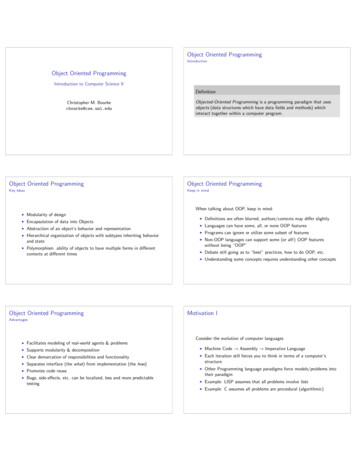
Transcription
Object Oriented ProgrammingIntroductionObject Oriented ProgrammingIntroduction to Computer Science IIDefinitionChristopher M. Bourkecbourke@cse.unl.eduObjected-Oriented Programming is a programming paradigm that usesobjects (data structures which have data fields and methods) whichinteract together within a computer program.Object Oriented ProgrammingObject Oriented ProgrammingKey IdeasKeep in mindWhen talking about OOP, keep in mind:IModularity of designIEncapsulation of data into ObjectsIAbstraction of an object’s behavior and representationIHierarchical organization of objects with subtypes inheriting behaviorand stateIPolymorphism ability of objects to have multiple forms in differentcontexts at different timesObject Oriented ProgrammingIDefinitions are often blurred, authors/contexts may differ slightlyILanguages can have some, all, or none OOP featuresIPrograms can ignore or utilize some subset of featuresINon-OOP languages can support some (or all!) OOP featureswithout being “OOP”IDebate still going as to “best” practices, how to do OOP, etc.IUnderstanding some concepts requires understanding other conceptsMotivation IAdvantagesConsider the evolution of computer languagesIFacilitates modeling of real-world agents & problemsISupports modularity & decompositionIIClear demarcation of responsibilities and functionalityIISeparates interface (the what) from implementation (the how)IPromotes code reuseIBugs, side-effects, etc. can be localized, less and more predictabletestingMachine Code Assembly Imperative LanguageEach iteration still forces you to think in terms of a computer’sstructureIOther Programming language paradigms force models/problems intotheir paradigmIExample: LISP assumes that all problems involve listsIExample: C assumes all problems are procedural (algorithmic)
Motivation IIIIn general, problems are forced into a language’s paradigmIInvolves some “translation” or pigeonholingIGood for domain-specific problems or just number-crunching, bad forgeneralityIOpposite of what it should be: computers should be serving our needsILanguages should model our world and problemsVersus DeclarativeDeclarative ParadigmI“Declares” behavior of a programISpecifies logic of computation, not control flowIFunctional, avoids state (Lisp, Scheme, Haskell)ILogic programmingIDomain languages: Regular Expressions, HTML, Excel, SQLINot necessarily Turing-completeHistory of OOP IIParadigms have “evolved” to OOPIMIT 50s - 60s: LISP “atoms”I1960: Sketchpad (first CAD, revolutionary GUI) by Ivan Sutherland,introduced initial conceptsI1967: Simula 67 by Dahl & Nygaard (bank simulation; agents:tellers, customers, accounts, transactions; physical modeling)1972: Smalltalk by Alan KayIIIIIIBased on Plato’s Theory of FormsAllegory of the Cave“A form is an objective ’blueprint’ of perfection” (an archetype)No perfect circle, just shadows, representations of it verything has aquintessence, an archetype, an apotheosis1983: Bjarne Stoustrup develops C IInspired to add OOP features to C from SimulaContrasting with Other ParadigmsIMost modern programming languages support some OOP featuresIFeatures back-added due to demand or advantages that OOP providesIOOP vs. Declarative paradigmIOOP vs. Imperative paradigmVersus ImperativeImperative ParadigmISpecifies a specific process (algorithm) that changes a program’s stateIBuilt from one or more procedures (methods, functions)IProcedural & Structured paradigms (state changes are local orparameterized), not encapsulatedIExamples: FORTRAN, CHistory of OOP IIIFirst compilers simply translated C syntax to pure CI1983: Brad Cox & Tom Love develop Objective-CI1990s: OOP came to dominate programming methodologies(especially due to GUI development)ILanguages updated to include OOP features1995: Java!IIIIIIIIInspired to add OOP features to C from Smalltalk1995 by James Gosling, Sun Microsystems“simple, object-oriented and familiar”“robust & secure”“architecture-neutral and portable”“high performance”“interpreted, threaded, dynamic”
Continued Refinements IIDesign Patterns - a general reusable solution to a common andreoccurring problem in software design (also called idioms)IAnti-patterns and “smells”IModeling tools (UML – Unified Modeling Language)IDesign-by-contract frameworksIIntegrated Development Environments (IDEs)IDevelopment of libraries, frameworksIDevelopment of methodologies, best practices, etc.ObjectsContinued Refinements IIFigure : UML Diagram ExampleObjectsAbstract Data TypesIBasis for Objects: Abstract Data Types (ADTs)DefinitionIMathematical model for classes of data structures with similarbehavior or semanticsAn object is a general entity characterized by the following.IPurely theoretical entitiesIIdentity – An aspect that distinguishes it from other objectsIGeneralization applicable to areas other than OOPIState – properties (attributes, elements, data, fields)IAlgebraic structures, AI, algorithms, etc.IIExamples: stacks, queues, maps, strings, and their operationsBehavior – describes an object’s interface (methods, routines,functions)Objects IObjects IIKey ConceptsKey ConceptsMessage sendingIObjects provide servicesIClient code (user) can access these services by sending messagesIExample: a signal to execute one of its methodsIA signal to turn a Light object on() or off()IFacilitated by an externally available interfaceComplex Data TypesICollection of multiple pieces of informationIMixed typesIVarying visibility
Objects IIIObjects IVKey ConceptsKey ConceptsConstructionIObjects are constructed rather than assignedIConstruction may be specified through constructorsIAssignment through referencesIContrast with primitive typesObjects VObject DesignIGeneral approach: decompose an entity to the point where it issimple enough to implement or a suitable object already existsIKeep it simpleISemantics may dictate designIAvoid the God-Object anti-pattern4 Main PrinciplesKey ConceptsExamplesIAn animal is a general typeIDogs, Cats, Snakes, Lizards are all animalsIHow can we further develop a taxonomy?Should the following be incorporated?IIIPets?Gender?IStrict is-a relationshipIExamples: vehicle, automobile, truck, car, boat planeAbstraction IDefinitionAbstraction is the mechanism by which objects are given theirrepresentation and implementation details are hidden.Abstraction is apparent in many areas:IApplication layers (database, middle-tier, front-end)ISystem LayersIProtocol layers (OSI networking model)Object Oriented Programming involves 4 main principles1. Abstraction2. Encapsulation3. Inheritance4. PolymorphismAbstraction IIAlready familiar with Procedural Abstraction: the implementation of aprocess is codified into a reusable piece of code inside a function.Example: a square root function may be implemented using variousalgorithms.ITaylor seriesIBabylonian methodISome other interpolation method
Abstraction IIIAbstraction IVIAbstraction in OOP takes this further: methods implementations areabstracted, but so are the internal representations of the objectsIObjects are defined with a representation similar to is meaning(semantics) while hiding implementation detailsIDefines the conceptual boundaries of an object, distinguishingbetween objectsIn the end: who cares?IProcedural abstraction relieves us of the need to worry aboutimplementation detailsAllows the client (programmer, user) to deal with objects based ontheir public interface without worrying about detailsIIt just works!Example: A sorting functionISelection sort? Quick sort? Other?ITemporary space?IHow does it swap?Abstraction in languagesAbstraction Example 1Date/TimeA date/time stamp object may be defined to represent a particular pointin time (with functionality for formatting, comparing and operating withdurations, intervals, other date/time instances).IAbstraction of objects is achieved through its publicly availableinterfaceIInterface specifies how you can use the objectIA collection of individual numbers (year, month, day, etc.)A single numerical value (time from a particular epoch)ISO 8601-formatted string (ex:2012-05-04T12:20:34.000343 01:00)How could such an object be represented?Specifies which methods may be signaled by client codeIIMay specify a contractIIIn conjunction with inheritance: behavior of subtypes may change, beenhanced, etc.IAbstraction Example 2Abstraction relieves us of the need to worry about such detailsIRepresentation is internal to the objectIConsistency and changes are handled by the objectIWe only interact with its interfaceExample 3StringsMost languages support strings, but may use different representationsIA null-terminated character arrayIA length-prefixed character arrayIDynamic character arrayIASCII or Unicode?Representing a string as an object relieves us of the need to worry aboutthese detailsIContrast with procedural language (C): memory management,consistency, expectations must be handled manuallyIInstead of worrying about details: just use it!A Graph has vertices (nodes) and edges; may be represented as:ImatrixIadjacency listImaps
Encapsulation IEncapsulation IIDefinitionEncapsulation is a mechanism for restricting access to (some of) anobject’s components and for bundling data and methods operating on thatdata.Includes:IA form of information hidingIIn contrast to abstraction which is implementation hidingIIncludes the aspect of grouping data – modularityIProtection of dataIAllows restriction to access and control of dataIAllows internal behavior to change, evolve, be fixed without affectingclient codeIUsually breakable (through reflection, exposing in subclasses)1. Grouping of data2. Protection of data3. Grouping of methods that act on an object’s dataEncapsulation IIIEncapsulationBest PracticeIEnables mutator and accessor methodsIIIChanges are made to be intentionalSide-effects can be controlled and localizedAllows validationIBest practice: make everything private, control access throughgetters/settersIObjects should not just be data containersIEnables objects to be immutable (predictable, thread-safe)IIKey idea: abstraction allows a user to access essential information,encapsulation hides the data, they compliment each otherAny method that acts on an object’s data should be a membermethodIDo not break encapsulation by placing related functionality elsewhereEncapsulation I 1 public class BankAccount {2private double balance ;3private double apr ;4.5// constructors , getters / setters6.7public double g et Mo nt hl yE ar ni ng s () {8return this . balance * ( this . apr / 12.0) ;9}10 } Java Example1. Grouping of data2. Protection of data3. Grouping of functionality that acts on that data Encapsulation IIJava Example public class BankUtils {Breaking encapsulation:1234 56} public static double g et Mo nt hl yE ar ni ng s (BankAccount acct ) {return acct . getBalance () * ( acct . getAPR () /12.0) ;}
Side-effectsWhy EncapsulationDefinitionBeginners question: why can’t everything just be public?A function or expression has a side-effect if it modifies some state or hasan observable interaction with calling functions or other parts of theprogram (either globally, to other threads, the outside world, etc.).Without Encapsulation:Examples:IInternal state could be modified with impunityIEverything essentially becomes globalIDifficult to test/controlIA sort method that reorders the original listIAnti-modularIA setter method that modifies an object’s state that can be observedthrough its public interfaceINo way to enforce rulesIA subroutine used in a logical expression that makes changes to itsparametersIAny changes to global, static variables, parameters, data I/O, etc.InheritanceSave on typing: utilize IDE macros (Eclipse: Source generategetters/setters)Aspects of InheritanceDefinitionInheritance is the ability to extend the definition of objects; creatingobjects from previously created or defined objectsMost common form: classical inheritance (subclassing)IAllows us to define a hierarchy of related objectsISubclasses inherit behavior and/or fields from superclassesISubclasses can augment, modify, or change the behavior of methodsIFacilitates code reuseIGeneralized common behavior and/or interface can be defined insuperclassesIsubclass/superclassISubclasses provide specialization (more specificity)Iderived/base classesIIchild/parent classesGeneral properties or behavior is specialized into more concreteimplementationsISuper/subclass defines an is-a relationshipInheritanceJava ExampleJava ExampleIBird is a superclassIAll birds move(), but how?IOstrich and Robin may be subclassesIOstrich may specify a walk() methodIRobin may specify a fly() methodImove() may be overridden in the subclasses to call their respectivemethodsISubclasses may be referred to as their super class123456789101112131415161718192021222324252627 public class Bird {public void move () {System . out . println ( " Moving somehow . " ) ;}} public class Robin extends Bird {public void fly () {System . out . println ( " Flying . " ) ;}.@Overridepublic void move () {this . fly () ;}}public class Ostrich extends Bird {public void walk () {System . out . println ( " Walking . " ) ;}.@Overridepublic void move () {this . walk () ;}}
Collections Hierarchy ICollections Hierarchy stIA general collection is a data structure that holds stuffIA set is a collection that holds stuff in an unordered manner; a list isa collection that holds stuff in an ordered mannerIA HashSet is a set that holds stuff in an unordered manner using ahash tableIAn ArrayList is a list that holds stuff in an ordered manner using anarray, etc.Figure : Java Collections Library Inheritance structureVirtual FunctionsHow this works: Dynamic DispatchA virtual function is a function that can be overridden in a subclass.IIC : all functions are non-virtual by default; can be made virtual byuse of the keyword virtualJava: all non-private functions are virtual by default; can be madenon-virtual by use of the keyword finalIIIstatic methods can be overridden1visibility of methods can be more open, but not decreasedwithin a class, access to the superclass’s method is accessible via thesuper keywordIUpon instantiation, a particular constructor is calledIThis creates the object in memory as a virtual table (vtable)IObject has data in memory, but also references to the code for itsmethodsIWhen a method is called, the method which the vtable refers to isinvokedIThis process is a run-time mechanism (since methods can be invokedvia a reference to the super class)1but when static methods are invoked, they should be done so via the class nameanywayHow this works: Dynamic DispatchMultiple InheritanceJava Example12 345678910 Bird b new Bird () ;b . move () ; // prints " Moving somehow ."Robin r new Robin () ;r . move () ; // prints " Flying ."r . fly () ; // prints " Flying ."b r ; // subclass referred to as its superclassb . move () ; // prints " Flying ."// b . fly () ; -- compile errorb new Ostrich () ;b . move () ; // prints " Walking ." IAn object can inherit from multiple parents, called multipleinheritanceIExample: Musician (inherits from Person, Performer)ISupported by some languagesIProblematic to implement properly: the diamond problemIExample: Animal, Dog, Cat, “DogCat”?INecessary to establish a mechanism to disambiguate properties
Why InheritanceLiskov Substitution PrincipleThe Liskov Substitution Principle states: if S is a (subtype of) T , thenany instance of T should be replaceable by an instance of S withoutaltering any of the desired properties of the program.IMotivation: Classification & TaxonomyIUbiquitous: sheer volume of data requires classification to organize itIHierarchical structure is most natural (though not always applicable)IA principle, not a lemmaIFundamental to communicationII.e. should be the caseICommunication requires a common classification/descriptionINot guaranteedICertainly possible to violate (breakable)Beware of PitfallsPolymorphismDefinitionIGood practice: avoid the yo-yo anti-pattern (keep relations shallow)IWhen in doubt: use composition instead!Avoid the rectangle problem (or the circle problem):IIIIObject types should not be mutable to sub/super typesThe is-a relationship (should be) an invariantCode Check: take a look at unl.cse.rectangle problemSubtype PolymorphismIThe “default” type of polymorphismIYou can refer to subclass instances as their superclassInstance’s methods are called dynamically using late-bindingIIIIIIBecause subtypes can provide their own method implementation(overrides),There is not enough information at compile time to determine whichmethod to callExample: Bird may a move() method; while an Ostrich has a run()method, Robin has a fly() methodCode Check: let’s take a look at unl.cse.oop.inheritanceDynamic Dispatch: compiler is able to create a virtual method table(v-table) with references to the correct method to invokePolymorphism is the ability to create a variable, method, or an object thathas more than one form (type).IDerived from Greek: “having multiple forms”IInspired by biology (organisms may have different phenotypes:observable traits; genetic, behavioral, or consequence there of)IAllows more abstract, reusable codeBehavioral SubtypingBehavioral subtyping is a strict type of polymorphism where:ILiskov Substitution Principle is strictly enforcedIMuch stronger form of polymorphismIPre- and Post- conditions are defined & enforced (design-by-contract)IPreconditions cannot be strengthened in subtypesIPostconditions cannot be weakened in subtypesIInvariants are guaranteedISubtypes must be pure inheritance (no new methods)INot generally used in practice
Method OverridingAd-hoc Polymorphism IISubclass augments, modifies, or provides a completely differentimplementation of a methodIMethod has the same name and signatureIMay support covariant return typesIAd-hoc: done as needed for a particular purposeINot the same as (operator) overloading (which is done at compiletime)IOn-the-fly polymorphismMethod Overloading IAd-hoc Polymorphism covers a wide variety of as-needed polymorphicbehavior.Method Overloading IISome examples in practice:Method Overloading: several different “versions” of a method may bedefinedIMethods have the same identifier (name)IIn general, have the same return typeIHowever, must differ in the type or arity (number of) parametersIResolution of which method is called is inferred at compile time basedon the arguments at invocationIMay be considered static dispatchOperator Overloading IIThe same name can be used for several versions of an absolute valuefunction (rather than different names for each numeric type)IC: standard math library has three functions with different names(labs, fabs, abs) for three numeric types; Java: 4 methods withthe same name, different argumentsIJava: String class has 9 valueOf(.) methods to give the Stringvalue of every typeIJava: Integer class has 3 different toString(.) methods (built-in,conversion, base-conversion)Operator Overloading IIShould be avoided:Operator Overloading: built-in operators’ ( , , etc.) behavior can beredefined depending on its operandsISome overloading is already built into languages:IIIIString String may be concatenationNumber Number may be additionNumber String may be conversion-addition orconversion-concatenationIOf limited use (operator-operand combinations can only have onemeaning)IAll combinations of operands that are used must be definedICoding requires defining a function anyway, why not just use thefunction?IBuilt-in operators have a clear and understood meaning; when appliedto user defined types or completely redefined, meaning becomesambiguous, unclear, open to interpretationExample: what does Array Array mean?Some languages allow you to overload an operatorIICould define to mean union when used with arrays or graphsCould define to mean time-addition when used withdate-time/interval typesIIIIConcatenation?Vector addition?Set union?
Type Coercion IIWhen operators are overloaded, rules must be defined for how typesand behaviors are resolved.ITypes are coerced into other types with specific rules so that theoperands become compatibleIType Coercion: one type is mapped to another compatible typeIType Promotion: mapping a type to a super-type for compatibilityIRules may be defined by the language or incompatible types may bedisallowed entirelyISuch type casting may require an explicit instruction by the userType Coercion IIIType Coercion IIExamples:ICasting numbers to strings in order to concatenateIIntegers are cast to floats in order to add/subtract/multiplyIFloats may be down-casted to integers when the assignment operatoris usedType Coercion IVBirdCovariantContravariantGeneral types of conversions:ICovariant: converting from a specialized type to a generalized type(subclass to superclass, Ostrich to Bird)IContravariant: converting from a generalized type to a specializedtype (super to subclass, Bird to Ostrich)IInvariant: no conversion is possibleRobinInvariantOstrichFigure : Covariance, Contravariance, InvarianceIn general, only covariance is “safe” since subtypes define an is-arelationship.More formally, if A B, then a transformation f is:Type Coercion VParametric Polymorphism IParametric polymorphism allow us to define methods or classes that havestrong static type-safety, but that can be applied to many different types.IIIcovariant if f (A) f (B)contravariant if f (B) f (A)invariant otherwiseAllows us to write generic code that depends only on some aspect(s) of alltypes that are applicable to the class/method without depending on theactual type.A method or class is given a generic type, a parameter (parameterized)The specific type is specified by the client code or deduced at runtimeThe parameterized code itself is generic enough that it applies to all types(does not use any type-specific functionality)
Parametric Polymorphism IIBounded Parametric PolymorphismExamples:IA generic “container” class (list or set) can be parameterized to holda specific type (defined at instantiation)IA generic print method that prints elements in an array or collectionin a tidy formatIA generic getMax() method can be used for all numeric typesIA single, generic sorting method can be defined and used for any typethat is “comparable” in some mannerIExample: a parameterized summation method would need to boundthe types to numerical typesOpen RecursionISome information may be needed by the implementationICode needs to know that such objects are numeric types or are“comparable”IBounding the parameterized type guarantees a minimum set ofinformation implied by the typeIBounding the types provides a minimal guarantee of the interfacethey provide (which can then be used!)Open RecursionJava keywordsIObjects are defined, but instances are constructedIAn instance may need to refer to its own methods or fieldsINo variable reference at compile timeISpecial keyword(s) defined (this or self2 )IBound to a specific object (instance) via late-binding2Ithis can be used inside an object to refer to itselfIsuper can be used to access methods/variables of a super classIthis(.) can be used to call an object’s constructorsIsuper(.) can be used to call an object’s superclass’s constructorObjective-C, Python, Ruby; VB uses Me!Association, Aggregation, Composition IAssociation, Aggregation, Composition IIAggregation: “the” relation between two classesAssociation: “a” relation between two classesIAn object knows about another objectICan call its methods, interact with itIMost general object–object relationshipIOne class “has” another (or usually a collection of another) classIUsually by referenceIRelationship does not imply ownershipIOther instances may also “have” the objectIDestruction issuesIExample: University – Departments – Faculty
Association, Aggregation, Composition IIIAssociation, Aggregation, Composition IVComposition vs InheritanceComposition: One object owns anotherIUpon destruction of owner, owned object(s) are also destroyedIShould be private: no one else should depend on themComposition in Java:IIILess important in Java as garbage collection is automaticIf multiple owners, owned objects won’t get destroyedIInheritance is good when hierarchy is rigid and well-established;design is matureIInheritance is fragile as changes up-stream (super classes) will affecteverything downstreamIComposition is more extensible; does not lock you into a hierarchythat would require refactoringIComposition is more flexible, easier to change and changes have lessof an effectIUse composition when in doubtOther IssuesOther IssuesMixinsDuck TypingA mixin is an object that provides functionality to be inherited or reusedIObject’s functionality is mixed in to another objectIMixin itself is abstract and cannot be instantiatedINot a form of specializationIA means to collect functionality into an objectJava: not directly supportedIIIIIOOP languages without classes use dynamic typing (JavaScript,Python)IMethods and properties define a type rather than semanticsIIf it walks like a duck (waddle()), talks like a duck (quack()) then itsa DuckInterfaces provide specification, but not behaviorAbstract classes can provide functionality, but must be inherited andcannot be multiply inheritedPreferred solution: compositionOther IssuesSOLID ICopy ConstructorsSOLID Principles (Features, Martin):IUsual to implement a copy constructor: a constructor that takes aninstance of its own classSRP: Single Responsibility Principle – a class should have only asingle responsibility (i.e. only one potential change in the software’sspecification should be able to affect the specification of the class)IIPitfall: the copy should be a deep copy and not just a copy ofreferencesOCP: Open/Closed Principle – software entities should be open forextension, but closed for modificationIIIf not a deep copy, changes to the “shared” resources will be realizedby both copiesLSP: Liskov Substitution Principle – objects in a program should bereplaceable with instances of their subtypes without altering thecorrectness of that programIISP: Interface Segregation Principle – many client-specific interfacesare better than one general-purpose interfaceIDIP: Dependency Inversion Principle – one should ?Depend uponAbstractions. Do not depend upon concretionsIIts often convenient to have a way to create different copies of objectsI
Summary Example ISummary Example IIMaps 1 Map String , Student m new HashMap String ,Student () ;2 .3 String nuid " 12345678 " ;4 Student s new Student (.) ;5 m . put ( nuid , s ) ;6 .7 String someNuid " 87654321 " ;8 Student joe m . get ( someNuid ) ; IMaps are key-value data structuresIAbstraction: get and put methods define how to use this datastructure without worrying about its implementation detailsSummary Example III MapsIEncapsulation: maps could be implemented using a tree or hashtable;could use open or closed addressing; representation details areirrelevant and hiddent to usIInheritance: HashMap is-a Map that uses a hash table (while a TreeMapis a map that uses a tree)IPolymorphism: through parametric polymorphism, we can mapspecific types to other specific typesIJava note: a Map is not Collection (see /collections/designfaq.html#14Software Engineering & Design Books re : Map data structures in Java Collections librarySoftware Engineering & Design Books IIFigure : Code Complete (1993) by Steve McConnellFigure : Design Patterns: Elements of Reusable Object-Oriented Software (1994)by Gamma et al.Software Engineering & Design Books IIIFigure : The Mythical Man-Month (1975) by Frederick Brooks Jr.
Software Engineering & Design Books IVFigure : Refactoring: Improving the Design of Existing Code (1999) by MartinFowler
Object Design I General approach: decompose an entity to the point where it is simple enough to implement or a suitable object already exists I Keep it simple I Semantics may dictate design I Avoid the God-Object anti-pattern ObjectsV Key Concepts Examples I An animal is a g