Order of operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .242.9Operations on strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .242.10 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .252.11 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .26Python built-ins (batteries included)293.1Function calls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .293.2Type conversion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .303.3Input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .313.4Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .323.5Examining things . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .333.6More types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .333.7Strings and lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .343.8Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .353.9Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .35Functions: part 1394.1Composing expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .394.2Function definitions and use . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .394.3Flow of execution . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .414.4Parameters and arguments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .424.5Function composition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .434.6Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .434.7Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .44Functions: part 2475.1Variables and parameters are local . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .475.2Stack diagrams . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .485.3Comments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .495.4Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .495.5Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .49Conditionals536.1Boolean values and expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .536.2Logical operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .546.3Conditional execution . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .546.4Alternative execution . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .546.5Chained conditionals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .55iv
7896.6Nested conditionals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .566.7Booleans and type conversion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .576.8Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .576.9Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .58Fruitful functions637.1The return statement . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .637.2Return values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .637.3Program development . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .657.4Composition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .667.5Boolean functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .677.6The function type . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .687.7Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .697.8Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .70Test driven development718.1Modules, files and the import statement . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .718.2Programming with style . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .728.3Triple quoted strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .728.4Unit testing with doctest . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .738.5Test-driven development demonstrated . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .758.6Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .758.7Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .76Files and modules799.1Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .799.2Processing things from a file . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .809.3Directories . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .829.4Modules . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .829.5Creating modules . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .839.6Namespaces . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .849.7Attributes and the dot operator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .849.8Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .849.9Laboratory Test . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .8610 Iteration: part 19110.1 Multiple assignment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9110.2 Updating variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9210.3 The while statement . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9210.4 Tracing a program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .93v
10.5 Counting digits . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9410.6 Abbreviated assignment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9410.7 Tables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9510.8 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9610.9 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9711 Iteration: part 29911.1 Two-dimensional tables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9911.2 Encapsulation and generalization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9911.3 More encapsulation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10011.4 Local variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10111.5 More generalization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10111.6 Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10311.7 Newton’s method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10311.8 Algorithms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10311.9 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10411.10Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10412 In-class test10713 Graphical user interface programming10913.1 Event driven programming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10913.2 TkInter introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10913.3 Introducing callbacks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11213.4 User input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11413.5 Mini-case study . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11513.6 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11613.7 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11714 Case study: Catch11914.1 Graphics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11914.2 Moving the ball . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11914.3 Adding randomness . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12114.4 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12314.5 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12415 Case study: Catch continued12515.1 Keyboard input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12515.2 Checking for collisions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12615.3 Keeping score . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 129vi
15.4 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13115.5 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13215.6 Optional extension project: pong.py . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13216 Strings part 113316.1 A compound data type . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13316.2 Length . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13316.3 Traversal and the for loop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13416.4 String slices . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13516.5 String comparison . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13616.6 Strings are immutable . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13616.7 The in operator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13616.8 A find function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13716.9 Looping and counting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13816.10Optional parameters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13816.11Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13916.12Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14017 Strings part 214317.1 str Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14317.2 Character classification . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14517.3 String formatting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14517.4 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14717.5 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14818 Lists part 115118.1 List values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15118.2 Accessing elements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15218.3 List length . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15318.4 List membership . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15318.5 List operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15418.6 List slices . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15418.7 The range function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15418.8 Lists are mutable . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15518.9 List deletion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15618.10Objects and values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15618.11Aliasing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15718.12Cloning lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15818.13Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 158vii
18.14Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15919 Lists part 216119.1 Lists and for loops . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16119.2 List parameters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16219.3 Pure functions and modifiers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16319.4 Which is better? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16319.5 Nested lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16419.6 Matrices . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16419.7 Strings and lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16519.8 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16519.9 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16620 Tuples16720.1 Tuples and mutability . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16720.2 Tuple assignment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16820.3 Tuples as return values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16920.4 Why tuples? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16920.5 When should you use tuples? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16920.6 Sets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17020.7 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17020.8 Laboratory Test . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17121 Dictionaries17721.1 Dictionary operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17821.2 Dictionary methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17821.3 Aliasing and copying . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17921.4 Sparse matrices . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17921.5 Counting letters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18021.6 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18121.7 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18222 System programming18322.1 The sys module and argv . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18322.2 The os and glob module . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18422.3 A mini-case study . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18622.3.1 ImageMagick . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18622.3.2 Scripting ImageMagick . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18722.4 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19022.5 Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 191viii
23 Classes and objects19323.1 Object-oriented programming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19323.2 User-defined compound types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19323.3 The init Method and self . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19423.4 Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19423.5 Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19523.6 Sameness . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19623.7 Rectangles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19723.8 Instances as return values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19823.9 Objects are mutable . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19923.10Copying . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19923.11Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20023.12Laboratory exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20224 Case study 220524.1 Program Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20524.2 The Initial Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20624.3 Opening a File . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20624.4 Saving to a File . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20824.5 Encrypting the Contents . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20824.6 Putting it All Together . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21124.7 Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21324.8 Laboratory Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21425 The last lecture21525.1 Stuff we haven’t covered . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21525.2 What you can expect from COMP160 and Computer Science . . . . . . . . . . . . . . . . . . . 215Extra Extension Exercises from Part 1217GNU Free Documentation License2211. APPLICABILITY AND DEFINITIONS . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2212. VERBATIM COPYING . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2223. COPYING IN QUANTITY . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2234. MODIFICATIONS . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2235. COMBINING DOCUMENTS . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2246. COLLECTIONS OF DOCUMENTS . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2257. AGGREGATION WITH INDEPENDENT WORKS . . . . . . . . . . . . . . . . . . . . . . . . . . 2258. TRANSLATION . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2259. TERMINATION . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 225ix
10. FUTURE REVISIONS OF THIS LICENSE . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 226ADDENDUM: How to use this License for your documents . . . . . . . . . . . . . . . . . . . . . . 226x
Lecture 1The way of the programThe goal of this paper is twofold: to teach you how to program in Python; and to teach you to think likea computer scientist. It is almost impossible to become a competent programmer without also learninghow to think like a computer scientist. This way of thinking combines some of the best features of mathematics, engineering, and natural science. Like mathematicians, computer scientists use formal languagesto denote ideas (specifically computations). Like engineers, they design things, assembling componentsinto systems and evaluating trade-offs among alternatives. Like scientists, they observe the behaviour ofcomplex systems, form hypotheses, and test predictions.If you aren’t interested in becoming a computer scientist, but just want to gain some programming skills,then this is the perfect paper for you. In this paper we are going to focus on practical programming skillssuitable for solving smallish programming problems. Problems that you will often encounter if you use acomputer regularly.The single most important skill for a computer scientist is problem solving. Problem solving means theability to formulate problems, think creatively about solutions, and express a solution clearly and accurately. As it turns out, the process of learning to program is an excellent opportunity to practice problemsolving skills. That’s why this chapter is called, “The way of the program.”On one level, you will be learning to program, a useful skill by itself. On another level, you will useprogramming as a means to an end. As we go along, that end will become clearer.1.1The Python programming languageThe programming language you will be learning is Python. Python is an example of a high-level language;other high-level languages you might have heard of are C , PHP, and Java.As you might infer from the name high-level language, there are also low-level languages, sometimesreferred to as machine languages or assembly languages. Loosely speaking, computers can only executeprograms written in low-level languages. Thus, programs written in a high-level language have to beprocessed before they can run. This extra processing takes some time, which is a small disadvantage ofhigh-level languages.But the advantages are enormous. First, it is much easier to program in a high-level language. Programswritten in a high-level language take less time to write, they are shorter and easier to read, and they are morelikely to be correct. Second, high-level languages are portable, meaning that they can run on different kindsof computers with few or no modifications. Low-level programs can run on only one kind of computer andhave to be rewritten to run on another.1
Due to these advantages, almost all programs are written in high-level languages. Low-level languages areused only for a few specialized applications.Two kinds of applications process high-level languages into low-level languages: interpreters and compilers. An interpreter reads a high-level program and executes it, meaning that it does what the program says.It processes the program a little at a time, alternately reading lines and performing computations.SOURCECODEOUTPUTINTERPRETERA compiler reads the program and translates it into a low-level program, which can then be run.In this case, the high-level program is called the source code, and the translated program is called theobject code or the executable. Once a program is compiled, you can execute it repeatedly without CUTOROUTPUTMany modern languages use both processes. They are first compiled into a lower level language, calledbyte code, and then interpreted by a program called a virtual machine. Python uses both processes, butbecause of the way programmers interact with it, it is usually considered an interpreted language.There are two ways to use the Python interpreter: shell mode and script mode. In shell mode, you type Pythonstatements into the Python shell and the interpreter immediately prints the result.In this course we will be using an IDE (Integrated Development Environment) called IDLE. When you firststart IDLE it will open an interpreter window.1Python 2.5.1 (r251:54863, Apr 15 2008, 22:57:26)[GCC 4.0.1 (Apple Inc. build 5465)] on darwinType "copyright", "credits" or "license()" for more **************************Personal firewall software may warn about the connection IDLEmakes to its subprocess using this computer’s internal loopbackinterface. This connection is not visible on any externalinterface and no data is sent to or received from the ***********************IDLE 1.2.1 The first few lines identify the version of Python being used as well as a few other messages; you can safelyignore the lines about the firewall. Next there is a line identifying the version of IDLE. The last line startswith
byte code, and then interpreted by a program called a virtual machine. Python uses both processes, but because of the way programmers interact with it, it is usually considered an interpreted language. There are two ways to use the Python interpreter: shell mode and scr