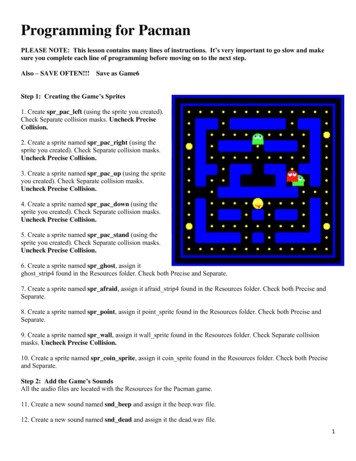
Transcription
Programming for PacmanPLEASE NOTE: This lesson contains many lines of instructions. It’s very important to go slow and makesure you complete each line of programming before moving on to the next step.Also – SAVE OFTEN!!!Save as Game6Step 1: Creating the Game’s Sprites1. Create spr pac left (using the sprite you created).Check Separate collision masks. Uncheck PreciseCollision.2. Create a sprite named spr pac right (using thesprite you created). Check Separate collision masks.Uncheck Precise Collision.3. Create a sprite named spr pac up (using the spriteyou created). Check Separate collision masks.Uncheck Precise Collision.4. Create a sprite named spr pac down (using thesprite you created). Check Separate collision masks.Uncheck Precise Collision.5. Create a sprite named spr pac stand (using thesprite you created). Check Separate collision masks.Uncheck Precise Collision.6. Create a sprite named spr ghost, assign itghost strip4 found in the Resources folder. Check both Precise and Separate.7. Create a sprite named spr afraid, assign it afraid strip4 found in the Resources folder. Check both Precise andSeparate.8. Create a sprite named spr point, assign it point sprite found in the Resources folder. Check both Precise andSeparate.9. Create a sprite named spr wall, assign it wall sprite found in the Resources folder. Check Separate collisionmasks. Uncheck Precise Collision.10. Create a sprite named spr coin sprite, assign it coin sprite found in the Resources folder. Check both Preciseand Separate.Step 2: Add the Game’s SoundsAll the audio files are located with the Resources for the Pacman game.11. Create a new sound named snd beep and assign it the beep.wav file.12. Create a new sound named snd dead and assign it the dead.wav file.1
13. Create a new sound name snd won and assign it the won.wav file14. Create a new sound name snd click and assign it the click.wav file15. Create a new sound name snd catch and assign it the catch.wav fileStep 3: Create the Background Tiles16. Click on Resources Create Background. Create a new background called bckgd level1, assign it the level1.pnggraphic file.17. Create a new background called bckgd level2, assign it the level2.png graphic file.18. Create a new background called bckgd level3, assign it the level3.png graphic file.19. Create a new background called bckgd level4, assign it the level4.png graphic file.Step 4: Creating the Game’s Objects20. Add a new object named obj pacman and assign it the spr pac standsprite. Check the visible box - leave solid unchecked. Enter -1 in the Depthbox.FYI - The default value for an object’s depth is 0, unless you set itto a different value in the object properties. The higher the valuethe further the instance is away. (You can also use negativevalues.) Instances with higher depth will lie behind instanceswith a lower depth. Setting the depth will guarantee that theinstances are displayed in the order you want (e.g. the plane infront of the cloud). Background instances should have a high(positive) depth, and foreground instances should have a low(negative) depth. In this case, we are setting the object of Pacmanto be in the foreground – in front of the points and coins.21. Add a new object named obj ghost, assigning it the spr ghost sprite.Check the visible box - leave solid unchecked. Enter -2 in the depth box.FYI - Entering a Depth of -2 will make the ghosts appear to be infront of Pacman, so it looks like the ghosts “attacked” him.22. Add a new object named obj afraid, assigning it the spr afraid sprite.Check the visible box - leave solid unchecked. Enter 0 in the depth box.FYI - This will make it appear that Pacman “ate” the scared ghosts, because the scared ghosts will be behindPacman.In the Parent field, select obj ghost from the drop down. This will enable obj afraid to take on the sameprogramming as obj ghost without having to repeat the same programming for this object.2
23. Add a new object named obj wall, assigning it the spr wall sprite. Do NOT check visible. Check the solid box.Enter 0 in the depth box.Why wouldn’t the wall be visible? Because we want to see the backgrounds behind the wall, but need thewall to serve as the object that the other objects collide against.24. Add a new object named obj point, assigning it the spr point sprite. Check the visible box - leave solidunchecked. Enter 0 in the depth box.25. Add a new object named obj coin, assigning it the spr coin sprite. Check the visible box - leave solidunchecked. Enter 0 in the depth box.Step 5: Creating Rooms26. Click on Create a room button.Under the settings folder tab, name the room room0.Enter Pacman as the Caption for the room (you will see later where thisdisplays).Set the width and height at 480.Set the room speed at 20.FYI – Speed is the # of steps per second.Under the backgrounds tab, select bckgd level1 as yourimage.Change the Snap X and Snap Y to 32 each.3
Add the objects to the room so it looks like the following and click the green to save:(room0)FYI - The wall object is placed over the blue areas (Remember, the wall object is not visible—the blue willappear to be the playing field).27. Following the same steps as listed above, create room1, using bckgd level2 as the background image andchange the room speed to 25. Add the objects to the room so it looks like the following:(room1)4
28. Following the same steps as listed above, create room2, using bckgd level3 as the background image andchange the room speed to 30. Add the objects to the room so it looks like the following:(room2)29. Following the same steps as listed above, create room3, using bckgd level4 as the background image andchange the room speed to 35. Add the objects to the room so it looks like the following:(room3)5
Step 6: Programming the ObjectsObj pacman (13 Events)Event 1: CreateAction: Change SpriteApplies to: SelfSprite: spr pac standSubimage: 0Speed: 0.5Event 2: StepAction: Test Instance CountObject: obj pointNumber: 0Operation: Equal toAction: Start BlockAction: Play SoundSound: sound wonLoop: FalseAction: SleepMilliseconds: 2000Redraw: trueAction: Check NextAction: Next RoomTransition: no effectAction: ElseAction: Start BlockAction: Show HighscoreBackground: No BackgroundBorder: ShowNew color: select a font color for the new score to displayOther color: Select a font color for the other high scores to displayFont: Select a fontAction: End GameAction: End BlockAction: End Block6
QUESTION: Why do you need 2 End Blocks?ANSWER: Every start block needs a corresponding End Block.Event 3: Collision Event with obj ghostAction: Play soundSound: snd deadLoop: FalseAction: SleepMilliseconds: 1500Redraw: trueAction: Jump to StartApplies to: Object obj ghostAction: Jump to StartApplies to: Object obj afraidAction: Move FixedApplies to: SelfDirections: Select middle button (to stop movement)Speed: 0Relative is NOT checkedAction: Change SpriteApplies to: SelfSprite: spr pac standSubimage: 0Speed: 0.5Action: Jump to StartApplies to: SelfAction: Set LivesNew lives: -1Relative IS checkedEvent 4: Collision Event with obj afraidAction: Play soundSound: snd catchLoop: FalseAction: Jump to StartApplies to: OtherAction: Change InstanceApplies to: obj ghostPerform Events: Not7
Action: Set ScoreNew Score: 100Relative IS checkedEvent 5: Collision with obj wallAction: Align to GridApplies to: SelfSnap Hor: 32Snap Vert: 32Action: Move FixedApplies to: SelfDirections: Center button(to stop movement)Speed: 0Relative is NOT checkedAction: Change SpriteApplies to: SelfSprite: spr pac standSubimage: 0Speed: 0.5Event 6: Collision with obj pointAction: Destroy InstanceApplies to: OtherAction: Play SoundSound: snd clickLoop: FalseAction: Set ScoreNew Score: 10Relative IS checkedEvent 7: Collision with obj coinAction: Play SoundSound: snd beepLoop: FalseAction: Destroy InstanceApplies to: OtherAction: Change InstanceApplies to: Object obj afraidChange into: obj ghostPerform Events: not8
Action: Change InstanceApplies to: Object obj ghostChange into: obj afraidPerform Events: notAction: Set AlarmApplies to: Other obj afraidNumber of steps: 160In Alarm no: Alarm 0Event 8: Keyboard Left Action: Check EmptyApplies to: Selfx: -4y: 0Objects: Only SolidRelative IS checkedAction: Start BlockAction: Check GridApplies to: SelfSnap Hor: 32Snap Vert: 32Action: Start BlockAction: Moved FixedApplies to: SelfDirection: Left ArrowSpeed: 4Relative is NOT checkedAction: Change SpriteApplies to: SelfSprite: spr pac leftSubimage: -1Speed: 0.5Action: End BlockAction: End BlockEvent 9: Keyboard Right Action: Check EmptyApplies to: Selfx: 4y: 0Objects: Only SolidRelative IS checked9
Action: Start BlockAction: Check GridApplies to: SelfSnap Hor: 32Snap Vert: 32Action: Start BlockAction: Moved FixedApplies to: SelfDirection: Right ArrowSpeed: 4Relative is NOT checkedAction: Change SpriteApplies to: SelfSprite: spr pac rightSubimage: -1Speed: 0.5Action: End BlockAction: End BlockEvent 9: Keyboard Up Action: Check EmptyApplies to: Selfx: 0y: -4Objects: Only SolidRelative IS checkedAction: Start BlockAction: Check GridApplies to: SelfSnap Hor: 32Snap Vert: 32Action: Start BlockAction: Moved FixedApplies to: SelfDirection: Up ArrowSpeed: 4Relative is NOT checked10
Action: Change SpriteApplies to: SelfSprite: spr pac upSubimage: -1Speed: 0.5Action: End BlockAction: End BlockEvent 10: Keyboard Down Action: Check EmptyApplies to: Selfx: 0y: 4Objects: Only SolidRelative IS checkedAction: Start BlockAction: Check GridApplies to: SelfSnap Hor: 32Snap Vert: 32Action: Start BlockAction: Moved FixedApplies to: SelfDirection: Down ArrowSpeed: 4Relative is NOT checkedAction: Change SpriteApplies to: SelfSprite: spr pac downSubimage: -1Speed: 0.5Action: End BlockAction: End BlockEvent 11: Other Outside RoomAction: Wrap ScreenApplies to: SelfDirection: in both directions11
Event 12: Other Game StartAction: Set LivesNew Lives: 3Relative is NOT checkedAction: Set ScoreNew Score: 0Relative is NOT checkedAction: Score CaptionShow Score: showScore Caption: Score: (add a space)Show Lives: showLives Caption: Lives: (add a space)Show Health: don’t showHealth Caption:FYI – Remember when youentered Pacman as the Captionfor the Room in the RoomSettings? Here is an example ofhow that displays (along with theScore, Lives and Health captions,if those are selected to show).What you enter for the Score,Lives and Health Captionsdisplay exactly as typed. Youneed to add a space so the valuesdon’t butt right up against thecaption.Event 13: Other No More LivesAction: Show High ScoreBackground: No BackgroundBorder: ShowNew color: select a font color for thenew score to displayOther color: Select a font color for theother high scores to displayFont: Select a fontAction: End Game12
Obj ghost (4 Events)Event 1: CreateAction: Change SpriteApplies to: SelfSprite: spr ghostSubimage: random(4)Speed: 0Action: Moved FixedApplies to: SelfDirections: select Up, Down, Left and Right arrowsSpeed: 4Relative is NOT checkedEvent 2: StepAction: Check GridApplies to: SelfSnap hor: 32Snap vert: 32Action: Start BlockAction: Test ExpressionApplies to: SelfExpression: hspeed 0Action: Start BlockAction: Check EmptyApplies to: Selfx: -4y: 0Objects: only solidsRelative IS checkedAction: Start BlockAction: Test ChanceSides: 2Action: Move FixedApplies to: SelfDirections: select Left ArrowSpeed: 4Relative is NOT checkedAction: End BlockAction: Check Empty13
Applies to: Selfx: 4y: 0Objects: only solidsRelative IS checkedAction: Start BlockAction: Test ChanceSides: 2Action: Move FixedApplies to: SelfDirection: Select right arrowSpeed: 4Relative is NOT checkedAction: End BlockAction: End BlockAction: ElseAction: Start BlockAction: Action: Check EmptyApplies to: Selfx: 0y: -4Objects: only solidsRelative IS checkedAction: Start BlockAction: Test ChanceSides: 2Action: Move FixedApplies to: SelfDirection: Select up arrowSpeed: 4Relative is NOT checkedAction: End BlockAction: Start Block14
Action: Action: Check EmptyApplies to: Selfx: 0y: 4Objects: only solidsRelative IS checkedAction: Start BlockAction: Test ChanceSides: 2Action: Move FixedApplies to: SelfDirection: Select down arrowSpeed: 4Relative is NOT checkedAction: End BlockAction: End BlockAction: End BlockEvent 3: Collision with obj wallAction: Align to GridApplies to: SelfSnap hor: 32Snap vert: 32Action: Reverse HorizontalApplies to: SelfAction: Reverse VerticalApplies to: SelfAction: Test ExpressionApplies to: SelfExpression: hspeed 0Action: Start BlockAction: Check EmptyApplies to: Selfx: -4y: 0Objects: only solidsRelative IS checked15
Action: Start BlockAction: Test ChanceSides: 2Action: Move FixedApplies to: SelfDirections: select Left ArrowSpeed: 4Relative is NOT checkedAction: End BlockAction: Check EmptyApplies to: Selfx: 4y: 0Objects: only solidsRelative IS checkedAction: Start BlockAction: Test ChanceSides: 2Action: Move FixedApplies to: SelfDirection: Select right arrowSpeed: 4Relative is NOT checkedAction: End BlockAction: End BlockAction: ElseAction: Start BlockAction: Action: Check EmptyApplies to: Selfx: 0y: -4Objects: only solidsRelative IS checkedAction: Start BlockAction: Test ChanceSides: 216
Action: Move FixedApplies to: SelfDirection: Select up arrowSpeed: 4Relative is NOT checkedAction: End BlockAction: Action: Check EmptyApplies to: Selfx: 0y: 4Objects: only solidsRelative IS checkedAction: Start BlockAction: Test ChanceSides: 2Action: Move FixedApplies to: SelfDirection: Select down arrowSpeed: 4Relative is NOT checkedAction: End BlockAction: End BlockEvent 4: Other Outside of RoomAction: Wrap ScreenApplies to: SelfDirection: in both directionsObj afraid (1 Event)Event 1: Alarm Alarm 0Action: Change InstanceApplies to: SelfChange into: obj ghostPerform events: notObj wallNo programming requiredObj pointNo programming required17
Obj coinNo programming requiredSAVE AS GAME6 and test for errors. Debug if necessary.ASSIGNMENT:For all of Step 6: Programming the Objects, explain section by section what is being programmed.Hint: Use HELP if you are unsure.18
Programming for Pacman PLEASE NOTE: This lesson contains many lines of instructions. It’s very important to go slow and make sure you complete each line of programming before moving on to the next step. Also – SAVE OFTEN!!! Save as Game6 Step 1: Creating the Game’s Sprit