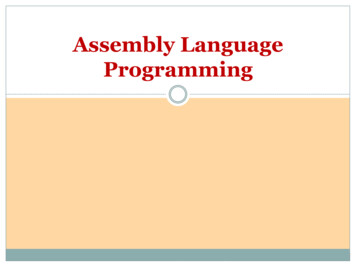
Transcription
Assembly LanguageProgramming
Integer Constants2[{ -}] digits [radix] Optional leading ‘ ’ or ‘–’ sign Binary, decimal, hexadecimal, or octal digits Common radix characters: h – hexadecimal d – decimal b – binaryIf no radix mentioned, then r – encoded realdefault is decimal q/o - octal Examples: 30d, 6Ah, 42, 1101b, 33q, 22o Hexadecimal number that beginning with letter musthave a leading zero to prevent the assemblerinterpreting it as an identifier : 0A5h
Character and String Constants3 Enclose character in single or double quotes 'A', "x" ASCII character 1 byte Enclose strings in single or double quotes "ABC" 'xyz' Each character occupies a single byte Embedded quotes: 'Say "Goodnight," Gracie'
Reserved Words4 Reserved words cannot be used as identifiers Instruction mnemonics (MOVE, ADD, MUL) Directives (INCLUDE) type attributes (BYTE, WORD) Operators ( , -) predefined symbols (@data) Directives command understood by the assembler not part of Intel instruction set case insensitive
Identifiers5 Identifiers (programmer-chosen name) May contain 1-247 characters, including digitsNot case-sensitive (by default)first character must be a letter (A.Z,a.z), , @, ? or Subsequent character may also be a digitIdentifier cannot be the same as assembler reserved word.common sense – create identifier names that easy to understand Avoid using single @ sign as first character. Ex : Valid identifierVar1, Count, MAX, first, open file, 12345, @@myfile, main
Directives6 Commands that are recognized / understood and acted upon bythe assembler Not part of the Intel instruction set Used to declare code, data areas, select memory model, declareprocedures, etc. Not case sensitive (.data, .DATA, .Data – they are the same) Different assemblers have different directives NASM ! MASM, for example Examples : .DATA – identify area of a program that contains variables .CODE – identify area of a program that contains instructions PROC – identify beginning of a procedure. name PROC
Instructions7 Assembled into machine code by assembler Executed at runtime by the CPU after the programhas been loaded into memory and started. Member of the Intel IA-32 instruction set Parts : Label (optional)Mnemonic (required)Operand (required)Comment (optional)
Example:Standard Format for Instruction8Label MnemonicsInstruction 1Instruction 2target:Operands; Commentsmoveax, 1000h; eax 1000haddebx, 4000h;ebx ebx 4000h
Labels9 Act as place marker marks the address (offset) of code and data Follow identifier rules Data label must be unique example: myArraymov ax, myArray Code label target of jump and loop instructions example: L1:target :mov ax,bx jmp target
Mnemonics and Operands10 Instruction Mnemonics is a short word that identifies theoperation carried out by an instruction (useful name). Examples : MOVmove (assign one value to another) ADDadd two values SUBsubtract one value from another MULmultiply two values INCincrement DECdecrement JMPjump to a new location CALLcall a procedure
Operands11 An assembly language can have between zero to three stc;set carry flag – no operandoperands (depending on the type of instruction)inc eax ; add 1 to eax – 1 operandTypes of operand :mov count , ebx ; move content of reg ebx into count constant (immediate value) constant expression Example: 2 4register Example: 96, 2005h, 101011010bExample: EAX, EBX, AX, AHmemory (data label) Example: count
Comments13 Comments are good. explain the program's purposewhen it was written, and by whomrevision informationtricky coding techniquesapplication-specific explanations Single-line comments begin with semicolon (;) Example:; this is a comment for add BX to AX Multi-line comments begin with COMMENT directive and a programmer-chosencharacter end with the same programmer-chosen character
Example: Multi-line Comments14COMMENT !This is a commentThis line is also a commentEverything here are comments but after exclamationcharacter it is no longer a comment!
Example: Program Template15TITLE Budget Calculator(budget.asm); Program Description:; Author:; Date Created:; Last Modification Date:INCLUDE Irvine32.inc.data(insert variables here)val1 dword 10000h::.codemain PROC(insert executable instructions here)mov ax,@data ; initialize DSexit; exit to o/smain ENDP(insert additional procedures here)END main
Example: Adding and Subtracting IntegersTITLE Add and Subtract(AddSub.asm); This program adds and subtracts 32-bit integers.INCLUDE Irvine32.inc.codemain PROCmov eax,10000hadd eax,40000hsub eax,20000hcall DumpRegsexitmain ENDPEND mainINCLUDE directive copies necessarydefinitions and setup info from text filenamed Irvine32.inc; EAX 10000h; EAX 50000h; EAX 30000hCalls a procedure that displays thecurrent values of the CPU registers.
Example: Adding and Subtracting IntegersMOV - Instruction move from source to dest. op.EAX – destination operand10000h – source operandEAX: 0ABC 0000hmov eax,10000h ; EAX 10000hEAX: 0001 0000hadd eax,40000h ; EAX 10000 40000 50000hEAX: 0005 0000hsub eax,20000h ; EAX 50000 – 20000 30000hEAX: 0003 0000h
Example Output19 Program output, showing registers and flags:EAX 00030000EBX 7FFDF000ECX 00000101EDX FFFFFFFFESI 00000000EDI 00000000EBP 0012FFF0ESP 0012FFC4EIP 00401024EFL 00000206CF 0SF 0ZF 0OF 0
Registers132dwordEAXAXwordAHbyteAL
Writing and executing a program 1. writeprogram inTextpad
Writing and executing a program 2. Save andbuild 32
Writing and executing a program 2. Save andbuild 32
Writing and executing a program 3. RunMASM
Writing and executing a program 4. View results
Activity 1: Lets Try271) Set EAX with the value of 123400h2) Add the content of EAX with 567800h3) Subtract content of EAX with 77700h4) Store content of EAX to a variable named TOTAL(declare as DWORD)
TOTAL dword ?Try changing to TOTAL word ?And see whathappens.
TITLE Class Exercise 1(CLASSEXE.asm); This program adds and subtracts 32-bit integers.INCLUDE Irvine32.inc.dataTOTAL dword 0.codemain PROCmov eax,123400hadd eax,567800hsub eax,77700hmov total, eaxmov ebx , totalcall DumpRegsexitmain ENDPEND main
Intrinsic Data Types30 BYTE, SBYTE (signed-byte) 8-bit unsigned integer; 8-bit signed integer WORD, SWORD 16-bit unsigned & signed integer DWORD, SDWORD 32-bit unsigned & signed integer QWORD (quadword) 64-bit integer TBYTE (tenbyte) 80-bit integer
Data Definition Statement31 A data definition statement sets aside storage inmemory for a variable. May optionally assign a name (label) to the data Syntax:[name] directive initializer [,initializer] . . .Nilai1BYTE34Nilai2BYTE‘A’Nilai3BYTE27h, 4Ch, 6Ah All initializers become binary data in memoryexample : 00110010b, 32h, 50d
Defining BYTE and SBYTE Data (8 bits)32 Each of the following defines a single byte of storage:
Example: Sequences of Bytes33
Example: Defining Bytes34list1BYTE 10,20,30,40list2BYTE 10,20,30,40BYTE 50,60,70,80BYTE 81,82,83,84list3BYTE ?,32,41h,00100010blist4BYTE 0Ah,20h,‘A’,22h
Defining Strings35 A string is implemented as an array of characters For convenience, it is usually enclosed in quotation marksIt usually has a null byte at the end Examples:
Defining Strings36 End-of-line character sequence:0Dh carriage return 0Ah line feed Example:
Using the DUP (duplicate) Operator37 Use DUP to allocate (create space for) an array or string. Counter and argument must be constants or constantexpressions.
Defining WORD and SWORD Data (2 bytes)38 Define storage for 16-bit integers or double characters single value or multiple values
BYTE vs WORD39
WORD40
Defining DWORD and SDWORD Data (4 bytes)41 Storage definitions for signed and unsigned 32-bitintegers:
Defining QWORD (8 bytes) ,TBYTE (10 bytes) , Real Data42 Storage definitions for quadwords, tenbyte values,and real numbers:
Little Endian Order43 All data types larger than a byte store their individualbytes in reverse order. The least significant byte (LSB) occurs at the first(lowest) memory address.
Example 144MyData DWORD 3740625, 2 DUP (2ABCCDEh)
Example 245MyData2WORD‘B’, 25, 3 DUP (4FAh)
Adding Variables to AddSub46
Equal-Sign Directive47 name expression expression is a 32-bit integer (expression or constant) may be redefined name is called a symbolic constant good programming style to use symbols
EQU Directive48
NASM ! MASM, for example . Example: Program Template 15 TITLE Budget Calculator (budget.asm) ; Program Description: ; Author: ; Date Created: ; Last Modification Date: INCLUDE Irvine32.inc .data (insert variables here) val1 dword 10000h : : .code main PROC (insert executable instructions here) mov ax,@data ; initialize DS exit; exit to o/s main ENDP (insert additional procedures here) END .