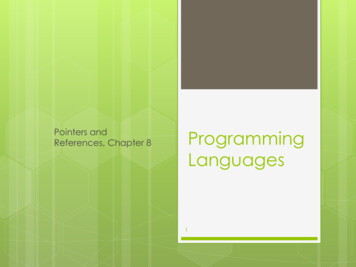
Transcription
Pointers andReferences, Chapter 8ProgrammingLanguages1
2ObjectiveToday’s primary objective: You can compare and contrast pointersand references You know that references are safer You have seen a presentation similar tothe presentations you are to give
3Pointers versus ReferencesI will cover: Why this topic Context Description pointers and references Coding examples Implementation Pros and cons Questions Quiz
4Why This TopicI choose this topic because: I remember how it was before there werereference types Both pointers and reference types are stillused, and extensively References are relevant to functionallanguages
5ContextProgramming languages need variablesthat point to other variables: Recursive types – needed for list, trees Complex objects Dynamically obtained memory
6
7Pointers / References inLanguagesPointers – C, C , C# (unsafe mode), Go(Go implements differently to getadvantages of references)References – C , C#, Java, Python, Ruby,Perl, PHP
8C Pointers & ReferencesPointer – variable that holds the memory address ofanother variableOperators:& - “address of”* - value pointed to by this addressReference – alias, that is, another name for analready existing variableLike a constant pointer with automatic indirection& - “address of”. or - - value being referenced
9Parameter PassingCall-by-value – copy of value is passedDefinitionvoid change (int num) {num 100;}Callint x 5; change(x); x is not changed Call-by-reference – address of the value is passedDefinitionvoid change (int *num) {(*num) 100;}Callint x 5; change(&x); x is changed
10Value & Reference Types
11BoxingBoxing – wrap a value-type variable tomake it a reference typeRelevant to languages that distinguishbetween value and reference types
12Variable ModelsValue model of variables – variables are seen asnamed containersReference model of variables – variables are anamed reference to a variablevalue modelreference modelb: 2c: ba: b c
13Pointers versus ive Type ofLanguageModel forvariables CAdaSmallTalkRubyJava built-in typesJava objectsC# built-in types,structs, C# canuse pointers(unsafe mode)C# objectsValue modelReference Model
14Pointers versus ReferencesPointers correspond to a value modelReferences correspond to a reference model
15C Example DeclarationPointer – stores the address of anothervariableReference – variable which refers toanother variableint i 3;int *ptr &i;int &ref i;// Pointer// Reference
16C Example Useint i 3;int *ptr &i;int &ref i;*ptr 13;ref 13;
Haskell17Haskell - all data are immutable (like Scheme) no need to distinguish between passing anargument by value or by reference compiler is free to choose whicheverrepresentation it thinks will be the most efficient b lazy language (uses delayed computation) if compiler isn't confident that a function willevaluate its arguments, it passes the argument asa "thunk", which is a delayed computation, whichis not passing by value or by reference “thunk” might be shared by multiplecomputations, and we only want it to beevaluated once (rather than every time it's used)
18Scheme Example (references)(define rotate-R(lambda (myList)(reverse (rotate-L (reverse myList) ) )))
19Ocaml Example (references)type char tree Empty Node of char * chr tree * chr tree;;Node (‘C’, Node (‘A’, Empty,Empty), Node (‘E’, Empty, Empty)))
20Ada Example (pointers)type chr tree;type chr tree ptr is acces chr tree;type chr tree is recordleft, right : chr tree ptr;value : character;end record;my ptr : new chr tree
21C Example (pointers)struct chr tree {struct chr tree *left, *right;char val;};my ptr malloc(sizeof(struct chr tree))
22Implementation of ReferencesC and Java standards carefully avoid dictating how acompiler implements referencesEvery C compiler implements references as pointers,consequently references require the same amount ofstorage as pointers
23Implementation of ReferencesWith references - garbage collection possibleGarbage collection - automatic heap storagemanagementProgrammer no longer:Forgets to reclaim storage - memory leakClaims storage too early – dangling references
24PointersProsFlexibleConsDangerousCan use arithmetic forProgrammer needs toefficient array manipulation manage memoryDuring lifetime can point todifferent objectsCan be hard to debugCan get the addressMemory leaks anddangling pointers arepossibleCan be set to null
25ReferencesProsSafeConsCan’t get the value ofthe addressDuring lifetime, onlyreferences one object(constant)Valid reference can neverbe nullSystem can managememory (garbagecollection)Run-time cost togarbage collectDereferencing is doneautomaticallyCompiler can do moreoptimizationCan’t do pointerarithmetic
26Question 1An advantage of pointers over referencesis that:a. Pointers can point to different objectsover their lifetimeb. Compiler can do more optimizationc. Memory can be reclaimed via garbagecollectiond. Safetye. All of the above
27Question 2An advantage of references over pointersis that:a. Dereferencing is done automaticallyb. Memory can be reclaimed via garbagecollectionc. Compiler can do more optimizationd. Safetye. All of the above
Programming languages need variables . References -C , C#, Java, Python, Ruby, Perl, PHP 7. C Pointers & References Pointer -variable that holds the memory address of another variable Operators: & - "address of" * - value pointed to by this address . Language Model for