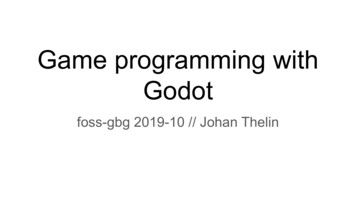
Transcription
Game programming withGodotfoss-gbg 2019-10 // Johan Thelin
What we will look at today Intro to the editor2D and 3D gamesIntro to VR using GodotIntro to deployment using Godot
Intro I encourage you to code along!All examples can be found at https://github.com/e8johan/godot-tutorial . Assets01 flippable02 platformer02b platformer animated03 on a roll04 on a roll vrthe raw assets2D intro example2D platformer2D platformer with animated hero3D example3D VR example
BioJohan Thelin - co-founder of Kuro StudioAutoliv XDIN Bitsim Trolltech Pelagicore KuroI’ve done lots and lots of embedded devices with Qt, Linux, etcAbsolutely zero game programming experience :-)
What is GodotModern game engineVisual editorOpen sourcewww.godotengine.org
Getting Godot A 25MB download from https://godotengine.org/downloadAvailable for Linux, Windows, MacOS and Server The download contains the executable - just download and run or use your distro’s package manager Today, we will focus on the standard version - no C# or other external depsI’m using version 3.1
The Editor Main view File system 2D3DScriptres://SceneInspector
Let’s create! You will learn about NodesScenesScriptsCreate nodes Node2D Sprite x2 Area2D CollisionShape2D
The flipper scriptextends Node2Donready var front Frontonready var back Backfunc on Area2D input event(viewport, event, shape idx):if event is InputEventMouseButton:if event.is pressed() and event.button index BUTTON LEFT:front.visible !front.visibleback.visible !front.visible
GDScript Very pythonesque - but not PythonCan do typing - will improve performance Resources I go to for help var x : int 10var y : calculate something()func double it(value : int) - int:Enums are ting started/scripting/gdscript/gdscript delines/godot-gdscript/There is more - my code is far from stylistically correct or efficient
Creating a world Create a world with two cards, and a UI Update UI based on state
Signal and setter for the Cardextends Node2Dsignal flipped changedvar flipped : bool false setget set flippedonready var front Frontonready var back Backfunc on Area2D input event(viewport, event, shape idx):if event is InputEventMouseButton:if event.is pressed() and event.button index BUTTON LEFT:set flipped(front.visible)func set flipped(f : bool) - void:front.visible !fback.visible fflipped femit signal("flipped changed")
The World scriptextends Node2Dfunc ready(): Label.text str(no of flipped cards()) Card.connect("flipped changed", self, " on flipped changed") Card2.connect("flipped changed", self, " on flipped changed")func on flipped changed(): Label.text str(no of flipped cards())func no of flipped cards() - int:var res : int 0if Card.flipped:res 1if Card2.flipped:res 1return res
Finding children - the smarter wayfunc find all cards() - Array:var res : Array []var children : self.get children()for child in children:if child.is in group("cards"):res.append(child)return resFrom /table.gd#L210
Platformer time! The game plan: Create tile setCreate tile mapCreate characterJump aroundYou will learn about Tiled 2D worlds2D movementAnimationsInput events
The tile set 2D Scene - “Tiles” ExtentScene Convert to TileSet.
The tile map 2D Scene - WorldTileMap Cell sizeSprite - for bg
Enter: the hero! KinematicBody2D CollisionShape2D CapsuleShapeSpriteCaptainCode!
Importing
Enter into the World
Jump around!extends KinematicBody2Dvar velocity Vector2.ZEROfunc physics process(delta):get input()velocity.y gravity * deltavelocity move and slide(velocity, Vector2.UP)if Input.is action just pressed("jump"):if is on floor():velocity.y jump speedfunc get input():velocity.x 0if Input.is action pressed("ui right"):velocity.x speedif Input.is action pressed("ui left"):velocity.x - speedhttp://kidscancode.org/godot recipes/2d/platform character/export (int) var speed 240export (int) var jump speed -320export (int) var gravity 600
What is an input?
Animations! Change Captain Code from Sprite to AnimatedSpriteNew SpriteFrames in FramesAdd the following: IdleRunJump
Play the right animationfunc get input() - int:var res : int 0velocity.x 0if Input.is action pressed("ui right"):velocity.x speedres 1if Input.is action pressed("ui left"):velocity.x - speedres -1return resfunc physics process(delta):var dir : get input()if dir 0: Sprite.flip h false Sprite.play("walk")elif dir 0: Sprite.play("walk") Sprite.flip h trueelse: Sprite.play("idle") if is on floor():.else: Sprite.play("jump")
What about 3D? You will learn about: GridMaps and Mesh librariesBasic lights and shadows3D collisions
Meshes and Maps MeshInstance Mesh: CubeMeshCreate Convex Static BodyMaterialSpatialMaterialAlbedo
A World New 3D Scene - World GridMapMesh Library meshes.tresDon’t forget the Camera!
A movable sphere KinematicBody - Player MeshInstanceCollisionShape Setup inputs Add to world
Movement scriptextends KinematicBodyvar velocity : Vector3 Vector3.ZEROconst SPEED 10const GRAVITY 10func physics process(delta):velocity.y - GRAVITY * deltaif Input.is action pressed("forward"):velocity.z -SPEEDelif Input.is action pressed("back"):velocity.z SPEEDelse:velocity.z 0if Input.is action pressed("left"):velocity.x -SPEEDelif Input.is action pressed("right"):velocity.x SPEEDelse:velocity.x 0velocity move and slide(velocity, Vector3.UP)
On a roll!
Various Bodies StaticBody KinematicBody Static, does not movePerfect for walls and suchCan be moved aboutCommonly used for charactersRigidBody Simulates newtonian physics
Let’s add some light DirectionalLightEnable shadows
Let’s make the ball jump Add input “jump”Add to physics process:if is on floor():if Input.is action just pressed("jump"):velocity.y JUMP
Going VR Godot comes with AR and VR supportI’ve played around with SteamVR (Oculus DK2) and Google Cardboard Supports cameras and wands
Setting up VR Replace Camera node with ARVROrigin and ARVRCamera Initialize sub-system:func ready():var interface ARVRServer.find interface("Native mobile")if interface and interface.initialize():get viewport().arvr true Preferably do some error handling :-)
Try it!
Deploying Godot projects Editor Project ExportRequires some setup Manage Export TemplatesAndroidiOSWindows UniversalAnd you can build your own!
Deploying Godot projects
Android development
Things we didn’t learn SoundDynamic instantiation3D assets from BlenderSkeleton animationsShadersPath findingC# bindingsGDNative, i.e. rust, C , and beyondSetting up an Android targetSetting up an iOS targetAnd much, much more.Thank you!
Game programming with Godot foss-gbg 2019-10 // Johan Thelin. What we will look at today Intro to the editor 2D and 3D games . The game plan: Create tile set Create tile map Create character Jum