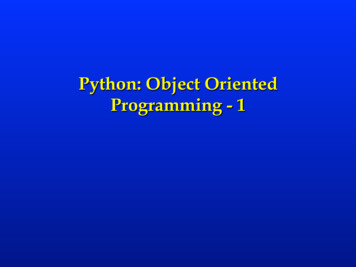
Transcription
Python: Object OrientedProgramming - 1
Objects Python supports different type of data– 125 (int), 24.04 (float), “Hello” (string)– [3,7,8,10] (list)– {“India”:”New Delhi”, “Japan”:”Tokyo”} Each of above is an object Every object has a type, internal datarepresentation and procedures forinteraction. An object is an instance– 125 is an instance of int, Hello is an instance ofstring
Objects In Pyhton everything is an objectCan create an objectCan manipulate objectsCan destroy objectsexplicitly using del or just “forget” about them pythonsystem will reclaim destroyed or inaccessible objects–called “garbage collection”
Objects objects are a data abstraction that capture:(1) an internal representation- through data attributes(2) an interface for interacting with object- through methods (procedures/functions)- defines behaviors but hides implementation
Example: List lists are internally represented as linked list [1,2,3,4]:internal representation should be private manipulation of lists– L[i], L[i:j], – len(), min(), max(), del(L[i])– L.append(), L.extend(), L.remove(), L.reverse()
Classes Classes make it easy to reuse code There is a distinction between creating aclass and using an instance of the class creating the class involvesdefining the class namedefining class attributesfor example, someone wrote code toimplement a list class
Classes
Classes defining a class involvesclass Coordinate (object):definitionof classname ofclassparentclass# define attributes here use a special method init to initializesome data attributes
Classes defining a class involvesclass Coordinate (object):def init (self, x, y):self.x xself.y y creating an instance– c Coordinate(3,4)– origin Coordinate(0,0)
Classes: Methods
Classes: Methods
Classes: MethodsEquivalent
Classes: Methods defining your own print methodclass Coordinate (object):def init (self, x, y):self.x xself.y ydef str (self):return “ “ str(self.x) ”,” str(self.y) ” ”c Coordinate(3,4)print(c) à 3,4
Example: Fraction
Example: Fraction fraction (rational number)class Fraction (object):def init (self, n, d):self.num nself.denom ddef str (self):return str(self.num) ”/” str(self.denom)
Example: Fraction fraction (rational number)add two rational numbers:def add (self, other):num self.num*other.denom self.denom*other.numdenom self.denom*other.denomreturn Fraction(num, denom)
Example: Fraction fraction (rational number)subtract two rational numbers:def subtract (self, other):num self.num*other.denom self.denom*other.numdenom self.denom*other.denomreturn Fraction(num, denom)
Example: Fraction fraction (rational number)multiply two rational numbers:def multiply(self, other):num self.num*other.numdenom self.denom*other.denomreturn Fraction(num, denom)
Example: Fraction fraction (rational number)divide two rational numbers:def divide(self, other):num self.num*other.denomdenom self.denom*other.numreturn Fraction(num, denom)
Example: Fraction fraction (rational number)def float (self):return self.num/self.denomdef inverse(self):return self.denom/self.numdef reduce(self): .
Python: Object Oriented Programming - 1 . Objects Python supports different type of data . string) – [3,7,8,10] (list) – {“India”:”New Delhi”, “Japan”:”Tokyo”} Each of above is an object Every object has a type, internal data representation and procedures for interaction.