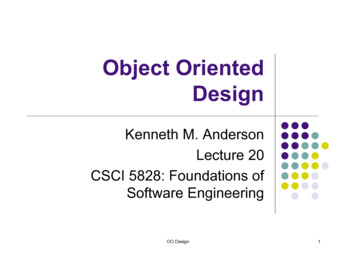
Transcription
Object OrientedDesignKenneth M. AndersonLecture 20CSCI 5828: Foundations ofSoftware EngineeringOO Design1
Object-Oriented Design Traditional procedural systems separate data andprocedures, and model these separatelyObject orientation combines data and methodstogether into a cohesive whole data abstractionThe purpose of Object-Oriented (OO) design is todefine the classes (and their relationships) that areneeded to build a system that meets therequirements contained in the SRSOO Design2
OO A&D OO techniques can be used in analysis(requirements) as well as design The methods and notations are similarIn OO analysis we model the problem domain, whilein OO design we model the solution domainOften structures created during OO analysis aresubsumed (reused, extended) in the structuresproduced by OO design The line between OO analysis and OO design is blurry, asanalysis structures will transition into model elements of thetarget systemOO Design3
Relationship of OO A&DOO Design4
OO Concepts Encapsulation grouping of related ideas into one unit which wecan refer to by a single nameFor example, methods, classes, packagesProvides information hiding by restricting theexternal visibility of a unit’s informationIn OO A&D, the object is the unit ofencapsulation An object’s data is hidden behind the publicinterface (methods) of the objectOO Design5
OO Concepts State Retention Identity – each object can be identified and treatedas a distinct entity the functions of function-oriented design do not retain state;an object, on the other hand, is aware of its past andmaintains state across method invocationsvery important issue, see lecture 10Behavior – state and methods together define thebehavior of an object, or how an object responds tothe messages passed to itOO Design6
OO Concepts. Classes – a class is a stencil from whichobjects are created; defines the structure andservices of a “class” of objects. A class has An interface which defines which parts of anobject can be accessed from outsideA body that implements the operationsInstance variables to hold object stateObjects and classes are different; a class is atype, an object is an instance State and identity is associated with objectsOO Design7
OO Concepts – access Operations in a class can be Public: accessible from outsidePrivate: accessible only from within the classProtected: accessible from within the class andfrom within subclassesOO Design8
Inheritance Inheritance is unique to OO and not availablein function-oriented languages/modelsIf class B inherits information from class A, itimplicitly acquires the attributes and methodsof A Attributes and methods of A are reused by BWhen B inherits from A, B is the subclass orderived class and A is the base class orsuperclassOO Design9
Inheritance. A subclass B generally has a derived part(inherited from A) as well as new attributes(new instance variables or methods) B’s specification only defines the new attributesThis creates an “is-a” relationship objects of type B are also objects of type AOO Design10
Inheritance OO Design11
Inheritance The inheritance relationship between classesforms a class hierarchy In models, hierarchy should represent the naturalrelationships present in the problem domainIn a hierarchy, all the common features of a set ofobjects can be accumulated in a superclassThis relationship is also known as ageneralization-specialization relationship since subclasses specialize (or extend) the moregeneric information contained in the superclassOO Design12
OO Design13
Inheritance There are several types of inheritance Strict inheritance: a subclass uses all of the features of itsparent class without modification The subclass only adds new attributes or methodsNon-strict inheritance: a subclass may redefine features ofthe superclass or ignore features of the superclassStrict inheritance supports “is-a” cleanly and hasfewer side effects If a subclass redefines a method of the parent, it canpotentially break the contract that the superclass offers itsusersOO Design14
Inheritance Single inheritance – a subclass inherits fromonly one superclass Class hierarchy is a treeMultiple inheritance – a class inherits frommore than one class Can cause runtime conflictsRepeated inheritance - a class inherits from aclass but from two separate pathsOO Design15
Inheritance and Polymorphism Inheritance enables polymorphism, i.e. anobject can be of different types An object of type B is also an object of type AHence an object has a static type and adynamic type Implications on type checkingAlso brings dynamic binding of operations whichallows writing of general code where operationsdo different things depending on the typeOO Design16
Module Level Concepts Basic modules are classesDuring OO design, a key activity is to specify theclasses in the system being builtIn creating our design, we want it to be “correct” (i.e.cover its requirements) But a design should also be “good” – efficient, modifiable,stable, We can evaluate an OO design using threeconcepts coupling, cohesion, and open-closed principleOO Design17
Coupling In OO design, three types of coupling exists interactioncomponentinheritanceOO Design18
Coupling Interaction coupling occurs when themethods of a class invoke methods ofanother class this can’t be avoided, obviously but we want to ensure that an object’s publicinterface is used a method of class A should NOT directly manipulatethe attributes of another class BWhy?OO Design19
Coupling Component coupling – when a class A hasvariables of another class C A has instance variables of type CA has a method with a parameter of type CA has a method with a local variable of type CWhen A is coupled with C, it is coupled withall subclasses of C as well Component coupling will generally imply thepresence of interaction coupling alsoOO Design20
Coupling Inheritance coupling – two classes arecoupled if one is a subclass of the other again, can’t be avoided, inheritance is a usefuland desirable feature of OO approacheshowever, a subclass should strive to only addfeatures (attributes, methods) to its superclass as opposed to modifying the features it inherits fromits superclassOO Design21
Cohesion Cohesion is an intramodule conceptFocuses on why elements are together Only elements tightly related should exist together in amodule (class)This gives a module a clear abstraction and makes it easierto understandHigher cohesion leads to lower coupling as manyotherwise interacting elements are alreadycontained in the moduleGoal is to have high cohesion in modulesThree types of cohesion in OO design method, class, and inheritanceOO Design22
Cohesion Method cohesion A class should attempt to have highly cohesivemethods, in which all of the elements within amethod body help to implement a clearly specifiedfunctionClass cohesion A class itself should be cohesive with each of itsmethods (and attributes) contributing toimplement the class’s clearly specified roleOO Design23
Cohesion Inheritance cohesion – focuses on whyclasses are together in a hierarchy Two reasons for subclassing generalization-specialization and reuseThe former occurs when the classes in the hierarchyare modeling true semantic (“is-a”) relationships foundin the problem domainThe latter sometimes occurs when a pre-existing classdoes most of what you need but for a different part ofthe semantic space; the subclass may not participatein an “is-a” relationship; this should be avoided!OO Design24
Open-closed Principle Principle: Classes should be open forextension but closed for modification Behavior can be extended to accommodate newrequirements, but existing code is not modified allows addition of code, but not modification of existingcodeMinimizes risk of having existing functionality stopworking due to changes – a very importantconsideration while changing codeOO Design25
Open-closed Principle In OO design, this principle is satisfied byusing inheritance and polymorphism Inheritance allows creating a new class to extendbehavior without changing the original classThis can be used to support the open-closedprincipleConsider example of a client object whichinteracts with a printer object for printingOO Design26
ExampleOO Design27
Example. Client directly calls methods on Printer1If another printer is required A new class Printer2 will be createdBut the client will have to be modified if it wants to use thisnew classAlternative approach Have Printer1 be a subclass of an abstract base classcalled PrinterClient is coded to access a variable of type Printer, which isinstantiated to be an instance of the Printer1 classWhen Printer2 comes along, it is made a subclass ofPrinter as well, and the client can use it withoutmodificationOO Design28
Example OO Design29
Liskov’s Substitution Principle Principle: A program using an object o1 ofbase class C should remain unchanged if o1is replaced by an object of a subclass of C The open-closed principle allows the creation ofhierarchies that intrinsically support this principleOO Design30
Unified Modeling Language(UML) and Modeling UML is a graphical design notation useful forOO analysis and design Provides nine types of diagrams to model bothstatic and dynamic aspects of a software systemUML is used by various OO designmethodologies to capture decisions about thestructure of a system under designOO Design31
Modeling Modeling is used in many disciplinesA model is a simplification of reality A good model includes those elements thathave broad effect and omits minor elements “All models are wrong, some are useful”A model of a system is not the system!We’ve covered models at the beginning ofthe semester in the concurrency textbookOO Design32
Modeling UML is used to create models of OO systemsIt contains notations to model both structuraland behavioral aspects of these systems Structure-related notations class, object, package, use case, component, anddeployment diagramsBehavior-related notations structure, collaboration, state, and activity diagramsOO Design33
Class Diagrams The class diagram is a central piece of thedesign specification of an OO design. Itspecifies the classes in a systemthe associations between classes including aggregation and composition relationshipsthe inheritance hierarchyWe covered class diagrams back in lecture10OO Design34
Interaction Diagrams Class diagrams represent static structures Interaction diagrams are used to provide insight intoa system’s dynamic behavior They do not model the behavior of a systemUseful for showing, e.g., how the objects of a use caseinteract to achieve its functionalityInteraction is between objects, not classes An object look likes a class, except its name is underlinedInteraction diagrams come in two (mostlyequivalent) styles Collaboration diagramSequence diagramOO Design35
Sequence Diagram Objects participating in an interaction are shown atthe top For each object a vertical bar represents its lifelineA message from one object to another is represented as alabeled arrowMessages can be guarded (similar to boolean guards inFSP)The ordering of messages is captured along asequence diagram’s vertical axisOO Design36
Example – sequence diag.OO Design37
Collaboration diagram Also shows how objects interactInstead of a timeline, the diagram shows theinstantiation of associations between classesat run-time The ordering of a set of messages is captured bynumbering themOO Design38
Example – collaboration diagOO Design39
Other Diagrams State diagrams (Labeled Transition Systems)Activity diagrams (from Scott Ambler’s website)OO Design40
OO Design Methodologies Many OO A&D methodologies have beenproposedBasic goal is to identify classes, understandtheir behavior, and relationships Different UML models are used for thisOO Design41
OO Design Basic steps (note: different from text book) Step 1: Analyze use casesStep 2: Create activity diagrams for each use caseStep 3: Create class diagram based on 1 and 2Step 4: Create interaction diagrams for activities containedin diagrams created in step 2Step 5: Create state diagrams for classes created in step 3Step 6: Iterate; each step above will reveal informationabout the other models that will need to be updated for instance, services specified on objects in a sequencediagram, have to be added to those objects’ classes in theclass diagramOO Design42
Restaurant example: Initial classesOO Design43
Note: this is not pureUML notation; seeLecture 10 forAdditional detailsOO Design44
Restaurant example: sequence diagramOO Design45
Metrics OO metrics focus on identifying thecomplexity of classes in an OO design Weighted Methods per ClassDepth of Inheritance TreeNumber of ChildrenCoupling Between ClassesResponse for a ClassLack of Cohesion in MethodsOO Design46
Weighted Methods Per Class The complexity of a class depends on thenumber of methods it has and the complexityof those methods For a class with methods M1, M2, , Mn,determine a complexity value for each method, c1,c2, , cn using any metric that estimates complexity forfunctions (estimated size, interface complexity, dataflow complexity, etc.)WMC Σci ; this metric has been shown to have areasonable correlation with fault pronenessOO Design47
Metrics Depth of Inheritance Tree DIT of class C is depth from the root classDIT is significant in predicting fault proneness basic idea: the deeper in the tree, the more methods aparticular class has, making it harder to changeNumber of Children Immediate number of subclasses of CGives a sense of reuse of C’s featuresMost classes have a NOC of 0; one study showed,however, that classes with a high NOC had a tendency tobe less fault prone than othersOO Design48
Metrics Coupling between classes Number of classes to which this class is coupledTwo classes are coupled if methods of one usemethods or attributes of anotherA study has shown that the CBC metric issignificant in predicting the fault proneness ofclassesOO Design49
Metrics Response for a Class CBC metric does not quantify the strength of theconnections its class has with other classes (it only countsthem)The response for a class metric attempts to quantify this bycapturing the total number of methods that can be invokedfrom an object of this classThus even if a class has a CBC of “1”, its RFC value maybe much higherA study has shown that the higher a class’s RFC value is,the larger the probability that class will contain defectsOO Design50
Metrics Lack of cohesion in methods Highly cohesive classes have small LCOM values Two methods form a cohesive pair if they access commonvariables (they form a non-cohesive pair if they have nocommon variables)LCOM is the number of method pairs that are non-cohesiveminus the number of cohesive pairsA high LCOM value indicates that the class is trying to dotoo many things and its features should be partitioned intodifferent classesHowever, a study found that this metric is NOTuseful in predicting the fault proneness of a classOO Design51
Metrics Note: the study referenced in the previousslides was published in the following paper V. R. Basili, L. Briand, and W. L. Melo. Avalidation of object-oriented design metrics asquality indicators. IEEE Transactions on SoftwareEngineering, 22(10):751-761, Oct 1996.OO Design52
Summary OO design is a newer paradigm that is replacing functionoriented design techniquesOO A&D combines both data and methods into cohesive units(classes)UML is a notation that is often used to model OO systems It provides various diagrams for modeling a system’s structure,dynamic behavior, states, architecture, etc.Creating an OO design is an iterative process based onapplying the knowledge stored in a system’s use casesSeveral OO metrics exist that are useful in prediciting the faultproneness of a classOO Design53
Inheritance Inheritance is unique to OO and not available in function-oriented languages/models If class B inherits information from class A, it implicitly acquires the attributes and methods of A Attributes and methods of A are reused by B When B inherits from A, B is the subcla