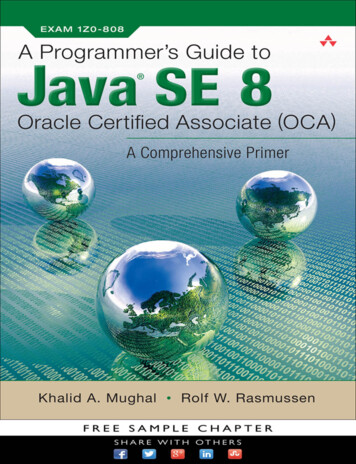
Transcription
A Programmer’s Guide toJava SE 8 Oracle Certified Associate (OCA)
This page intentionally left blank
A Programmer’s Guide toJava SE 8 Oracle Certified Associate (OCA)A Comprehensive PrimerKhalid A. MughalRolf W. RasmussenBoston Columbus Indianapolis New York San Francisco Amsterdam Cape TownDubai London Madrid Milan Munich Paris Montreal Toronto Delhi Mexico CitySão Paulo Sydney Hong Kong Seoul Singapore Taipei Tokyo
Many of the designations used by manufacturers and sellers to distinguish their productsare claimed as trademarks. Where those designations appear in this book, and the publisherwas aware of a trademark claim, the designations have been printed with initial capitalletters or in all capitals.The authors and publisher have taken care in the preparation of this book, but make noexpressed or implied warranty of any kind and assume no responsibility for errors or omissions. No liability is assumed for incidental or consequential damages in connection with orarising out of the use of the information or programs contained herein.For information about buying this title in bulk quantities, or for special sales opportunities(which may include electronic versions; custom cover designs; and content particular toyour business, training goals, marketing focus, or branding interests), please contact ourcorporate sales department at corpsales@pearsoned.com or (800) 382-3419.For government sales inquiries, please contact governmentsales@pearsoned.com.For questions about sales outside the U.S., please contact intlcs@pearson.com.Visit us on the Web: informit.com/awLibrary of Congress Control Number: 2016937073Copyright 2017 Pearson Education, Inc.All rights reserved. Printed in the United States of America. This publication is protected bycopyright, and permission must be obtained from the publisher prior to any prohibitedreproduction, storage in a retrieval system, or transmission in any form or by any means,electronic, mechanical, photocopying, recording, or likewise. For information regardingpermissions, request forms and the appropriate contacts within the Pearson EducationGlobal Rights & Permissions Department, please visit www.pearsoned.com/permissions/.ISBN-13: 978-0-13-293021-5ISBN-10: 0-13-293021-8Text printed in the United States on recycled paper at RR Donnelley in Crawfordsville, Indiana.First printing, July 2016
To the loving memory of my mother, Zubaida Begum,and my father, Mohammed Azim—K.A.M.For Olivia E. Rasmussen andLouise J. Dahlmo—R.W.R.
This page intentionally left blank
Contents viiPrefacexxix1Basics of Java Programming2Language Fundamentals273Declarations474 Access Control955Operators and Expressions11436 Control Flow1997Object-Oriented Programming2638Fundamental Classes3419Object Lifetime383The ArrayList E Class and Lambda Expressions4131011 Date and Time461vii
CONTENTS OVERVIEWviiiATaking the Java SE 8 Programmer I Exam507B Exam Topics: Java SE 8 Programmer I515C Annotated Answers to Review Questions519DSolutions to Programming Exercises553EMock Exam: Java SE 8 Programmer I571F Annotated Answers to Mock Exam IIndex605619
viiPreface1Basics of Java oductionClassesDeclaring Members: Fields and MethodsObjectsClass Instantiation, Reference Values, and ReferencesObject AliasesInstance MembersInvoking MethodsStatic MembersInheritanceAssociations: Aggregation and CompositionTenets of JavaReview QuestionsJava ProgramsSample Java ApplicationEssential Elements of a Java ApplicationCompiling and Running an ApplicationProgram OutputFormatted Outputxxix122344667710121313151616171818ix
CONTENTSx1.122The Java EcosystemObject-Oriented ParadigmInterpreted: The JVMArchitecture-Neutral and Portable BytecodeSimplicityDynamic and DistributedRobust and SecureHigh Performance and MultithreadedReview QuestionsChapter SummaryProgramming ExerciseLanguage Fundamentals2.12.22.32.4Basic Language ElementsLexical TokensIdentifiersKeywordsSeparatorsLiteralsInteger LiteralsFloating-Point LiteralsUnderscores in Numerical LiteralsBoolean LiteralsCharacter LiteralsString LiteralsWhitespaceCommentsReview QuestionsPrimitive Data TypesThe Integer TypesThe char TypeThe Floating-Point TypesThe boolean TypeReview QuestionsVariable DeclarationsDeclaring and Initializing VariablesReference VariablesInitial Values for VariablesDefault Values for FieldsInitializing Local Variables of Primitive Data TypesInitializing Local Reference VariablesLifetime of VariablesReview QuestionsChapter SummaryProgramming 3232343535363738383839404041414242434344454646
ass DeclarationsMethod DeclarationsStatementsInstance Methods and the Object Reference thisMethod OverloadingConstructorsThe Default ConstructorOverloaded ConstructorsReview QuestionsArraysDeclaring Array VariablesConstructing an ArrayInitializing an ArrayUsing an ArrayAnonymous ArraysMultidimensional ArraysSorting ArraysSearching ArraysReview QuestionsParameter PassingPassing Primitive Data ValuesPassing Reference ValuesPassing ArraysArray Elements as Actual Parametersfinal ParametersVariable Arity MethodsCalling a Variable Arity MethodVariable Arity and Fixed Arity Method CallsThe main() MethodProgram ArgumentsEnumerated TypesDeclaring Type-safe EnumsUsing Type-safe EnumsSelected Methods for Enum TypesReview QuestionsChapter SummaryProgramming ExerciseAccess Control4.14.2Java Source File StructurePackagesDefining PackagesUsing PackagesCompiling Code into 73757778808182848586878788899092939596979899105
CONTENTSxii4.34.44.54.64.74.85Running Code from PackagesSearching for ClassesReview QuestionsScope RulesClass Scope for MembersBlock Scope for Local VariablesAccessibility Modifiers for Top-Level Type DeclarationsNon-Accessibility Modifiers for Classesabstract Classesfinal ClassesReview QuestionsMember Accessibility Modifierspublic Membersprotected MembersDefault Accessibility for Membersprivate MembersReview QuestionsNon-Accessibility Modifiers for Membersstatic Membersfinal Membersabstract Methodssynchronized Methodsnative Methodstransient Fieldsvolatile FieldsReview QuestionsChapter SummaryProgramming ExerciseOperators and Expressions5.15.25.35.4ConversionsWidening and Narrowing Primitive ConversionsWidening and Narrowing Reference ConversionsBoxing and Unboxing ConversionsOther ConversionsType Conversion ContextsAssignment ContextMethod Invocation ContextCasting Context of the Unary Type Cast Operator: (type)Numeric Promotion ContextPrecedence and Associativity Rules for OperatorsEvaluation Order of OperandsLeft-Hand Operand Evaluation FirstOperand Evaluation before Operation ExecutionLeft-to-Right Evaluation of Argument 5145146147147148148149150152152153154
55.165.17Representing IntegersCalculating Two’s ComplementConverting Binary Numbers to DecimalsConverting Decimals to Binary NumbersRelationships among Binary, Octal, and Hexadecimal NumbersThe Simple Assignment Operator Assigning Primitive ValuesAssigning ReferencesMultiple AssignmentsType Conversions in an Assignment ContextReview QuestionsArithmetic Operators: *, /, %, , Arithmetic Operator Precedence and AssociativityEvaluation Order in Arithmetic ExpressionsRange of Numeric ValuesUnary Arithmetic Operators: -, Multiplicative Binary Operators: *, /, %Additive Binary Operators: , Numeric Promotions in Arithmetic ExpressionsArithmetic Compound Assignment Operators: * , / , % , , - Review QuestionsThe Binary String Concatenation Operator Variable Increment and Decrement Operators: , -The Increment Operator The Decrement Operator -Review QuestionsBoolean ExpressionsRelational Operators: , , , EqualityPrimitive Data Value Equality: , ! Object Reference Equality: , ! Object Value EqualityBoolean Logical Operators: !, , &, Operand Evaluation for Boolean Logical OperatorsBoolean Logical Compound Assignment Operators: & , , Conditional Operators: &&, Short-Circuit EvaluationInteger Bitwise Operators: , &, , Bitwise Compound Assignment Operators: & , , Review QuestionsThe Conditional Operator: ?:Other Operators: new, [], instanceof, - Review QuestionsChapter SummaryProgramming 2183184185185186187189192192194195196197197
CONTENTSxiv6Control Flow6.16.26.36.46.56.66.76.86.96.107Overview of Control Flow StatementsSelection StatementsThe Simple if StatementThe if-else StatementThe switch StatementReview QuestionsIteration StatementsThe while StatementThe do-while StatementThe for(;;) StatementThe for(:) StatementTransfer StatementsLabeled StatementsThe break StatementThe continue StatementThe return StatementReview QuestionsStack-Based Execution and Exception PropagationException TypesThe Exception ClassThe RuntimeException ClassThe Error ClassChecked and Unchecked ExceptionsDefining Customized ExceptionsException Handling: try, catch, and finallyThe try BlockThe catch ClauseThe finally ClauseThe throw StatementThe throws ClauseOverriding the throws ClauseAdvantages of Exception HandlingReview QuestionsChapter SummaryProgramming ExercisesObject-Oriented Programming7.17.2Single Implementation InheritanceRelationships: is-a and has-aThe Supertype–Subtype RelationshipOverriding MethodsInstance Method OverridingCovariant return in Overriding MethodsOverriding versus 9251253254255258258263264266267268268273273
48Hiding MembersField HidingStatic Method HidingThe Object Reference superReview QuestionsChaining Constructors Using this() and super()The this() Constructor CallThe super() Constructor CallReview QuestionsInterfacesDefining InterfacesAbstract Methods in InterfacesImplementing InterfacesExtending InterfacesInterface ReferencesDefault Methods in InterfacesStatic Methods in InterfacesConstants in InterfacesReview QuestionsArrays and SubtypingArrays and Subtype CovarianceArray Store CheckReference Values and ConversionsReference Value Assignment ConversionsMethod Invocation Conversions Involving ReferencesOverloaded Method ResolutionReference Casting and the instanceof OperatorThe Cast OperatorThe instanceof OperatorReview QuestionsPolymorphism and Dynamic Method LookupInheritance versus AggregationBasic Concepts in Object-Oriented DesignEncapsulationCohesionCouplingReview QuestionsChapter SummaryProgramming ExercisesFundamental Classes8.18.2Overview of the java.lang PackageThe Object ClassReview 29331334335335336336338339341342342346
CONTENTSxvi8.38.48.59The Wrapper ClassesCommon Wrapper Class ConstructorsCommon Wrapper Class Utility MethodsNumeric Wrapper ClassesThe Character ClassThe Boolean ClassReview QuestionsThe String ClassImmutabilityCreating and Initializing StringsThe CharSequence InterfaceReading Characters from a StringComparing StringsCharacter Case in a StringConcatenation of StringsJoining of CharSequence ObjectsSearching for Characters and SubstringsExtracting SubstringsConverting Primitive Values and Objects to StringsFormatted StringsReview QuestionsThe StringBuilder and StringBuffer ClassesThread-SafetyMutabilityConstructing String BuildersReading and Changing Characters in String BuildersConstructing Strings from String BuildersAppending, Inserting, and Deleting Characters in String BuildersControlling String Builder CapacityReview QuestionsChapter SummaryProgramming ExercisesObject Lifetime9.19.29.39.49.59.69.79.89.9Garbage CollectionReachable ObjectsFacilitating Garbage CollectionObject FinalizationFinalizer ChainingInvoking Garbage Collection ProgrammaticallyReview QuestionsInitializersField Initializer ExpressionsDeclaration Order of Initializer ExpressionsStatic Initializer 82383384384386390391393396399400401402
CONTENTSxvii9.109.1110The ArrayList E Class and Lambda Expressions10.110.211Declaration Order of Static InitializersInstance Initializer BlocksDeclaration Order of Instance InitializersConstructing Initial Object StateReview QuestionsChapter SummaryThe ArrayList E ClassListsDeclaring References and Constructing ArrayListsModifying an ArrayListQuerying an ArrayListTraversing an ArrayListConverting an ArrayList to an ArraySorting an ArrayListArrays versus ArrayListReview QuestionsLambda ExpressionsBehavior ParameterizationFunctional InterfacesDefining Lambda ExpressionsType Checking and Execution of Lambda ExpressionsFiltering Revisited: The Predicate T Functional InterfaceReview QuestionsChapter SummaryProgramming ExerciseDate and Time11.111.211.311.4Basic Date and Time ConceptsWorking with Temporal ClassesCreating Temporal ObjectsQuerying Temporal ObjectsComparing Temporal ObjectsCreating Modified Copies of Temporal ObjectsTemporal ArithmeticWorking with PeriodsCreating PeriodsQuerying PeriodsCreating Modified Copies of PeriodsMore Temporal ArithmeticReview QuestionsFormatting and ParsingDefault 468470470474476476478479479483486487
CONTENTSxviiiPredefined FormattersLocalized FormattersCustomized FormattersReview QuestionsChapter SummaryProgramming ExerciseA488490495500502503Taking the Java SE 8 Programmer I Exam507A.1A.2Preparing for the ExamRegistering for the ExamContact InformationObtaining an Exam VoucherSigning Up for the TestAfter Taking the ExamHow the Exam Is ConductedThe Testing LocationsUtilizing the Allotted TimeThe Exam ProgramThe Exam ResultThe QuestionsAssumptions about the Exam QuestionsTypes of Questions AskedTypes of Answers ExpectedTopics Covered by the 2512513B Exam Topics: Java SE 8 Programmer I515C Annotated Answers to Review Questions519D Solutions to Programming Exercises553E571A.3A.4Mock Exam: Java SE 8 Programmer IF Annotated Answers to Mock Exam IIndex605619
Figures1.1UML Notationfor ClassesChapter111.2 UML Notation for Objects1.3 Aliases1.4 Class Diagram Showing Static Members of a Class1.5 Members of a Class1.6 Class Diagram Depicting Inheritance Relationship1.7 Class Diagram Depicting Associations1.8 Class Diagram Depicting Composition2.1PrimitiveChapter2 27Data Types in Java3.1ArrayArraysChapter 3of473.2 Parameter Passing: Primitive Data Values3.3 Parameter Passing: Reference Values3.4 Parameter Passing: Arrays4.1Java SourceChapter4 95 File Structure4.2 Package Hierarchy4.3 File Hierarchy4.4 Searching for Classes4.5 Block Scope4.6 Public Accessibility for Members4.7 Protected Accessibility for Members4.8 Default Accessibility for Members4.9 Private Accessibility for Members5.1WideningPrimitive ConversionsChapter5 1435.2 Converting among Binary, Octal, and Hexadecimal Numbers5.3 Overflow and Underflow in Floating-Point Arithmetic5.4 Numeric Promotion in Arithmetic Expressions6.1ActivityDiagram for if StatementsChapter6 1996.2 Activity Diagram for a switch Statement6.3 Activity Diagram for the while Statement6.4 Activity Diagram for the do-while Statement6.5 Activity Diagram for the for Statement6.6 Enhanced for Statement6.7 Method 28129144158166170201204213214215217231xix
FIGURESxx6.8 Exception Propagation6.9 Partial Exception Inheritance Hierarchy6.10 The try-catch-finally Construct6.11 Exception Handling (Scenario 1)6.12 Exception Handling (Scenario 2)6.13 Exception Handling (Scenario 3)7.1InheritanceChapter7 263Hierarchy7.2 Inheritance Hierarchy for Example 7.2 and Example 7.37.3 Inheritance Hierarchies7.4 Inheritance Relationships for Interface Constants7.5 Reference Type Hierarchy: Arrays and Subtype Covariance7.6 Type Hierarchy That Illustrates Polymorphism7.7 Implementing Data Structures by Inheritance and Aggregation8.1Partial8InheritanceHierarchy in the java.lang PackageChapter3418.2 Converting Values among Primitive, Wrapper, and String Types9.1MemoryOrganization at RuntimeChapter9 38310.1Partial10ArrayListChapter413 Inheritance HierarchyChapter 11 6415
TablesChapter 1 11.1ChapterChapterChapterChapter1.22.12 3.13 474.14 954.24.34.44.55.15 1435.25.35.45.55.65.75.85.95.10Terminology for Class MembersFormat Specifier ExamplesKeywords in JavaReserved Literals in JavaReserved Keywords Not Currently in UseSeparators in JavaExamples of LiteralsExamples of Decimal, Binary, Octal, and Hexadecimal LiteralsExamples of Character LiteralsEscape SequencesExamples of Escape Sequence \dddRange of Integer ValuesRange of Character ValuesRange of Floating-Point ValuesBoolean ValuesSummary of Primitive Data TypesDefault ValuesParameter Passing by ValueAccessing Members within a ClassSummary of Accessibility Modifiers for Top-Level TypesSummary of Non-Accessibility Modifiers for ClassesSummary of Accessibility Modifiers for MembersSummary of Non-Accessibility Modifiers for MembersSelected Conversion Contexts and Conversion CategoriesOperator SummaryRepresenting Signed byte Values Using Two’s ComplementExamples of Truncated ValuesArithmetic OperatorsExamples of Arithmetic Expression EvaluationArithmetic Compound Assignment OperatorsRelational OperatorsPrimitive Data Value Equality OperatorsReference Equality 122129139147151155162164169172180181182xxi
5.145.155.165.175.186.16 1997.17 2637.27.38 10.191034138341310.21111.146111.211.311.411.5Truth Values for Boolean Logical OperatorsBoolean Logical Compound Assignment OperatorsConditional OperatorsTruth Values for Conditional OperatorsInteger Bitwise OperatorsResult Table for Bitwise OperatorsExamples of Bitwise OperationsBitwise Compound Assignment OperatorsThe return StatementOverriding versus OverloadingSame Signature for Subclass and Superclass MethodTypes and ValuesSummary of Arrays versus ArrayListsSelected Functional Interfaces from the java.util.function PackageSelected Common Method Prefix of the Temporal ClassesSelected ISO-Based Predefined Formatters for Date and TimeFormat Styles for Date and TimeCombination of Format Styles and Localized FormattersSelected Date/Time Pattern 63489490491496
Examples1.1Basic Elementsof a Class DeclarationChapter111.2 Static Members in Class Declaration1.3 Defining a Subclass1.4 An Application1.5 Formatted Output2.1Defaultfor FieldsChapter2 Values272.2 Flagging Uninitialized Local Variables of Primitive Data Types2.3 Flagging Uninitialized Local Reference Variables3.1Using 3theChapter47this Reference3.2 Namespaces3.3 Using Arrays3.4 Using Anonymous Arrays3.5 Using Multidimensional Arrays3.6 Passing Primitive Values3.7 Passing Reference Values3.8 Passing Arrays3.9 Array Elements as Primitive Data Values3.10 Array Elements as Reference Values3.11 Calling a Variable Arity Method3.12 Passing Program Arguments3.13 Using Enums4.1DefiningPackages and Using Type ImportChapter4 954.2 Single Static Import4.3 Avoiding the Interface Constant Antipattern4.4 Importing Enum Constants4.5 Shadowing Static Import4.6 Conflict in Importing Static Method with the Same Signature4.7 Class Scope4.8 Accessibility Modifiers for Classes and Interfaces4.9 Abstract Classes4.10 Public Accessibility of Members4.11 Accessing Static Members4.12 Using final 102102103104105116118121125132134xxiii
EXAMPLESxxiv4.13 Synchronized Methods5.1EvaluationChapter5 143Order of Operands and Arguments5.2 Numeric Promotion in Arithmetic Expressions5.3 Short-Circuit Evaluation Involving Conditional Operators5.4 Bitwise Operations6.1Fall-ThroughChapter6 199 in a switch Statement6.2 Using break in a switch Statement6.3 Nested switch Statement6.4 Strings in switch Statement6.5 Enums in switch Statement6.6 The break Statement6.7 Labeled break Statement6.8 continue Statement6.9 Labeled continue Statement6.10 The return Statement6.11 Method Execution6.12 The try-catch Construct6.13 Exception Propagation6.14 The try-catch-finally Construct6.15 The try-finally Construct6.16 The finally Clause and the return Statement6.17 Throwing Exceptions6.18 The throws Clause7.1ExtendingClasses: Inheritance and AccessibilityChapter7 2637.2 Overriding, Overloading, and Hiding7.3 Using the super Keyword7.4 Constructor Overloading7.5 The this() Constructor Call7.6 The super() Constructor Call7.7 Implementing Interfaces7.8 Default Methods in Interfaces7.9 Default Methods and Multiple Inheritance7.10 Static Methods in Interfaces7.11 Constants in Interfaces7.12 Inheriting Constants in Interfaces7.13 Assigning and Passing Reference Values7.14 Choosing the Most Specific Method (Simple Case)7.15 Overloaded Method Resolution7.16 The instanceof and Cast Operators7.17 Using the instanceof Operator7.18 Polymorphism and Dynamic Method Lookup7.19 Implementing Data Structures by Inheritance and Aggregation8.1Methodsin the Object ClassChapter8 3418.2 String Representation of Integers8.3 String Construction and Equality8.4 Reading Characters from a 99301302304312317318322323330332344353359362
EXAMPLESxxv9.1GarbageCollection EligibilityChapter9 3839.2 Using Finalizers9.3 Invoking Garbage Collection9.4 Initializer Expression Order and Method Calls9.5 Static Initializers and Forward References9.6 Instance Initializers and Forward References9.7 Object State Construction9.8 Initialization Anomaly under Object State Construction10.1Using 10an ArrayListChapter41310.2 Implementing Customized Methods for Filtering an ArrayList10.3 Implementing an Interface for Filtering an ArrayList10.4 User-Defined Functional Interface for Filtering an ArrayList10.5 Using the Predicate T Functional Interface for Filtering an ArrayList10.6 Accessing Members in an Enclosing Object10.7 Accessing Local Variables in an Enclosing Method10.8 Filtering an ArrayList11.1CreatingObjectsChapter11 Temporal46111.2 Using Temporal Objects11.3 Temporal Arithmetic11.4 Period-Based Loop11.5 More Temporal Arithmetic11.6 Using Default Date and Time Formatters11.7 Using Predefined Format Styles with Time-Based Values11.8 Using Predefined Format Styles with Date-Based Values11.9 Using Predefined Format Styles with Date and Time-Based Values11.10 Formatting and Parsing with Letter Patterns11.11 Formatting with Date/Time Letter 449452467472475481482488492493494497499
This page intentionally left blank
ForewordJava is now over twenty years old and the current release, JDK 8, despite its name,is really the eleventh significant release of the platform. Whilst staying true to theoriginal ideas of the platform, there have been numerous developments adding avariety of features to the language syntax as well as a huge number of APIs to thecore class libraries. This has enabled developers to become substantially more productive and has helped to eliminate a variety of common situations that can easilyresult in bugs.Java has continued to grow in popularity, which is in large part attributable to thecontinued evolution of the platform, which keeps it fresh and addresses things thatdevelopers want. According to some sources, there are more than nine million Javaprogrammers across the globe and this number looks set to continue to grow asmost universities use Java as a primary teaching language.With so many Java programmers available to employers, how do they ensure thatcandidates have the necessary skills to develop high-quality, reliable code? Theanswer is certification: a standardized test of a developer’s knowledge about thewide variety of features and techniques required to use Java efficiently and effectively. Originally introduced by Sun Microsystems, the certification process andexam has been updated to match the features of each release of Java. Oracle hascontinued this since acquiring Sun in 2010.Taking and passing the exams is not a simple task. To ensure that developers meeta high standard of knowledge about Java, the candidate must demonstrate theability to understand a wide variety of programming techniques, a clear grasp ofthe Java syntax, and a comprehensive knowledge of the standard class libraryAPIs. With the release of JDK 8, not only do Java developers need to understandthe details of imperative and object-oriented programming, they now need to havea grasp of functional programming so they can effectively use the key new features: lambda expressions and the Streams API.Which is why, ultimately, you need this book to help you prepare for the exam. Theauthors have done a great job of presenting the material you need to know to passxxvii
xxviiiFOREWORDthe exam in an approachable and easy-to-grasp way. The book starts with the fundamental concepts and language syntax and works its way through what you needto know about object-oriented programming before addressing more complex topicslike generic types. The latter part of the book addresses the most recent changes inJDK 8, that of lambda expressions, the Streams API, and the new Date and Time API.Having worked with Java almost since it was first released, both at Sun Microsystemsand then at Oracle Corporation, I think you will find this book an invaluable guideto help you pass the Oracle Certified Associate Exam for Java SE 8. I wish you thebest of luck!—Simon RitterDeputy CTO, Azul Systems
PrefaceWriting This BookDear Reader, what you hold in your hand is the result of a meticulous high-techoperation that took many months and required inspecting many parts, removingcertain parts, retrofitting some old parts, and adding many new parts to our previous book on an earlier Java programmer certification exam, until we were completely satisfied with the result. After you have read the book and passed the exam,we hope that you will appreciate the TLC (tender loving care) that has gone intothis operation. This is how it all came about.Learning the names of Java certifications and the required exams is the first itemon the agenda. This book provides coverage for the exam to earn Oracle CertifiedAssociate (OCA), Java SE 8 Programmer Certification (also know as OCAJP8). Theexam required for this certification has the name Java SE 8 Programmer I Exam(Exam number 1Z0-808). It is the first of two exams required to obtain Oracle Certified Professional (OCP), Java SE 8 Programmer Certification (also known as OCPJP8).The second exam required for this professional certification has the name Java SE 8Programmer II Exam (Exam number 1Z0-809). To reiterate, this book covers onlythe topics for the Java SE 8 Programmer I Exam that is required to obtain OCAJP8certification.A book on the new Java SE 8 certification was a long time coming. The mantle ofJava had been passed on to Oracle and Java 7 had hit the newsstand. We startedout to write a book to cover the topics for the two exams required to earn the OracleCertified Professional, Java SE 7 Programmer Certification. Soon after the release ofJava 8, Oracle announced the certification for Java SE 8. We decided to switch tothe new version. It was not a difficult decision to make. Java 8 marks a watershedwhen the language went from being a pure object-oriented language to one that alsoincorporates features of functional-style programming. As the saying goes, Java 8changed the whole ballgame. Java passed its twentieth birthday in 2015. Java 8,released a year earlier, represented a significant milestone in its history. There waslittle reason to dwell on earlier versions.xxix
PREFACExxxThe next decision concerned whether it would be best to provide coverage for the twoJava SE 8 Programmer Certification exams in one or two books. Pragmatic reasons dictated two books. It would take far too long to complete a book that covered bothexams, mainly because the second exam was largely revamped and would require alot of new material. We decided to complete the book for the first exam. Once that decision was made, our draft manuscript went back on the operating table.Our approach to writing this book has not changed from the one we employed forour previous books, mainly because it has proved successful. No stones were leftunturned to create this book, as we explain here.The most noticeable changes in the exam for OCAJP8 are the inclusion of the coreclasses in the new Date and Time API and the writing of predicates using lambdaexpressions. The emphasis remains on analyzing code scenarios, rather than individual language constructs. The exam continues to require actual experience withthe language, not just mere recitation of facts. We still claim that proficiency in thelanguage is the key to success.Since the exam emphasizes the core features of Java, this book provides in-depthcoverage of topics related to those features. As in our earlier books, supplementarytopics are also included to aid in mastering the exam topics.This book is no different from our previous books in one other important aspect: Itis a one-stop guide, providing a mixture of theory and practice that enables readersto prepare for the exam. It can be used to learn Java and to prepare for the exam.After the exam is passed, it can also come in handy as a language guide.Apa
Invoking Methods 7 1.5 Static Members 7 1.6 Inheritance 10 1.7 Associations: Aggregation and Composition 12 1.8 Tenets of Java 13 Review Questions 13 1.9 Java Programs 15 1.10 Sample Java Application 16 Essential Elements of a Java Application 16 Compiling and Running an