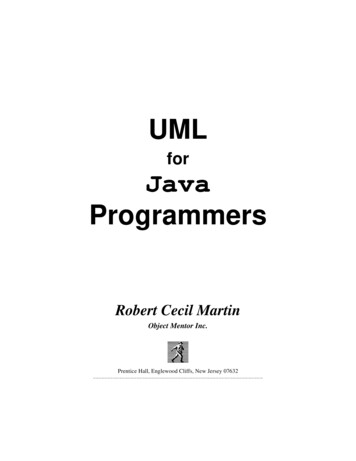
Transcription
UMLforJavaProgrammersRobert Cecil MartinObject Mentor Inc.Prentice Hall, Englewood Cliffs, New Jersey 07632Don’t Panic Do n’t Panic Do n’t Panic Do n’t Panic Don ’t Panic Don ’t Panic Don ’t Panic Don ’t Panic Don’t Panic Don’t Panic Don’t Panic Don’t Panic Don’t Panic Don’t Panic Don’t Panic Don’t Panic Don’t Pan ic Don’t Pan ic Don’t Pan ic Don’t Panic Don’t Panic Don’t Panic Do n’t Panic Do n’t Panic Do n’t Panic Do n’t Panic Don ’t Panic Don ’t Panic Don ’t Panic
Martin, Robert Cecil.The Principles, Practices, & Patterns of Agile Software Development/Robert Cecil Martin.p. cm.“An Alan R. Apt Book.”Includes index.ISBNxxxxxxxxxPublisher: Alan AptProduction Editor:Cover Designer:Copy Editor: 2002 by Prentice-Hall, Inc.A Simon & Schuster CompanyThe author and publisher of this book have used their best efforts in preparing thisbook. These efforts include the development, research, and testing of the theories and programs to determine their effectiveness. The author and publisher shall not be liable in anyevent for incidental or consequential damages in connection with, or arising out of, thefurnishing, performance, or use of these programs.All rights reserved. No part of this book may be reproduced, in any form or by anymeans, without permission in writing from the publisher.Printed in the United States of America1098765ISBN 0-13-203837-44321
PRENTICE-HALL INTERNATIONAL(UK) Limited, LondonPRENTICE-HALL OF A USTRALIA PTY. LIMITED,SydneyPRENTICE-HALL CANADA, I NC., TorontoPRENTICE-HALL HISPANOAMERICANA,S.A., MexicoPRENTICE-HALL OF I NDIA PRIVATE LIMITED,PRENTICE-HALL OF J APAN, INC.,New DelhiTokyoSIMON & SCHUSTER ASIA PTE. LTD.,SingaporeEDITORA PRENTICE-H ALL DO BRASIL , LTDA.,Rio de Janeiro
This book is dedicated to my grandchildren:XXX: the son of Micah and Angelique.Alexis: the daughter of Angela and Matt.It has been said that grandchildren are the desert of life.If that’s so, what am I supposed to do with all the manymain courses I’m not done with yet?
Source Code and Contact Information:Much of the source code presented in this book can be obtained from theObject Mentor Inc. web site. www.objectmentor.com/UMLFJPRobert C. Martin: unclebob@objectmentor.comObject Mentor Inc.: info@objectmentor.comwww.objectmentor.com
iChapter :Chapter 1:Overview of UML for Java Programmers .1Diagram Types .2Class Diagrams.4Object Diagrams.5Sequence Diagrams .6Collaboration Diagrams .6State Diagrams .7Conclusion.8Bibliography .8Chapter 2:Working with Diagrams .9Why Model?.9Why build models of software? .10Why should we build comprehensive designs before coding? .10Making Effective use of UML .10Communicating with Others. .11Back end Documentation .13What to keep, and What to throw away. .14Iterative Refinement .15Behavior first.15Check the structure.17Envisioning the code. .19Iterative Refinement .20Minimalism .21When and how to draw diagrams.21When to draw diagrams, and when to stop. .21CASE Tools.22But what about documentation?.23And Javadocs?.23Conclusion.24Chapter 3:Class Diagrams .25
iiThe Basics .25Classes .25Association .26Multiplicity.26Inheritance .27An Example Class Diagram .28The Details .30Class Stereotypes.30Abstract classes .31Properties.31Aggregation.32Composition .33Multiplicity.34Association Stereotypes .35Inner Classes .36Anonymous Inner Classes.36Association classes.37Association Qualifiers .38Conclusion.38Bibliography .39Chapter 4:Sequence Diagrams .41The Basics .41Objects, Lifelines, Messages, and other odds and ends. .41Creation and Destruction.43Simple Loops.44Cases and Scenarios .44Advanced Concepts.48Loops and Conditions.48Messages that take time.49Asynchronous Messages. .51Multiple Threads .53Active Objects .54Sending Messages to Interfaces. .54Conclusion.56
iiiChapter :Chapter 5:Use Cases .57Writing Use Cases .57What is a use case.58The Primary Course .58Alternate Courses .59What else? .59Use Cases Diagrams .60System Boundary Diagram .60Use Case Relationships .61Conclusion.61Chapter 6:Principles of OOD .63Design Quality .63Design Smells.63Dependency Management .64The Single Reponsibility Principle (SRP) .64The Open Closed Principle (OCP) .66The Liskov Substitution Principle (LSP) .78The Dependency Inversion Principle (DIP).80The Interface Segregation Principle.81Conclusion.82Bibliography .83Chapter 7:The Practices: dX .85Iterative Development.85The Initial Exploration .85Estimating the features .86Spikes .87Planning .87Planning Releases.87Planning Iterations.87The midpoint. .88
ivVelocity Feedback.89Organizing the Iterations into Management Phases.89What’s in an Iteration?.89Developing in Pairs .90Acceptance Tests.90Unit Tests .91Refactoring .91Open Office .92Continuous Integration .92Conclusion.92Bibliography .93Chapter 8:Packages .95Java Packages .95Packages .95Dependencies .96Binary Components -- .jar files.97Principles of Package Design .97The Release/Reuse Equivalency Principle (REP) .98The Common Closure Principle (CCP).98The Common Reuse Principle (CRP) .99The Acyclic Dependencies Principle (ADP).99The Stable Dependencies Principle (SDP).99The Stable Abstractions Principle (SAP) .100Conclusion.100Chapter 9:Object Diagrams .103A Snapshot in Time.103Active Objects.105Conclusion.108Chapter 10:State Diagrams .109
vChapter :The Basics .109Special Events .110Super States .111Initial and Final Pseudo States .113Using FSM Diagrams.113SMC .114ICE: A Case Study .116Conclusion.121Chapter 11:Heuristics and Coffee .123The Mark IV Special Coffee Maker.123A Challenge.126A Common, but Hideous, Coffee Maker Solution.126MissingMethods. .126Vapor Classes.127Imaginary Abstraction.128God Classes .129A Coffee Maker Solution .129Crossed Wires .130The Coffee Maker User Interface.131Use Case 1: User pushes brew button. .131Use Case 2: Containment Vessel not Ready. .132Use Case 3: Brewing Complete. .132Use Case 4: Coffee all gone. .134Implementing the Abstract Model.134Use Case 1. User pushes Brew Button (Mark IV) .135Implementing the isReady() functions. .136Implementing the start() functions. .137How does M4UserInterface.checkButton get called? .138Completing the Coffee Maker.139The Benefits of this design.141How did I really come up with this design?.141Chapter 12:SMC Remote Service: Case Study .153
viCaveat Emptor.153Unit Tests. .154The SMCRemote System.154SMCRemoteClient .154SMCRemoteClient Command Line .155SMCRemote Communication Protocols .155SMCRemoteClient .157The Loggers.164The Remote Sessions. .165RemoteSessionBase .166The Remote Registrar.169The Remote Compiler .171FileCarrier .176SMCRemoteClient Conclusion .177SMCRemoteServer .178SocketService .178SMCRemoteService .183SMCRemoteServer.187ServerSession .190Three Level FSM rdGenerator.202Conclusion.203Tests for SMCRemoteClient .204Tests for SocketService .211Tests for SMCRemoteServer.214Other Tests.224ServerController (SMC Generated) .227Bibliography .235
1Overview of UML for JavaProgrammersThe Unified Modeling Language (UML) is a graphical notation for drawing diagrams ofsoftware concepts. One can use it for drawing diagram
prentice-hall international (uk) limited,london prentice-hall of australia pty.limited, sydney prentice-hall canada,inc., toronto prentice-hall hispanoamericana, s.a., mexico prentice-hall of india private limited, new delhi prentice-hall of japan,inc., tokyo simon &schuster asia pte.ltd., singa