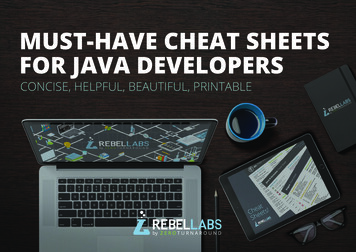
Transcription
MUST-HAVE CHEAT SHEETSFOR JAVA DEVELOPERSCONCISE, HELPFUL, BEAUTIFUL, PRINTABLEeat ts!hC eeShbyby Z E R O T U R N A R O U N DZEROTURNAROUND
Java 8 Streams Cheat SheetDefinitionsA stream is a pipeline of functionsthat can be evaluated.Streams can transform data.A stream is not a data structure.Streams cannot mutate data.Stream examplesParallel streamsGet the unique surnames in uppercase of the first 15 bookauthors that are 50 years old or over.library.stream().map(book - book.getAuthor()).filter(author - author.getAge() ap(String::toUpperCase).collect(toList());Parallel streams use the common ForkJoinPool for threading.library.parallelStream().Compute the sum of ages of all female authors younger than 25.Intermediate operations. Always return streams. Lazily executed.Common examples or).filter(a - a.getGender() Gender.FEMALE).map(Author::getAge).filter(age - age 25).reduce(0, Integer::sum):Terminal operations. Return concrete types or produce a side effect. Eagerly executed.Common examples include:FunctionOutputWhen to usereduceconcrete typeto cumulate elementssortedcollectlist, map or setto group elementspeekforEachside effectto perform a side effecton elementsdistinctor intermediate operation:IntStream.range(1, 10).parallel().Useful ngBy(Book::getGenre));Stream ranges:IntStream.range(0, 20).Infinite streams:IntStream.iterate(0, e - e 1).Max/Min:IntStream.range(1, 10).max();FlatMap:twitterList.stream().map(member - member.getFollowers()).flatMap(followers - ��t update shared mutablevariables i.e.List Book myList new ArrayList ();library.stream().forEach(e - myList.add(e));Avoid blocking operationswhen using parallel streams.BROUGHT TO YOU BY
Java Collections Cheat SheetWhat can your collection do for you?Notable Java collectionslibrariesYour dataCollection classFastutilhttp://fastutil.di.unimi.it/Fast & compact type-specific collections for JavaGreat default choice for collections of primitivetypes, like int or long. Also handles bigcollections with more than 231 elements well.Guavahttps://github.com/google/guavaGoogle Core Libraries for Java 6 Perhaps the default collection library for Javaprojects. Contains a magnitude of convenientmethods for creating collection, like fluentbuilders, as well as advanced collection types.Eclipse tures you want with the collections you needThread-safe alternativeHashMapConcurrentHashMapHashBiMap (Guava)Maps.synchronizedBiMap(new zedMultiMap(new ronizedMap(new omMap(new ConcurrentHashMap ())Java Concurrency Tools for the JVM.If you work on high throughput concurrentapplications and need a way to increase yourperformance, check out JCTools.PrimitivesupportOrder of FORandom accessBy keyBy valueBy index*IntArrayList equeArrayBlockingQueue***** O(log(n)) complexity, while all others are O(1) - constant time**** when using Queue interface methods: offer() / poll()How fast are your collections?need: primitive type collections, ateelementsupportInt2IntMap (Fastutil)includes almost any collection you mightJCToolsKey-valuepairs*Previously known as gs-collections, this librarybidirectional maps and so on.IndividualelementsOperations on your collectionsCollection classRandom accessby index / keySearch (log(n))Remember, not all operations are equally fast. Here’s a reminderof how to treat the Big-O complexity notation:O(1) - constant time, really fast, doesn’t depend on thesize of your collectionO(log(n)) - pretty fast, your collection size has to beextreme to notice a performance impactO(n) - linear to your collection size: the larger yourcollection is, the slower your operations will beBROUGHT TO YOU BY
Git Cheat SheetCreate a RepositoryWorking with BranchesMake a changeSynchronizeFrom scratch -- Create a new localrepository git init [project name]List all local branches git branchStages the file, ready for commit git add [file]List all branches, local and remote git branch -avStage all changed files, ready for commit git add .Get the latest changes from origin(no merge) git fetchSwitch to a branch, my branch,and update working directory git checkout my branchCommit all staged files to versioned history git commit -m “commit message”Download from an existing repository git clone my urlObserve your RepositoryCreate a new branch called new branch git branch new branchCommit all your tracked files toversioned history git commit -am “commit message”Show the changes to files not yet staged git diffDelete the branch called my branch git branch -d my branchUnstages file, keeping the file changes git reset [file]Show the changes to staged files git diff --cachedMerge branch a into branch b git checkout branch b git merge branch aRevert everything to the last commit git reset --hardList new or modified files not yetcommitted git statusShow all staged and unstagedfile changes git diff HEADShow the changes between twocommit ids git diff commit1 commit2Tag the current commit git tag my tagFetch the latest changes from originand merge git pullFetch the latest changes from originand rebase git pull --rebasePush local changes to the origin git pushFinally!When in doubt, use git help git command --helpOr visit https://training.github.com/for official GitHub training.List the change dates and authorsfor a file git blame [file]Show the file changes for a commitid and/or file git show [commit]:[file]Show full change history git logShow change history for file/directoryincluding diffs git log -p [file/directory]BROUGHT TO YOU BY
cheat sheetGetting started with MavenUseful command line optionsEssential pluginsCreate Java projectmvn archetype:generate-DgroupId org.yourcompany.project-DartifactId application-DskipTests true compiles the tests, but skipsrunning themCreate web projectmvn archetype:generate-DgroupId org.yourcompany.project-DartifactId application-DarchetypeArtifactId maven-archetype-webapp-T - number of threads:-T 4 is a decent default-T 2C - 2 threads per CPUHelp plugin — used to get relative information about aproject or the system.mvn help:describe describes the attributes of a pluginmvn help:effective-pom displays the effective POMas an XML for the current build, with the active profilesfactored in.Create archetype from existing projectmvn archetype:create-from-project-pl, --projects makes Maven build only specifiedmodules and not the whole project-Dmaven.test.skip true skips compiling the testsand does not run them-rf, --resume-from resume build from thespecified project-am, --also-make makes Maven figure out whatmodules out target depends on and build them tooMain phasesclean — delete target directoryvalidate — validate, if the project is correctcompile — compile source code, classes storedin target/classestest — run testspackage — take the compiled code and package it in itsdistributable format, e.g. JAR, WARverify — run any checks to verify the package is validand meets quality criteriainstall — install the package into the local repositorydeploy — copies the final package to the remote repositoryPluginsCentralDependency plugin — provides the capability to-o, --offline work offline-X, --debug enable debug output-P, --activate-profiles comma-delimited listof profiles to activate-U, --update-snapshots forces a check for updateddependencies on remote repositories-ff, --fail-fast stop at first failurecompilemaven-compiler-pluginCompiler plugin — compiles your java code.Set language level with the following configuration: plugin groupId org.apache.maven.plugins /groupId artifactId maven-compiler-plugin /artifactId version 3.6.1 /version configuration source 1.8 /source target 1.8 /target /configuration /plugin Version plugin — used when you want to managethe versions of artifacts in a project's POM.compiler:compileDependenciestestWrapper plugin — an easy way to ensurea user of your Maven build has everythingthat is necessary.Spring Boot plugin — compiles yourLocal Repository /.m2/settings.xmlmanipulate artifacts.mvn dependency:analyze analyzes the dependenciesof this projectmvn dependency:tree prints a tree of dependenciespackagemaven-jar-pluginjar:jarSpring Boot app, build an executable fat jar.Exec — amazing general purpose plugin,BROUGHT TO YOU BY
SQL cheat sheetBasic Queries-- filter your columnsSELECT col1, col2, col3, . FROM table1-- filter the rowsWHERE col4 1 AND col5 2-- aggregate the dataGROUP by -- limit aggregated dataHAVING count(*) 1-- order of the resultsORDER BY col2Useful keywords for SELECTS:DISTINCT - return unique resultsBETWEEN a AND b - limit the range, the values can benumbers, text, or datesLIKE - pattern search within the column textIN (a, b, c) - check if the value is contained among given.Data Modification-- update specific data with the WHERE clauseUPDATE table1 SET col1 1 WHERE col2 2-- insert values manuallyINSERT INTO table1 (ID, FIRST NAME, LAST NAME)VALUES (1, ‘Rebel’, ‘Labs’);-- or by using the results of a queryINSERT INTO table1 (ID, FIRST NAME, LAST NAME)SELECT id, last name, first name FROM table2ViewsA VIEW is a virtual table, which is a result of a query.They can be used to create virtual tables of complex queries.CREATE VIEW view1 ASSELECT col1, col2FROM table1WHERE The Joy of JOINsABLEFT OUTER JOIN - all rows from table A,even if they do not exist in table BAINNER JOIN - fetch the results thatexist in both tablesBRIGHT OUTER JOIN - all rows from table B,even if they do not exist in table AUpdates on JOINed QueriesUseful Utility FunctionsYou can use JOINs in your UPDATEsUPDATE t1 SET a 1FROM table1 t1 JOIN table2 t2 ON t1.id t2.t1 idWHERE t1.col1 0 AND t2.col2 IS NULL;You can use subqueries instead of JOINs:-- convert strings to dates:TO DATE (Oracle, PostgreSQL), STR TO DATE (MySQL)-- return the first non-NULL argument:COALESCE (col1, col2, “default value”)-- return current time:CURRENT TIMESTAMP-- compute set operations on two result setsSELECT col1, col2 FROM table1UNION / EXCEPT / INTERSECTSELECT col3, col4 FROM table2;SELECT col1, col2 FROM table1 WHERE id IN(SELECT t1 id FROM table2 WHERE date CURRENT TIMESTAMP)Union Except -NB! Use database specific syntax, it might be faster!Semi JOINsIndexesIf you query by a column, index it!CREATE INDEX index1 ON table1 (col1)Don’t forget:Avoid overlapping indexesAvoid indexing on too many columnsIndexes can speed up DELETE and UPDATE operationsreturns data from both queriesrows from the first query that are not presentin the second queryIntersect - rows that are returned from both queriesReportingUse aggregation functionsCOUNT - return the number of rowsSUM - cumulate the valuesAVG - return the average for the groupMIN / MAX - smallest / largest valueBROUGHT TO YOU BY
Regex cheat sheetCharacter classesUseful Java classes & methods[abc]matches a or b, or c.[ abc] negation, matches everything except a, b, or c.[a-c]range, matches a or b, or c.[a-c[f-h]]union, matches a, b, c, f, g, h.[a-c&&[b-c]] intersection, matches b or c.[a-c&&[ b-c]] subtraction, matches a.PATTERNPredefined character classes.\d\D\s\S\w\WAny character.A digit: [0-9]A non-digit: [ 0-9]A whitespace character: [ \t\n\x0B\f\r]A non-whitespace character: [ \s]A word character: [a-zA-Z 0-9]A non-word character: [ \w]Boundary matches \b\B\A\G\Z\zThe beginning of a line.The end of a line.A word boundary.A non-word boundary.The beginning of the input.The end of the previous match.The end of the input but for the final terminator, if any.The end of the input.Pattern flagsPattern.CASE INSENSITIVE - enables case-insensitivematching.Pattern.COMMENTS - whitespace and comments startingwith # are ignored until the end of a line.Pattern.MULTILINE - one expression can matchmultiple lines.Pattern.UNIX LINES - only the '\n' line terminatoris recognized in the behavior of ., , and .A pattern is a compiler representation of a regular expression.QuantifiersGreedyPattern compile(String regex)Compiles the given regular expression into a pattern.String[] split(CharSequence input)Splits the given input sequence around matches ofthis pattern.String quote(String s)Returns a literal pattern String for the specified String.Predicate String asPredicate()Creates a predicate which can be used to match a string.MATCHERAn engine that performs match operations on a charactersequence by interpreting a Pattern.boolean matches()Attempts to match the entire region against the pattern.boolean find()Attempts to find the next subsequence of the inputsequence that matches the pattern.int start()Returns the start index of the previous match.int end()Returns the offset after the last character matched.DescriptionX?X?X? X, once or not at all.X*X*?X* X, zero or more times.X X ?X X, one or more times.X{n}X{n}?X{n} X, exactly n times.X{n,}X{n,}?X{n,} X, at least n times.X{n,m}X{n,m}?X{n,m} X, at least n butnot more than m times.Pattern compile(String regex, int flags)Compiles the given regular expression into a patternwith the given flags.boolean matches(String regex)Tells whether or not this string matches the givenregular expression.Reluctant PossessiveGreedy - matches the longest matching group.Reluctant - matches the shortest group.Possessive - longest match or bust (no backoff).Groups & backreferencesA group is a captured subsequence of characters which maybe used later in the expression with a backreference.(.) - defines a group.\N - refers to a matched group.(\d\d) - a group of two digits.(\d\d)/\1- two digits repeated twice.\1 - refers to the matched group.Logical operationsXYX YX then Y.X or Y.BROUGHT TO YOU BY
mvn help:effective-pom displays the effective POM as an XML for the current build, with the active profiles factored in. — provides the capability to manipulate artifacts. mvn dependency:analyze analyzes the dependencies of this project mvn dependency:tree prints a tree of dependencies Compiler plugin —